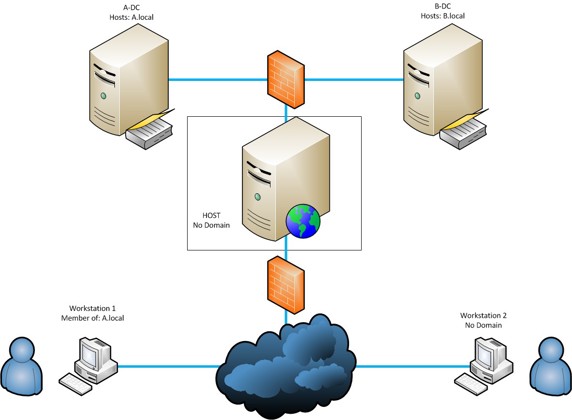
In today's world, securing applications is paramount, especially when dealing with sensitive data. Whether you’re building a small app or an enterprise-level solution, encryption, authentication, and authorization are vital for safeguarding user data and controlling access to resources. Java provides robust tools and libraries to handle these security tasks, but it’s essential to follow best practices to ensure your application remains secure.
This article explores how to implement encryption, authentication, and authorization in Java, using real-world examples and step-by-step solutions. We'll also highlight best practices that developers should follow to secure their applications effectively.
Real-Life Example: Securing an Online Banking Application
Imagine you're building an online banking application. Users need to log in securely, access their bank accounts, and make transactions. This involves encrypting sensitive information (like passwords and transaction details), verifying user identities through authentication, and ensuring only authorized users can perform certain actions, such as transferring money.
1. Encryption in Java
Encryption ensures that sensitive data, such as passwords or personal information, remains confidential by converting it into an unreadable format. In Java, the javax.crypto
package provides classes for encryption, decryption, and key generation.
Example: Encrypting and Decrypting Data
Let’s encrypt a string (e.g., a user’s password) using AES (Advanced Encryption Standard), one of the most commonly used encryption algorithms.
java import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.util.Base64; public class EncryptionExample { public static void main(String[] args) throws Exception { String data = "SensitiveData123"; SecretKey secretKey = KeyGenerator.getInstance("AES").generateKey(); // Encrypting the data Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, secretKey); byte[] encryptedData = cipher.doFinal(data.getBytes()); String encryptedString = Base64.getEncoder().encodeToString(encryptedData); System.out.println("Encrypted Data: " + encryptedString); // Decrypting the data cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedData = cipher.doFinal(Base64.getDecoder().decode(encryptedString)); System.out.println("Decrypted Data: " + new String(decryptedData)); } }
Explanation:
- KeyGenerator creates a secret key for AES encryption.
- Cipher handles encryption and decryption operations.
- We convert the encrypted bytes to Base64 for readability.
Real-World Analogy: Think of encryption like locking a valuable document in a safe. Even if someone gets hold of the document, they can't read it without the key.
2. Authentication in Java
Authentication verifies a user's identity before granting access to the application. A typical approach is to compare a provided password against a stored, hashed password.
Example: Password Hashing and Authentication
Storing passwords in plaintext is a security risk. Instead, we hash them using algorithms like PBKDF2, BCrypt, or Argon2.
Here’s how you can hash a password using PBKDF2:
java Copy code import javax.crypto.SecretKeyFactory; import javax.crypto.spec.PBEKeySpec; import java.security.SecureRandom; import java.util.Base64; public class PasswordHashingExample { public static String hashPassword(String password) throws Exception { byte[] salt = new byte[16]; SecureRandom random = new SecureRandom(); random.nextBytes(salt); PBEKeySpec spec = new PBEKeySpec(password.toCharArray(), salt, 65536, 128); SecretKeyFactory factory = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA1"); byte[] hash = factory.generateSecret(spec).getEncoded(); return Base64.getEncoder().encodeToString(salt) + ":" + Base64.getEncoder().encodeToString(hash); } public static void main(String[] args) throws Exception { String password = "SecurePassword123"; String hashedPassword = hashPassword(password); System.out.println("Hashed Password: " + hashedPassword); } }
Explanation:
- Salt: Random data added to the password before hashing to make the hash unique.
- PBEKeySpec: Defines the password and salt, along with the iteration count and key length.
Real-World Analogy: Hashing a password is like shredding a piece of paper with sensitive information. The original form (password) is no longer retrievable directly from the hash.
3. Authorization in Java
Authorization ensures that authenticated users can only access the resources they are allowed to. This is especially critical in applications where different users have different roles, like in an online banking system where a customer and an admin have different privileges.
Java provides several ways to handle authorization, including frameworks like Spring Security.
Example: Role-Based Access Control (RBAC) in Spring Security
Here’s an example of setting up role-based authorization using Spring Security:
xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> java import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.inMemoryAuthentication() .withUser("user").password("{noop}password").roles("USER") .and() .withUser("admin").password("{noop}admin").roles("ADMIN"); } @Override protected void configure(HttpSecurity http) throws Exception { http.authorizeRequests() .antMatchers("/admin/**").hasRole("ADMIN") .antMatchers("/user/**").hasRole("USER") .anyRequest().authenticated() .and() .formLogin(); } }
Explanation:
- In-memory authentication is used to define user credentials for demonstration.
HttpSecurity
is used to configure access rules: only users with theADMIN
role can access/admin
resources.
Real-World Analogy: Authorization is like security guards checking access badges. Employees with the right clearance can enter restricted areas, while others can only access general spaces.
Best Practices for Encryption, Authentication, and Authorization in Java
- Always Use Strong Encryption: Use modern algorithms like AES for encryption. Avoid weak or deprecated algorithms (e.g., DES or MD5).
- Never Store Plaintext Passwords: Always hash and salt passwords before storing them.
- Use Secure Randomness: For generating keys, salts, or tokens, always use
SecureRandom
rather thanRandom
. - Keep Secrets Secret: Securely store secret keys, certificates, or passwords using a key management system or environment variables.
- Use Role-Based Access Control (RBAC): Implement a clear role hierarchy to restrict access to certain areas of your application based on user roles.
- Implement Multi-Factor Authentication (MFA): Add an extra layer of security by requiring additional verification steps beyond passwords.
- Keep Dependencies Up-to-Date: Ensure your encryption and security libraries are updated regularly to protect against known vulnerabilities.
- Secure Data in Transit: Use SSL/TLS to encrypt data exchanged between your application and external systems.
- Use Frameworks for Authentication and Authorization: Use established frameworks like Spring Security, which provide built-in mechanisms for handling security.
- Log Authentication and Authorization Attempts: Track and analyze failed login or access attempts to identify potential security breaches.
Conclusion
Implementing encryption, authentication, and authorization in Java is essential for securing applications, especially those handling sensitive data like banking systems or personal user information. By following best practices, such as using modern encryption algorithms, securely storing passwords, and applying role-based access control, developers can safeguard their applications from threats.
Java provides powerful tools, but it’s critical to stay up-to-date with security protocols and ensure that all security measures are correctly implemented. Security is a multi-layered approach, and getting these fundamentals right is the first step toward building a secure Java application.