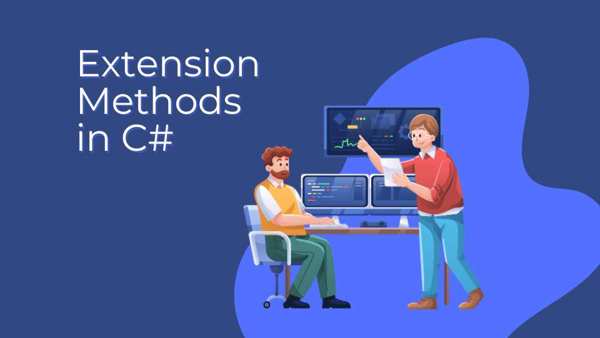
Extension methods in C# are a way to "add" new methods to existing types (classes, interfaces, or structs) without modifying their original code or creating a new derived type. Think of it like adding a new tool to a toolbox without having to buy a new toolbox. You can extend the functionality of existing types, even if you don’t have access to their source code.
Real-Life Example:
Imagine you have a string, and you want to check if it’s a valid email address. Instead of writing a separate utility function, you can create an extension method for the string
type, so you can call it like this: myString.IsValidEmail()
. This makes your code more readable and intuitive.
Advanced Code Example
Here’s how you can create and use an extension method in C#:
using System; // Define a static class to hold the extension method public static class StringExtensions { // Define the extension method for the 'string' type public static bool IsValidEmail(this string input) { // Simple email validation logic return input.Contains("@") && input.Contains("."); } } class Program { static void Main(string[] args) { string email = "test@example.com"; // Use the extension method bool isValid = email.IsValidEmail(); Console.WriteLine($"Is '{email}' a valid email? {isValid}"); // Output: Is 'test@example.com' a valid email? True } }
How it works:
- The
StringExtensions
class is defined asstatic
and contains theIsValidEmail
method. - The
this
keyword before thestring input
parameter indicates that this is an extension method for thestring
type. - You can now call
IsValidEmail()
on any string object as if it were a built-in method.
Best Practices and Features of Extension Methods
- Static Class and Method: Extension methods must be defined in a static class and must be static methods.
- Use
this
Keyword: The first parameter of the method must use thethis
keyword to specify the type being extended. - Readability: Use extension methods to make code more readable and fluent (e.g.,
list.Filter(...)
instead ofUtility.Filter(list, ...)
). - Avoid Overuse: Don’t overuse extension methods, as they can clutter the API and make the code harder to understand.
- Namespace Awareness: Extension methods are only available if the namespace containing them is imported with a
using
directive. - IntelliSense Support: Extension methods appear in IntelliSense, making them easy to discover and use.
Pros and Cons of Extension Methods
Pros:
- Extend Existing Types: Add functionality to existing types without modifying their source code.
- Improved Readability: Make code more fluent and intuitive (e.g.,
myList.Sort()
instead ofUtility.Sort(myList)
). - No Inheritance Required: Unlike inheritance, extension methods don’t require creating a derived class.
- Works with Sealed Classes: You can extend sealed classes (classes that cannot be inherited).
Cons:
- Limited Access: Extension methods cannot access private or protected members of the type they extend.
- Overuse Can Cause Confusion: Too many extension methods can make the API harder to understand.
- Namespace Dependency: Extension methods are only available if the correct namespace is imported.
Alternatives to Extension Methods
- Instance Methods: Modify the original class to add the method (if you have access to the source code).
- Utility Classes: Use static utility classes with regular static methods (e.g.,
StringUtility.IsValidEmail(email)
). - Inheritance: Create a derived class and add the method there (not applicable for sealed classes).
When to Use Extension Methods
Use extension methods when:
- You want to add functionality to a type you don’t have control over (e.g., built-in types like
string
orint
). - You want to make your code more readable and fluent.
- You need to extend sealed classes or interfaces.
- You want to provide utility methods that feel like part of the original type.
Avoid extension methods when:
- You have access to the source code and can modify the original class.
- The method logically belongs to a different class or utility.
- Overusing them would make the API confusing.
Summary
Extension methods in C# are a powerful feature that allows you to add new methods to existing types without modifying their source code. They improve code readability and provide a way to extend functionality for built-in or third-party types. However, they should be used judiciously to avoid cluttering the API. Use extension methods when you need to extend types you don’t control or when you want to make your code more fluent and intuitive.