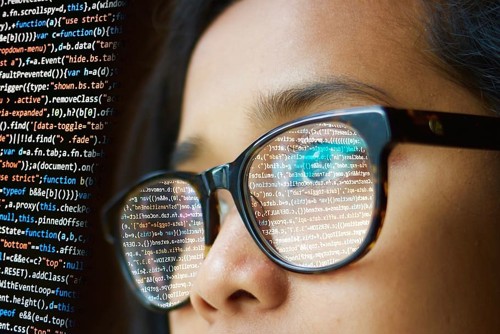
The journey to becoming a world-class programmer might seem daunting, but with the right strategy, commitment, and learning path, you can significantly improve your skills in just six months. Let’s take a step-by-step approach to transforming your programming abilities, whether you’re just starting out or looking to upskill. In this guide, we’ll walk through the stages of development, from mastering the basics to honing advanced techniques.
Month 1: Master the Fundamentals
Objective: Lay the foundation by mastering the core principles of programming.
Focus Areas:
- Choose Your Programming Language: Select a popular programming language such as Python, JavaScript, or Java. Python is often recommended for beginners due to its simplicity and versatility.
- Understand Basic Syntax: Learn how to write basic programs that include variables, loops, conditionals, and functions. These form the backbone of any programming language.
- Practice Daily: Set aside at least one hour per day to practice coding. Use platforms like Codecademy or freeCodeCamp to help structure your learning.
Example: Write a program that takes input from a user and performs simple calculations. This will help you understand how variables and user input work.
Step-by-Step Solution:
- Install an IDE: Download an Integrated Development Environment (IDE) like Visual Studio Code or PyCharm.
- Follow a Tutorial: Start with basic “Hello, World!” programs.
- Work on Small Projects: Begin coding small applications like a basic calculator or a number guessing game.
Month 2: Dive into Data Structures and Algorithms
Objective: Develop problem-solving skills by learning data structures and algorithms.
Focus Areas:
- Learn Data Structures: Understand how arrays, linked lists, stacks, queues, and hash tables work. These are crucial for optimizing your code.
- Master Sorting and Searching Algorithms: Focus on learning common algorithms such as bubble sort, quicksort, and binary search.
- Solve Algorithmic Problems: Platforms like LeetCode, HackerRank, and Codeforces offer challenges that can improve your ability to solve algorithmic problems.
Example: Create a program that sorts an array of numbers using a sorting algorithm like quicksort. This will teach you how algorithms work under the hood.
Step-by-Step Solution:
- Understand Sorting Concepts: Read about time complexity and sorting algorithms.
- Implement Sorting in Code: Write a Python function that sorts a list using quicksort.
- Test and Optimize: Test your program with different inputs and learn how to optimize it for better performance.
Month 3: Build Real-World Projects
Objective: Apply what you’ve learned by building practical, real-world projects.
Focus Areas:
- Work on Full-Stack Development: Learn both front-end and back-end development. For the front end, use HTML, CSS, and JavaScript. For the back end, focus on frameworks like Django (Python), Flask (Python), or Node.js (JavaScript).
- Create a Personal Project: Choose a project you’re passionate about, such as a to-do app, a weather app, or a blog website.
- Deploy Your Project: Use platforms like GitHub Pages or Heroku to deploy your projects online and showcase them in your portfolio.
Example: Build a blog site where users can post articles. This will teach you how databases, user authentication, and front-end design work together.
Step-by-Step Solution:
- Design the Front End: Use HTML and CSS for basic layout and styling.
- Develop the Back End: Implement the logic using a back-end framework to handle user registration, login, and post creation.
- Integrate a Database: Use SQL or NoSQL to manage data and user posts.
Month 4: Learn Version Control and Collaboration
Objective: Learn how to collaborate on projects using version control systems like Git.
Focus Areas:
- Master Git: Learn how to create repositories, commit changes, and manage branches in Git. Use GitHub to host your projects.
- Contribute to Open-Source Projects: Join open-source communities where you can contribute code and collaborate with other developers.
- Understand Agile Development: Learn the basics of agile methodologies, including sprints, user stories, and task management.
Example: Collaborate on an open-source project by fixing a bug or adding a small feature. This will teach you how to work in a team environment.
Step-by-Step Solution:
- Set Up GitHub: Create a GitHub account and initialize a repository for your projects.
- Learn Git Commands: Master essential Git commands like
git clone
,git commit
, andgit push
. - Contribute to Open Source: Find a project with the "good first issue" tag on GitHub and start contributing.
Month 5: Explore Advanced Topics
Objective: Start learning advanced programming concepts to deepen your understanding.
Focus Areas:
- Explore Object-Oriented Programming (OOP): Learn about classes, objects, inheritance, polymorphism, and encapsulation.
- Learn APIs: Understand how to work with RESTful APIs and integrate third-party services into your applications.
- Work with Databases: Deepen your knowledge of databases by learning SQL queries or working with NoSQL databases like MongoDB.
Example: Build an app that consumes a public API, such as fetching weather data from OpenWeatherMap or news from an RSS feed.
Step-by-Step Solution:
- Learn OOP Concepts: Implement classes and objects in a small project.
- Integrate an API: Write code to request data from an API and display the results.
- Handle Data: Use databases to store API data locally for further analysis or display.
Month 6: Prepare for Job Interviews and Certifications
Objective: Polish your skills and prepare for technical job interviews or certifications.
Focus Areas:
- Review Data Structures and Algorithms: Focus on the types of questions typically asked in coding interviews.
- Practice Mock Interviews: Use platforms like InterviewBit and Pramp to simulate coding interviews.
- Earn Certifications: Complete relevant certifications, such as those offered by Google, AWS, or Microsoft, to boost your resume.
Example: Prepare for a technical interview by solving algorithmic problems under timed conditions, similar to a real-world interview.
Step-by-Step Solution:
- Take Mock Interviews: Practice coding under pressure using mock interview platforms.
- Revise Key Concepts: Review the key concepts you’ve learned, including data structures, algorithms, and system design.
- Earn Certifications: Choose certifications relevant to your career goals, such as AWS Certified Developer or Google’s Python certification.
Conclusion
By following this step-by-step guide, you can become a world-class programmer in just six months. The key is to stay committed, practice consistently, and work on real-world projects that challenge and expand your skills. With dedication, you’ll be able to master the core concepts of programming, build a strong portfolio, and prepare for a rewarding career in software development.