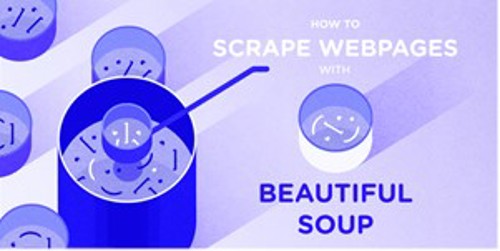
Imagine you’re running a small business that sells consumer electronics. You want to stay competitive, but manually checking the prices on your competitors' websites every day is time-consuming. This is where web scraping can come to the rescue. In this blog post, we'll walk through building a basic web scraping script to automate the extraction of data for market research or price comparison. We'll explore real-life use cases, the technology behind web scraping, and how to create your own.
What is Web Scraping?
Web scraping refers to the automated extraction of data from websites. It involves writing scripts or using tools that visit websites, pull data (like prices, reviews, or product names), and then store or analyze it.
Real-life Example: Let’s say you’re running an e-commerce store selling smartphones in Nigeria, and you want to compare prices across popular platforms like Jumia and Konga. Instead of visiting both sites manually, you can use a scraping script to get the prices daily, helping you make informed pricing decisions.
Why is Web Scraping Useful?
- Market Research: Extract trends, prices, and other valuable information from competitors’ websites.
- Price Comparison: Collect data from various vendors to compare and offer competitive prices.
- Lead Generation: Extract contact information like emails or phone numbers from directories for business outreach.
- Content Aggregation: Automatically pull content from different sources for news or blog updates.
Step-by-Step Guide: How to Build a Web Scraping Script
Let’s break down how to write a Python script to scrape product prices from an e-commerce website. We’ll use BeautifulSoup and Requests for this task.
Step 1: Install the Necessary Libraries
You’ll need two Python libraries to begin:
- Requests: Used to send HTTP requests to the website and retrieve the webpage’s content.
- BeautifulSoup: A library for parsing HTML and XML documents.
To install these libraries, run the following commands:
bashpip install requests pip install beautifulsoup4
Step 2: Choose a Website to Scrape
For this example, we’ll scrape the Jumia website to collect data on the prices of smartphones. We will extract details like product names, prices, and links to the product pages.
Step 3: Inspect the Website Structure
Before writing any code, visit the website you wish to scrape. Right-click on the page and select "Inspect" (or press F12). This opens the Developer Tools, allowing you to examine the structure of the HTML elements.
For Jumia, the product listings are contained within specific div tags. By identifying the right HTML elements, we can tell our script what data to extract.
Step 4: Write the Web Scraping Script
Here's a simple Python script that extracts the names and prices of smartphones from Jumia:
pythonimport requests
from bs4 import BeautifulSoup
# URL of the Jumia smartphone section
url = 'https://www.jumia.com.ng/smartphones/'
# Send a request to the website and retrieve the page content
response = requests.get(url)
# Parse the HTML content with BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
# Find all product listings on the page
products = soup.find_all('div', class_='sku -gallery')
# Loop through each product and extract the name and price
for product in products:
name = product.find('span', class_='name').text
price = product.find('span', class_='price').text
print(f'Product Name: {name}')
print(f'Price: {price}')
print('-' * 20)
Step 5: Run the Script
When you run this script, it will extract the product names and prices from the Jumia smartphone section. This can be stored in a file or database for further analysis.
Step 6: Storing the Data
You may want to save the scraped data into a CSV or JSON file for future use. Here’s how to store the data in a CSV file:
pythonimport csv
# Open a CSV file to write the data
with open('jumia_smartphones.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Product Name', 'Price'])
# Loop through each product and write the data to the CSV
for product in products:
name = product.find('span', class_='name').text
price = product.find('span', class_='price').text
writer.writerow([name, price])
Overcoming Challenges with Web Scraping
- Website Changes: Websites often update their structure, breaking your scraper. Regularly check and update your scraping logic.
- CAPTCHA: Some websites use CAPTCHA to block automated requests. In such cases, you may need to use advanced techniques like headless browsers or proxy rotation.
- Legal Issues: Always check a website's robots.txt file to ensure you are not violating their terms of service by scraping.
Advanced Techniques
Once you’ve mastered basic scraping, you can move on to more advanced techniques like:
- Using APIs: Some websites offer APIs that provide structured data for free or via subscription. These are often easier to work with than HTML scraping.
- Headless Browsers: Use tools like Selenium to scrape data from websites that rely on JavaScript for rendering.
- Data Cleaning and Analysis: After scraping the data, use libraries like Pandas to clean and analyze the data for actionable insights.
Real-Life Use Cases
Price Comparison Site: Suppose you're setting up a price comparison website for Nigerian smartphones. Using a web scraper, you can collect data from multiple e-commerce sites daily, providing users with the latest prices.
Market Research for New Product Launch: A business planning to launch a new gadget in Nigeria can use web scraping to gather market trends, competitor pricing, and consumer reviews from websites like Jumia and Konga.
Monitor Real Estate Trends: You could scrape real estate listing sites to track property prices in various Nigerian cities. This data can help buyers make informed decisions or assist real estate agents in adjusting their pricing strategies.
Conclusion
Web scraping is a powerful tool for automating the extraction of data from websites, offering endless opportunities for market research, price comparison, and other business intelligence tasks. By learning how to build your own scraping scripts, you can save time, gain valuable insights, and stay ahead in the competitive business landscape. However, always ensure that your scraping practices comply with legal guidelines and website policies.