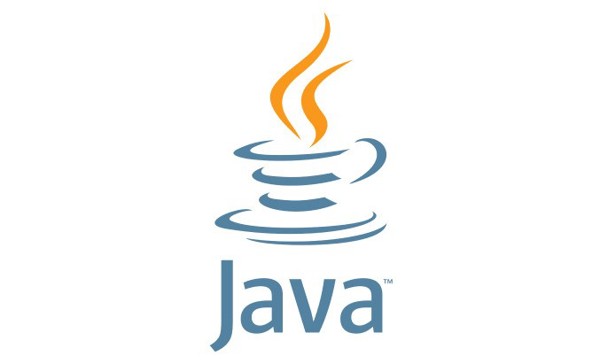
Java 8 introduced the Stream API, a powerful tool that simplifies operations on collections and other data sources, providing a functional-style way of processing data. In this article, we'll explore how to use Java Stream API effectively, with a focus on filtering, mapping, and reducing data streams, using real-life examples.
Stream API enables developers to write concise, expressive code that can easily be parallelized. We'll walk through each of these operations step-by-step and show how they relate to real-world problems. Additionally, best practices for optimizing performance and code readability will be highlighted.
Real-Life Example: A Retail Store's Data Processing
Imagine you're managing a retail store with thousands of products. You want to process product data to filter out products based on availability, map the remaining products to get their prices, and then sum up the prices for a revenue report. The Java Stream API helps achieve this in a clear and concise way.
Understanding Java Stream API Basics
A stream in Java is a sequence of elements from a source that supports aggregate operations. Streams are not data structures; they don’t store data, but instead, allow the developer to manipulate data through operations like filtering, mapping, and reducing.
Key Stream Operations:
- Filtering: Select elements that match a given condition.
- Mapping: Transform each element into another form.
- Reducing: Aggregate elements into a single result, like summing them.
Filtering: Removing Unnecessary Data
One of the common tasks when working with data is filtering out unnecessary information. For example, you might want to filter out products that are out of stock.
Here’s how to filter products with a price greater than $20:
java import java.util.List; import java.util.stream.Collectors; class Product { String name; double price; Product(String name, double price) { this.name = name; this.price = price; } public double getPrice() { return price; } @Override public String toString() { return name + " - $" + price; } } public class FilterExample { public static void main(String[] args) { List<Product> products = List.of( new Product("Laptop", 1200), new Product("Headphones", 15), new Product("Monitor", 150), new Product("Keyboard", 50) ); // Filter products priced over $20 List<Product> filteredProducts = products.stream() .filter(p -> p.getPrice() > 20) .collect(Collectors.toList()); System.out.println(filteredProducts); } }
Explanation:
- We created a list of products and used the
filter()
method to select products with a price greater than $20. - The filtered result is collected back into a list using
Collectors.toList()
.
Real-World Analogy: Imagine you're at a store, and you're only interested in buying items that cost more than $20. You’d ignore anything priced lower. That’s essentially what filter()
does here.
Mapping: Transforming Data
The map()
method is used to transform each element in the stream. In a store scenario, you might want to extract the prices of all available products.
java import java.util.List; import java.util.stream.Collectors; public class MapExample { public static void main(String[] args) { List<Product> products = List.of( new Product("Laptop", 1200), new Product("Headphones", 15), new Product("Monitor", 150), new Product("Keyboard", 50) ); // Extract the prices of the products List<Double> productPrices = products.stream() .map(Product::getPrice) .collect(Collectors.toList()); System.out.println(productPrices); } }
Explanation:
- The
map()
method takes each product from the list and transforms it into a list of prices by extracting the price attribute.
Real-World Analogy: If you're in a store with a list of products, you may only be interested in their prices, ignoring other details. The map()
function helps you get those specific details.
Reducing: Summing Up Data
Once you've filtered and mapped your data, you may want to reduce the stream to a single result, like calculating the total price of all products.
java import java.util.List; public class ReduceExample { public static void main(String[] args) { List<Product> products = List.of( new Product("Laptop", 1200), new Product("Headphones", 15), new Product("Monitor", 150), new Product("Keyboard", 50) ); // Calculate the total price of all products double totalPrice = products.stream() .map(Product::getPrice) .reduce(0.0, Double::sum); System.out.println("Total Price: $" + totalPrice); } }
Explanation:
reduce()
accumulates all product prices into a single total using thesum()
function.
Real-World Analogy: When you reach the checkout counter at a store, the cashier sums up all the prices of the products in your basket. That’s what reduce()
is doing.
Best Practices for Java Stream API
- Use Parallel Streams Wisely: Parallel streams can speed up performance when processing large data sets, but they should be used with care in non-thread-safe environments.
java products.parallelStream() // Processes in parallel
- Prefer Method References: Instead of using lambda expressions, use method references for cleaner code.
java .map(Product::getPrice)
- Avoid Modifying the Source: Streams are designed to be stateless and should not modify the underlying collection. Avoid side effects within stream operations.
- Use Collectors Efficiently: Always specify a terminal operation such as
collect()
,reduce()
, orforEach()
to complete the stream pipeline. - Chain Operations: Stream operations can be chained for more readable and concise code. For example, filtering and then mapping in one go:
java products.stream() .filter(p -> p.getPrice() > 20) .map(Product::getPrice) .collect(Collectors.toList());
Conclusion
The Java Stream API provides a powerful and flexible way to manipulate collections and other data structures through its filtering, mapping, and reducing capabilities. Using real-life scenarios, such as processing a list of products in a retail store, helps to understand the practical application of streams.
By following best practices, such as using parallel streams judiciously and leveraging method references, you can write clean, efficient, and maintainable code. Streams simplify complex data operations, making them more intuitive and scalable.