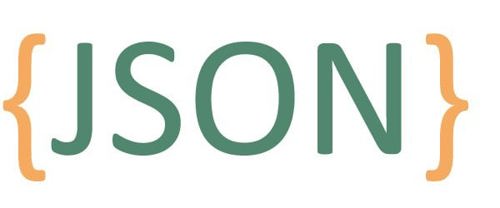
Meet Tobi, a budding web developer with a deep curiosity for learning. Like many beginners, he started with HTML and CSS but soon realized the need to communicate with servers and pass data effectively between different systems. That’s when he stumbled upon JavaScript Object Notation (JSON) – the key to simplifying data transfer between servers and web applications.
In this post, we’ll walk with Tobi on his journey to mastering JSON, from understanding the basics to leveraging advanced concepts. We’ll also include step-by-step solutions, real-life examples, and source code to guide you through the world of JSON.
Chapter 1: What is JSON?
JSON stands for JavaScript Object Notation. It’s a lightweight data format commonly used for exchanging data between a server and a web application. Tobi first encountered JSON while building his first API-based application.
Imagine JSON as a universal language that allows systems to talk to each other. For instance, if you wanted to build a weather app, the server would send you data about temperature, humidity, and conditions in JSON format. The app then interprets this data and displays it to the user.
Here’s a simple example of JSON:
json
{
"name": "Tobi",
"age": 25,
"skills": ["HTML", "CSS", "JavaScript"],
"employed": true
}
In the above JSON object:
"name" is a key with the value "Tobi"
"age" is a key with the value 25
"skills" is an array containing "HTML", "CSS", and "JavaScript"
"employed" is a boolean with the value true
This data format is easy to read and write for humans and machines. Tobi felt a sigh of relief when he saw how clear JSON was compared to other formats like XML.
Chapter 2: Getting Started with JSON in JavaScript
After understanding what JSON is, Tobi wondered, How do I use this in JavaScript?
In JavaScript, you can convert objects to JSON strings and vice versa using two core methods: JSON.stringify() and JSON.parse().
Convert JavaScript Object to JSON
Tobi used JSON.stringify() to convert his JavaScript object into JSON format:
javascript
let user = {
name: "Tobi",
age: 25,
skills: ["HTML", "CSS", "JavaScript"]
};
let jsonString = JSON.stringify(user);
console.log(jsonString);
Output:
json
{"name":"Tobi","age":25,"skills":["HTML","CSS","JavaScript"]}
Convert JSON String to JavaScript Object
Tobi also learned how to convert JSON back into a JavaScript object using JSON.parse():
javascript
let jsonData = '{"name":"Tobi","age":25,"skills":["HTML","CSS","JavaScript"]}';
let userObj = JSON.parse(jsonData);
console.log(userObj);
Output:
javascript
{ name: 'Tobi', age: 25, skills: [ 'HTML', 'CSS', 'JavaScript' ] }
Chapter 3: Common Uses of JSON in Web Development
Tobi realized that JSON is used everywhere in web development – from APIs to configurations and even database storage. Here are a few common uses:
Exchanging Data between Client and Server: JSON is most commonly used to exchange data between the client (browser) and server. This is especially important when working with RESTful APIs.
Configuration Files: JSON can also be used for configuration files. For instance, many JavaScript libraries use package.json to store important details about the project.
Database Storage: NoSQL databases like MongoDB store data in JSON-like formats, making it easier to work with data as objects.
Chapter 4: Advanced Concepts: Nested JSON and Arrays
As Tobi grew more confident, he tackled more complex JSON structures, such as nested JSON objects and arrays. Here’s an example of nested JSON:
In this example, the key "contacts" contains another JSON object, allowing you to represent complex, hierarchical data.
Tobi also worked with arrays inside JSON objects:
In the above structure, "projects" is an array that contains two objects, each representing a project.
Chapter 5: JSON in Real-Life Example
Let’s say Tobi is building a To-Do list app. Every time a user adds a new task, Tobi stores the task details in JSON format:
let jsonTask = JSON.stringify(task);
localStorage.setItem("task1", jsonTask);
// Retrieve and parse the JSON back into an object
let storedTask = JSON.parse(localStorage.getItem("task1"));
console.log(storedTask.description); // Output: Complete JSON tutorial
In this example, Tobi saves the task in localStorage as a JSON string, and retrieves it later by parsing it back into an object.
Chapter 6: Tips and Best Practices for Beginners to Advanced Developers
Use JSON Validators: Always validate your JSON to avoid syntax errors. You can use tools like jsonlint to check your JSON format.
Handle Errors: Use try-catch blocks when parsing JSON to handle potential errors. Badly formatted JSON can crash your program if not handled properly.
Leverage Modern Tools: Advanced developers can use libraries like axios or fetch to work with JSON APIs. JSON is widely used in modern frameworks such as React, Angular, and Vue.js.
Conclusion
Tobi's journey from a beginner to an advanced JSON user shows that understanding and working with JSON is critical for any web developer. Whether you’re exchanging data between client and server or working with configuration files, JSON simplifies the process. By mastering concepts like JSON.stringify(), JSON.parse(), and nested JSON, you can take your development skills to the next level.