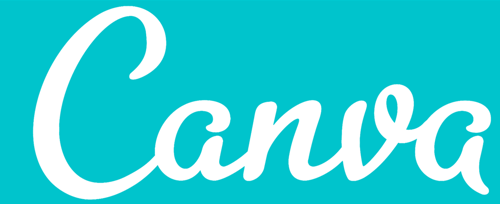
In the world of web development, Sarah—a creative web developer—found herself facing a challenge. She wanted to create an interactive design for her client that involved drawing shapes, text, and animations. Her client also requested that the design be responsive across different devices and browsers. After doing some research, she discovered two powerful tools that could help her achieve her goals: Canvas and SVG Graphics.
But Sarah didn’t know where to begin. Should she use Canvas, or was SVG better suited for the job? This story follows Sarah’s journey as she explores the advantages, differences, and best practices of Canvas and SVG Graphics in JavaScript. Along the way, we’ll dive into step-by-step code examples, real-life scenarios, and tips for both beginners and advanced users.
Chapter 1: Understanding Canvas and SVG
Before Sarah could decide which technology to use, she needed to understand the key differences between Canvas and SVG.
Canvas: This is a pixel-based graphics system. It allows Sarah to draw images, shapes, and animations by manipulating individual pixels on a grid. Canvas is part of the HTML5 API and is ideal for creating complex, dynamic graphics and animations.
SVG (Scalable Vector Graphics): Unlike Canvas, SVG is a vector-based system. It uses XML to describe graphics in terms of shapes and paths. SVG is resolution-independent, which means it scales perfectly without losing quality, making it ideal for responsive designs.
Sarah thought about her client’s needs—simple animations and high responsiveness across devices. She decided to start by experimenting with both Canvas and SVG to see which would suit the project best.
Chapter 2: Drawing with Canvas
Canvas works with the <canvas>
HTML element, and you can draw graphics using JavaScript. Here’s how Sarah started with basic shapes:
html<canvas id="myCanvas" width="500" height="400"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Draw a rectangle
ctx.fillStyle = '#FF0000';
ctx.fillRect(50, 50, 150, 100);
// Draw a circle
ctx.beginPath();
ctx.arc(200, 150, 50, 0, Math.PI * 2, false);
ctx.fillStyle = '#0000FF';
ctx.fill();
ctx.closePath();
</script>
Sarah’s code creates a rectangle and a circle on the canvas. She used the fillRect()
method for the rectangle and arc()
to draw the circle. The getContext('2d')
is essential, as it tells Canvas to render graphics in 2D mode.
Real-Life Example: Sarah’s Interactive Quiz
Sarah imagined using Canvas for a small game within her client’s quiz app. She could use Canvas to create interactive elements like dynamic scoreboards or custom visual feedback. Its ability to control every pixel would make it perfect for complex animations and transitions.
Chapter 3: Drawing with SVG
SVG uses the <svg>
tag in HTML, and it’s great for static images and vector-based graphics that need to scale across devices.
Sarah started experimenting with basic SVG shapes:
html<svg width="500" height="400">
<!-- Draw a rectangle -->
<rect x="50" y="50" width="150" height="100" fill="red"></rect>
<!-- Draw a circle -->
<circle cx="200" cy="150" r="50" fill="blue"></circle>
</svg>
Here, the <rect>
and <circle>
elements define a rectangle and a circle, respectively. Sarah loved how clean and simple the syntax was compared to Canvas.
Real-Life Example: Sarah’s Company Logo
SVG turned out to be the perfect solution for Sarah’s client’s logo. Since the logo needed to be scalable across devices, SVG ensured that the image would retain its quality on mobile phones, tablets, and desktop browsers.
Chapter 4: Animation in Canvas vs SVG
Sarah’s next step was to add animation to her graphics. She learned that Canvas animation relies on repeatedly redrawing the entire graphic using JavaScript, while SVG uses CSS and SMIL (Synchronized Multimedia Integration Language) to animate vector-based graphics.
Here’s how Sarah added a simple animation to her Canvas drawing using JavaScript:
javascriptlet x = 0;
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = '#FF0000';
ctx.fillRect(x, 50, 150, 100);
x += 2;
if (x < canvas.width) {
requestAnimationFrame(draw);
}
}
draw();
In this example, Sarah used requestAnimationFrame()
to animate the red rectangle as it moved across the canvas. The clearRect()
method was used to clear the previous frame before drawing the new one, ensuring smooth motion.
In contrast, here’s an example of animating an SVG element using CSS:
html<svg width="500" height="400">
<rect x="50" y="50" width="150" height="100" fill="red">
<animate attributeName="x" from="50" to="400" dur="2s" repeatCount="indefinite" />
</rect>
</svg>
The animate
tag allows Sarah to move the rectangle across the screen. This approach felt simpler than Canvas since Sarah didn’t need to worry about redrawing the graphic on every frame.
Chapter 5: When to Use Canvas and When to Use SVG
By now, Sarah was familiar with both technologies. Here’s a summary of what she learned:
Use Canvas when:
- You need to control individual pixels.
- You’re building complex animations, games, or real-time visualizations.
- You don’t need your graphics to scale indefinitely.
Use SVG when:
- You need resolution-independent, responsive graphics.
- You’re working with static or simple animated vector graphics (like logos or icons).
- You want to apply CSS styling and make use of SMIL animations.
Chapter 6: Advanced Tips and Tricks
Sarah had become quite comfortable with both Canvas and SVG, but there were a few advanced tips that helped her elevate her work:
For Canvas, optimize performance by reducing the number of redraws. Use
requestAnimationFrame()
instead ofsetTimeout()
for smoother animations.For SVG, combine paths to reduce the complexity of your design and improve performance on large files. Use tools like SVGOMG to optimize SVG files for the web.
Use fallback content: When supporting older browsers, provide alternative content if Canvas or SVG isn't supported:
html<canvas id="myCanvas">Your browser does not support the Canvas element.</canvas> <svg>Your browser does not support the SVG element.</svg>
Conclusion: Sarah’s Final Choice
In the end, Sarah used a combination of both technologies. She used Canvas for the interactive parts of the website and SVG for the client’s logo and icons. Both tools allowed her to create a rich, interactive web experience, ensuring her client’s website was fast, scalable, and responsive.
By understanding the strengths and limitations of both Canvas and SVG, Sarah was able to make informed decisions for different parts of her project. Whether you’re building games, animations, logos, or responsive designs, mastering these tools will open up a world of possibilities for your web development projects.