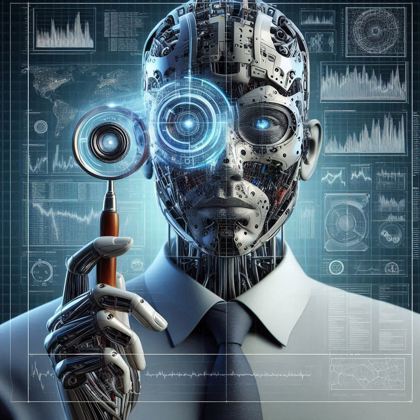
Machine learning has become one of the most powerful tools for businesses today. Whether it's forecasting sales, detecting fraud, or classifying images, machine learning allows companies to harness the power of data to make better, faster decisions. One of the most accessible machine learning libraries for Python developers is scikit-learn. In this blog, we will explore how to build predictive models using scikit-learn, covering real-life examples such as sales forecasting and fraud detection.
The Power of Predictive Modeling
Imagine a retail company that wants to forecast future sales. Instead of relying on intuition or historical trends, predictive models can use past sales data, market conditions, and other variables to create accurate forecasts. Similarly, banks and online businesses can use machine learning to detect fraud by recognizing unusual patterns in transactions.
Let’s walk through a step-by-step guide to building a predictive model using scikit-learn, focusing on sales forecasting.
Step 1: Setting up the Environment
First, we need to install the necessary libraries, including scikit-learn, pandas, and matplotlib. Here's how to set up your Python environment:
bashpip install scikit-learn pandas matplotlib
Step 2: Loading the Data
For this example, let’s use a hypothetical sales dataset that includes information such as the number of units sold, the day of the week, the advertising budget, and other related variables.
pythonimport pandas as pd
# Load sales data
data = pd.read_csv('sales_data.csv')
# Inspect the first few rows of the dataset
print(data.head())
Assume the dataset has columns such as units_sold
, day_of_week
, advertising_budget
, and discount_offered
. These variables will be used to predict the number of units sold.
Step 3: Preparing the Data
To build a predictive model, we need to split the data into features (independent variables) and the target variable (the dependent variable we are trying to predict).
python# Features (independent variables)
X = data[['day_of_week', 'advertising_budget', 'discount_offered']]
# Target (dependent variable)
y = data['units_sold']
Next, we split the data into training and testing sets. This allows us to train the model on one portion of the data and test its performance on another.
pythonfrom sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 4: Training the Model
Now that the data is prepared, we can choose a model to train. For this example, we’ll use a Linear Regression model, which is one of the simplest yet effective models for forecasting continuous values.
pythonfrom sklearn.linear_model import LinearRegression
# Initialize the model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
Step 5: Making Predictions
Once the model is trained, we can use it to make predictions on the test set and evaluate how well it performs.
python# Make predictions
y_pred = model.predict(X_test)
# Print first 10 predictions
print(y_pred[:10])
Step 6: Evaluating the Model
To assess the model’s performance, we can calculate the Mean Absolute Error (MAE), Mean Squared Error (MSE), and R-squared score.
pythonfrom sklearn.metrics import mean_absolute_error, mean_squared_error, r2_score
# Calculate evaluation metrics
mae = mean_absolute_error(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f"Mean Absolute Error: {mae}")
print(f"Mean Squared Error: {mse}")
print(f"R-squared: {r2}")
These metrics will give us insight into how accurate the model is and where it could be improved. In a real-life scenario, this predictive model could help a business make informed decisions about inventory levels, marketing spend, and resource allocation.
Real-life Example: Detecting Fraud with Predictive Modeling
In another scenario, we can use scikit-learn to build a model for fraud detection. Fraud detection involves identifying suspicious transactions that deviate from normal behavior. This time, we will use a Logistic Regression model, which is widely used for binary classification tasks.
Assume we have a dataset with features like transaction_amount
, transaction_type
, and account_balance
, where the target variable is whether the transaction is fraudulent (1
) or not (0
).
pythonfrom sklearn.linear_model import LogisticRegression
# Load the data
fraud_data = pd.read_csv('fraud_data.csv')
# Features
X = fraud_data[['transaction_amount', 'transaction_type', 'account_balance']]
# Target
y = fraud_data['is_fraud']
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize the model
fraud_model = LogisticRegression()
# Train the model
fraud_model.fit(X_train, y_train)
# Make predictions
y_pred_fraud = fraud_model.predict(X_test)
The process of training, predicting, and evaluating remains largely the same as we did with sales forecasting. This time, however, we focus on identifying fraudulent transactions and reducing financial risk.
Step-by-Step Fraud Detection Code
python# Evaluation for Fraud Detection
from sklearn.metrics import accuracy_score, precision_score, recall_score
# Accuracy of the model
accuracy = accuracy_score(y_test, y_pred_fraud)
# Precision (how many predicted frauds were actually frauds)
precision = precision_score(y_test, y_pred_fraud)
# Recall (how many frauds were correctly identified)
recall = recall_score(y_test, y_pred_fraud)
print(f"Accuracy: {accuracy}")
print(f"Precision: {precision}")
print(f"Recall: {recall}")
Conclusion
Machine learning models built using scikit-learn are powerful tools for businesses, whether it's forecasting sales, detecting fraud, or classifying images. By leveraging Python and open-source libraries, you can build effective models and make data-driven decisions that directly impact your business outcomes.
Predictive modeling with scikit-learn is an essential skill for developers and data scientists alike. The flexibility of scikit-learn allows it to be applied across a variety of industries, from retail to finance.