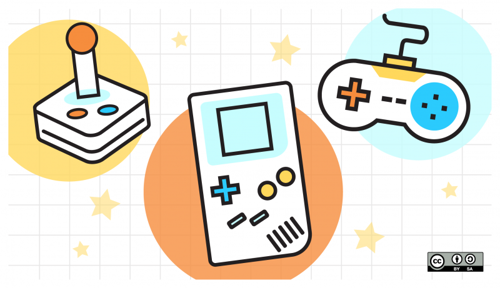
Creating games has become a lot more accessible with Python libraries like Pygame and Panda3D. Whether you want to build a simple arcade game, an interactive story, or complex simulations, these libraries can bring your ideas to life. In this blog, we’ll explore how to build games using Pygame and Panda3D, sharing real-life examples, step-by-step solutions, and the source code to help you get started.
Step 1: Getting Started with Pygame
Pygame is one of the most popular libraries for game development in Python. It provides all the necessary modules to create video games, from rendering graphics to managing user inputs and sound.
Setting Up Pygame
First, install Pygame via pip:
bashpip install pygame
Now let’s create a simple interactive game—a basic space shooter where a spaceship can move around and shoot enemies.
Step 2: Building the Game in Pygame
1. Create the Game Window
First, we need to create the game window, which will serve as the canvas for all the game elements. In Pygame, this is achieved using the pygame.display.set_mode()
function
import pygame
# Initialize Pygame
pygame.init()
# Create the game window
screen = pygame.display.set_mode((800, 600))
# Set the window title
pygame.display.set_caption("Space Shooter")
2. Load the Player and Background
Next, we load the player's spaceship and the background image.
python# Load player image and background
player_img = pygame.image.load("spaceship.png")
background = pygame.image.load("background.png")
# Set player starting position
player_x = 370
player_y = 480
player_x_change = 0
def player(x, y):
screen.blit(player_img, (x, y))
3. Handling Player Movement
We need to enable player movement using keyboard inputs (arrow keys). This is handled inside the game loop by checking the events.
python# Game loop
running = True
while running:
# Fill the screen with the background
screen.blit(background, (0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# If keystroke is pressed, check whether it's left or right
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player_x_change = -0.3
if event.key == pygame.K_RIGHT:
player_x_change = 0.3
if event.type == pygame.KEYUP:
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
player_x_change = 0
# Update the player position
player_x += player_x_change
# Keep player within boundaries
if player_x <= 0:
player_x = 0
elif player_x >= 736:
player_x = 736
player(player_x, player_y)
pygame.display.update()
4. Adding Enemy and Shooting Functionality
Next, let’s add enemies and shooting mechanics.
python# Load enemy image
enemy_img = pygame.image.load("enemy.png")
enemy_x = 50
enemy_y = 50
enemy_x_change = 0.2
# Define bullet
bullet_img = pygame.image.load("bullet.png")
bullet_x = 0
bullet_y = 480
bullet_y_change = 0.5
bullet_state = "ready" # Ready - can't see bullet, Fire - bullet is moving
# Fire bullet function
def fire_bullet(x, y):
global bullet_state
bullet_state = "fire"
screen.blit(bullet_img, (x + 16, y + 10))
# Game loop continued
while running:
# Enemy movement
enemy_x += enemy_x_change
if enemy_x <= 0:
enemy_x_change = 0.2
enemy_y += 40
elif enemy_x >= 736:
enemy_x_change = -0.2
enemy_y += 40
# Shooting
if bullet_state == "fire":
fire_bullet(bullet_x, bullet_y)
bullet_y -= bullet_y_change
player(player_x, player_y)
pygame.display.update()
With these simple mechanics, you have a basic space shooter where a player can move left and right, shoot enemies, and enemies move toward the player. You can expand the game by adding more enemies, power-ups, scoring, and different levels.
Step 3: Getting Started with Panda3D
If you're interested in 3D game development or simulations, Panda3D is an excellent choice. Panda3D is a game engine that supports 3D rendering, physics, and real-time interaction, making it ideal for more complex games.
Installing Panda3D
bashpip install panda3d
Setting up a Basic Panda3D Window
Here’s how to create a basic window using Panda3D.
pythonfrom direct.showbase.ShowBase import ShowBase
class MyGame(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# Load a 3D model
self.model = self.loader.loadModel("models/panda")
self.model.reparentTo(self.render)
self.model.setPos(0, 50, 0)
game = MyGame()
game.run()
In this code, we load a 3D model of a panda and render it in a 3D space. From here, you can expand into creating 3D simulations and interactive environments, such as building interactive stories where players explore virtual worlds or creating physics-based simulations.
Real-life Example: Interactive Story with Pygame
Let’s say you want to create an interactive story where players make choices that affect the outcome. You can use Pygame to design a game with different scenarios and decisions. For instance, imagine creating a medieval adventure where the player is a knight making decisions about quests, choosing weapons, and battling enemies.
Step-by-Step Interactive Story Example
python# Display different story options
def display_story(choice):
if choice == 1:
print("You chose to go on a quest!")
elif choice == 2:
print("You decided to stay in the castle!")
# Story choices
choice = int(input("1. Go on a quest\n2. Stay in the castle\nChoose your path: "))
display_story(choice)
From here, you can expand the game by adding combat mechanics, branching storylines, and more.
Conclusion
Whether you are interested in building simple 2D games using Pygame or more complex 3D simulations using Panda3D, Python makes game development accessible and fun. You can create interactive stories, arcade games, and even immersive simulations with relatively little code. The examples we discussed, from a basic space shooter to a 3D interactive model, illustrate the versatility of these libraries.