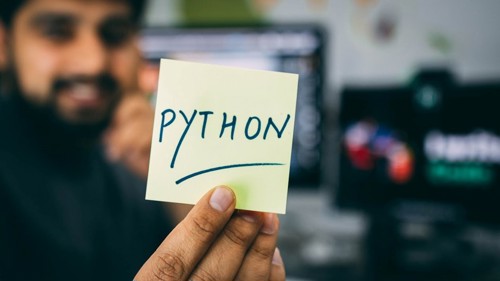
As a Python developer, your productivity and effectiveness often depend on the tools you use. Python’s ecosystem of packages makes it possible to streamline workflows, handle complex tasks, and tackle a wide range of challenges with ease. Whether you're working on web development, data science, automation, or machine learning, there’s a package for almost every need.
In this blog post, we’ll explore 50 essential Python packages that every developer should have in their toolkit. I’ll also provide real-life examples and step-by-step solutions to help you understand their practical applications.
1. Requests (HTTP Library)
What it does: Allows you to send HTTP requests easily.
Real-life Example: Let’s say you need to fetch data from an API:
pythonimport requests
response = requests.get('https://api.github.com')
print(response.json())
2. Flask (Web Development Framework)
What it does: Flask is a lightweight web framework that lets you build web applications quickly.
Example: A simple "Hello World" web server:
pythonfrom flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
3. Django (Full-stack Web Framework)
What it does: Django is a high-level web framework that encourages rapid development with a clean and pragmatic design.
Real-life Example: If you’re building an eCommerce platform, Django handles both the front-end and back-end, complete with an admin panel, user authentication, and database management.
4. NumPy (Scientific Computing)
What it does: Provides support for large, multi-dimensional arrays and matrices.
Example: Working with arrays and performing operations like matrix multiplication.
pythonimport numpy as np
matrix = np.array([[1, 2], [3, 4]])
print(np.matmul(matrix, matrix))
5. Pandas (Data Manipulation and Analysis)
What it does: Simplifies working with structured data, especially for data analysis.
Real-life Example: Handling large CSV files for data analysis.
pythonimport pandas as pd
df = pd.read_csv('data.csv')
print(df.head())
6. Matplotlib (Data Visualization)
What it does: A plotting library for visualizing data.
Example: Creating a simple line graph.
pythonimport matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [10, 20, 25, 30]
plt.plot(x, y)
plt.show()
7. Scikit-learn (Machine Learning)
What it does: Provides simple and efficient tools for data mining and machine learning.
Example: Training a simple model to predict housing prices.
pythonfrom sklearn.datasets import load_boston
from sklearn.linear_model import LinearRegression
boston = load_boston()
X = boston.data
y = boston.target
model = LinearRegression()
model.fit(X, y)
8. BeautifulSoup (Web Scraping)
What it does: Parses HTML and XML documents.
Example: Extracting all the links from a webpage.
pythonfrom bs4 import BeautifulSoup
import requests
page = requests.get("https://example.com")
soup = BeautifulSoup(page.content, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
9. Selenium (Web Automation)
What it does: Automates browser interaction, useful for web scraping and testing.
Example: Automating login to a website.
pythonfrom selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com/login')
driver.find_element_by_id('username').send_keys('your_username')
driver.find_element_by_id('password').send_keys('your_password')
driver.find_element_by_id('submit').click()
10. Pytest (Testing)
What it does: A testing framework for Python.
Example: Running unit tests for your project.
pythondef add(x, y):
return x + y
def test_add():
assert add(1, 2) == 3
11. Jupyter Notebook (Interactive Computing)
What it does: An open-source web application that allows you to create and share live code, equations, visualizations, and more.
Example: Ideal for experimenting with data science and machine learning projects.
12. Pillow (Image Processing)
What it does: Adds image processing capabilities.
Example: Resizing images.
pythonfrom PIL import Image
img = Image.open('image.jpg')
img_resized = img.resize((100, 100))
img_resized.save('resized_image.jpg')
13. Pygame (Game Development)
What it does: Helps in developing 2D games.
Example: Building a simple game where a player moves a character with the arrow keys.
14. Twilio (SMS and Voice APIs)
What it does: Enables sending SMS and voice messages.
Example: Automating SMS notifications for users.
15. SQLAlchemy (Database Management)
What it does: ORM (Object Relational Mapper) for databases.
Example: Working with databases in Python without writing SQL queries.
16. Bokeh (Interactive Visualization)
What it does: Provides interactive plots for modern web browsers.
17. OpenCV (Computer Vision)
What it does: Offers tools for computer vision applications.
18. Keras (Deep Learning)
What it does: High-level neural networks API.
19. TensorFlow (Machine Learning)
What it does: A complete machine learning framework for building and deploying machine learning models.
20. Scrapy (Web Scraping)
What it does: An efficient framework for scraping websites.