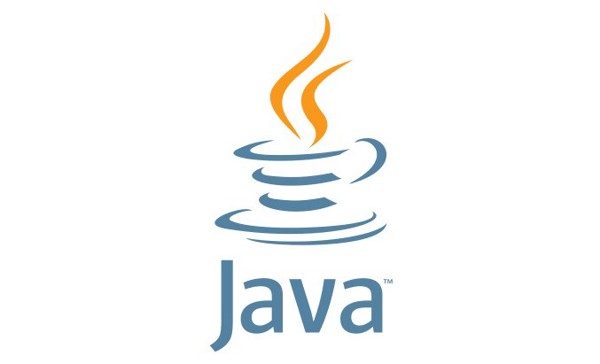
Java Database Connectivity (JDBC) is a standard Java API that allows developers to interact with relational databases. With JDBC, you can connect to a database, execute SQL queries, and perform CRUD operations (Create, Read, Update, Delete). This guide will show you how to use JDBC to manage a database effectively, with step-by-step examples of each operation.
Real-Life Example: Managing an Employee Database
Imagine you’re developing a system to manage employee information for a company. The database needs to store employee details, retrieve records when needed, update data, and remove outdated entries. JDBC provides a simple and powerful way to interact with a relational database, enabling you to perform all these operations seamlessly.
Setting Up JDBC
Before diving into CRUD operations, let’s set up the JDBC environment. You’ll need:
- Java Development Kit (JDK) installed on your machine.
- A database (e.g., MySQL, PostgreSQL) installed and running.
- JDBC Driver: Download the JDBC driver for your database and add it to your project’s classpath.
Here’s an example of how to set up a JDBC connection with a MySQL database.
Step 1: Establishing a Database Connection
java import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DatabaseConnection { private static final String URL = "jdbc:mysql://localhost:3306/employee_db"; private static final String USER = "root"; private static final String PASSWORD = "password"; public static Connection getConnection() { Connection connection = null; try { connection = DriverManager.getConnection(URL, USER, PASSWORD); System.out.println("Connection to the database established successfully."); } catch (SQLException e) { e.printStackTrace(); } return connection; } }
Explanation:
- URL specifies the location of the database.
- User and Password authenticate the connection.
DriverManager.getConnection()
establishes the connection to the database.
Real-World Analogy: Connecting to a database is like opening the door to a warehouse filled with files (data). You need the correct key (username and password) to access it.
CRUD Operations with JDBC
Once the connection is established, we can perform CRUD operations.
1. Create: Inserting New Records
Let’s add new employee records to the employee
table.
java import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.SQLException; public class InsertEmployee { public static void insertEmployee(int id, String name, String position) { String query = "INSERT INTO employee (id, name, position) VALUES (?, ?, ?)"; try (Connection connection = DatabaseConnection.getConnection(); PreparedStatement preparedStatement = connection.prepareStatement(query)) { preparedStatement.setInt(1, id); preparedStatement.setString(2, name); preparedStatement.setString(3, position); preparedStatement.executeUpdate(); System.out.println("Employee inserted successfully."); } catch (SQLException e) { e.printStackTrace(); } } public static void main(String[] args) { insertEmployee(1, "John Doe", "Software Engineer"); } }
Explanation:
- PreparedStatement: Used to prevent SQL injection and allow dynamic queries.
- We provide placeholders (
?
) for values, then set them withsetInt()
andsetString()
methods.
Real-World Analogy: This is like adding a new employee file to the system.
2. Read: Retrieving Data from the Database
Now, let’s fetch employee records from the database.
java import java.sql.Connection; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class RetrieveEmployees { public static void getEmployees() { String query = "SELECT * FROM employee"; try (Connection connection = DatabaseConnection.getConnection(); Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery(query)) { while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); String position = resultSet.getString("position"); System.out.println("ID: " + id + ", Name: " + name + ", Position: " + position); } } catch (SQLException e) { e.printStackTrace(); } } public static void main(String[] args) { getEmployees(); } }
Explanation:
- ResultSet: Holds the results from the executed SQL query.
- The
while(resultSet.next())
loop retrieves each row of the result set.
Real-World Analogy: Retrieving employee records is like searching through files in a cabinet to find the information you need.
3. Update: Modifying Existing Records
Let’s update an employee’s position.
java import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.SQLException; public class UpdateEmployee { public static void updateEmployeePosition(int id, String newPosition) { String query = "UPDATE employee SET position = ? WHERE id = ?"; try (Connection connection = DatabaseConnection.getConnection(); PreparedStatement preparedStatement = connection.prepareStatement(query)) { preparedStatement.setString(1, newPosition); preparedStatement.setInt(2, id); preparedStatement.executeUpdate(); System.out.println("Employee position updated successfully."); } catch (SQLException e) { e.printStackTrace(); } } public static void main(String[] args) { updateEmployeePosition(1, "Senior Software Engineer"); } }
Explanation:
- We use a SQL
UPDATE
statement to modify existing data.
Real-World Analogy: Updating employee data is like editing a file in a cabinet to reflect new information.
4. Delete: Removing Records from the Database
Finally, let’s delete an employee record.
java import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.SQLException; public class DeleteEmployee { public static void deleteEmployee(int id) { String query = "DELETE FROM employee WHERE id = ?"; try (Connection connection = DatabaseConnection.getConnection(); PreparedStatement preparedStatement = connection.prepareStatement(query)) { preparedStatement.setInt(1, id); preparedStatement.executeUpdate(); System.out.println("Employee deleted successfully."); } catch (SQLException e) { e.printStackTrace(); } } public static void main(String[] args) { deleteEmployee(1); } }
Explanation:
- We use a
DELETE
SQL query to remove data from the database.
Real-World Analogy: Deleting an employee record is like removing a file from the filing cabinet and discarding it.
Best Practices for Using JDBC
- Use Connection Pooling: Reuse database connections to improve performance rather than opening a new connection for each operation.
- Close Resources Properly: Always close the
Connection
,PreparedStatement
, andResultSet
after use. Use try-with-resources to do this efficiently. - Use Prepared Statements: Prevent SQL injection by using
PreparedStatement
instead of string concatenation in queries. - Handle Exceptions Gracefully: Ensure you catch and handle
SQLException
properly. Logging errors is essential for debugging. - Optimize Queries: Write efficient SQL queries and use indexing where necessary to improve database performance.
- Transaction Management: For multiple operations, use transactions to ensure data integrity. Use
commit()
androllback()
to manage transactions.
Conclusion
Java Database Connectivity (JDBC) offers a straightforward way to interact with relational databases using Java. With JDBC, you can easily perform CRUD operations and manage data in real-time applications, as demonstrated with employee data. Following best practices such as connection pooling, closing resources, and using prepared statements can improve both performance and security in your database-driven applications.