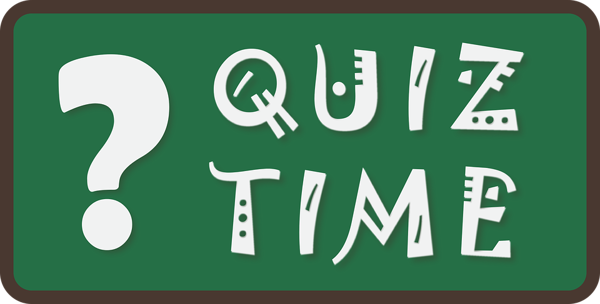
Building a dynamic quiz game with JavaScript is a great project for anyone looking to sharpen their web development skills. Whether you are learning JavaScript or building an interactive feature for your website, a quiz game is an excellent way to apply real-life coding techniques. In this guide, we'll walk you through creating a fully functional dynamic quiz game from scratch using JavaScript. We’ll also explore best practices and how the concepts apply to real-world scenarios.
Why Build a Quiz Game?
Consider this: You're organizing a training event at work and want to ensure that the attendees have understood the key takeaways. Instead of handing out printed tests or using external tools, you can create a customized, dynamic quiz tailored to the session using JavaScript. The participants can take the quiz directly from their browsers, and the results can be displayed instantly.
Let’s dive into the step-by-step process of building a dynamic quiz game with JavaScript.
Step 1: Set Up HTML Structure
First, we'll create a simple HTML page that contains the basic structure of our quiz. Here's a sample structure:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dynamic Quiz Game</title> </head> <body> <div id="quiz-container"> <h1>Quiz Game</h1> <div id="question-container"> <p id="question"></p> </div> <div id="answer-buttons" class="btn-container"> <button class="answer-btn"></button> <button class="answer-btn"></button> <button class="answer-btn"></button> <button class="answer-btn"></button> </div> <button id="next-btn" class="next-btn">Next</button> </div> <script src="quiz.js"></script> </body> </html>
Here, we set up the basic layout with a container for the quiz and buttons for the answers. There's also a "Next" button that allows the user to move to the next question.
Step 2: Write the JavaScript Logic
Now that we have the structure, we need the JavaScript to drive the functionality of the quiz.
javascript const questions = [ { question: "What is the capital of France?", answers: [ { text: "Berlin", correct: false }, { text: "Madrid", correct: false }, { text: "Paris", correct: true }, { text: "Rome", correct: false } ] }, { question: "Who wrote 'To Kill a Mockingbird'?", answers: [ { text: "Harper Lee", correct: true }, { text: "J.K. Rowling", correct: false }, { text: "Ernest Hemingway", correct: false }, { text: "Mark Twain", correct: false } ] } ]; const questionElement = document.getElementById('question'); const answerButtonsElement = document.getElementById('answer-buttons'); const nextButton = document.getElementById('next-btn'); let currentQuestionIndex = 0; let score = 0; function startGame() { currentQuestionIndex = 0; score = 0; nextButton.classList.add('hide'); showQuestion(); } function showQuestion() { resetState(); const currentQuestion = questions[currentQuestionIndex]; questionElement.innerText = currentQuestion.question; currentQuestion.answers.forEach(answer => { const button = document.createElement('button'); button.innerText = answer.text; button.classList.add('answer-btn'); if (answer.correct) { button.dataset.correct = answer.correct; } button.addEventListener('click', selectAnswer); answerButtonsElement.appendChild(button); }); } function resetState() { nextButton.classList.add('hide'); while (answerButtonsElement.firstChild) { answerButtonsElement.removeChild(answerButtonsElement.firstChild); } } function selectAnswer(e) { const selectedButton = e.target; const correct = selectedButton.dataset.correct === 'true'; if (correct) { score++; } Array.from(answerButtonsElement.children).forEach(button => { setStatusClass(button, button.dataset.correct === 'true'); }); if (questions.length > currentQuestionIndex + 1) { nextButton.classList.remove('hide'); } else { nextButton.innerText = 'Restart'; nextButton.classList.remove('hide'); } } function setStatusClass(element, correct) { clearStatusClass(element); if (correct) { element.classList.add('correct'); } else { element.classList.add('wrong'); } } function clearStatusClass(element) { element.classList.remove('correct'); element.classList.remove('wrong'); } nextButton.addEventListener('click', () => { currentQuestionIndex++; showQuestion(); }); startGame();
Step 3: CSS for Styling
To make the quiz look polished, we need some basic CSS styling.
css body { font-family: Arial, sans-serif; } #quiz-container { max-width: 600px; margin: 50px auto; text-align: center; } .btn-container { display: flex; flex-direction: column; } .answer-btn { margin: 10px; padding: 10px; background-color: #f0f0f0; border: none; cursor: pointer; } .answer-btn.correct { background-color: #28a745; } .answer-btn.wrong { background-color: #dc3545; } .next-btn { margin-top: 20px; padding: 10px 20px; background-color: #007bff; color: white; border: none; cursor: pointer; display: none; } .hide { display: none; }
Step 4: Handling Quiz Results and Feedback
After all the questions are answered, you can calculate the score and give feedback.
Add this to the JavaScript:
javascript function showResults() { questionElement.innerText = `Quiz complete! You scored ${score} out of ${questions.length}.`; nextButton.innerText = 'Restart'; nextButton.classList.remove('hide'); }
In the real world, you could use this feedback in an educational setting where a teacher uses it to assess students. It could also be used in job applications or training modules for evaluating the candidate’s knowledge.
Best Practices
- Separation of Concerns: Keep your HTML, CSS, and JavaScript separate for better maintainability.
- User-Friendly Feedback: Provide immediate feedback for correct or incorrect answers using CSS class changes.
- Dynamic Question Generation: The use of a question array keeps the quiz dynamic. You can easily add or remove questions without altering the main logic.
- Handling User Input: Always validate and handle user input carefully, especially in real-world applications like educational assessments.
- Optimized for Mobile: Ensure your design is responsive so users can take the quiz on any device.
Step-by-Step Features
- Question Navigation: The user can move to the next question after selecting an answer.
- Score Tracking: Each correct answer increments the score.
- Immediate Feedback: Buttons change colors based on the correctness of the answer.
- Restart Option: After the quiz is completed, users can restart it.