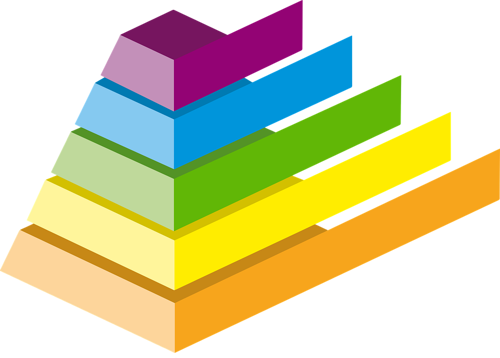
Data visualization is essential in the modern data-driven world, where businesses and organizations rely on insights to make decisions. Whether you're analyzing sales trends, financial performance, or customer behavior, tools like Matplotlib, Seaborn, and Plotly enable you to create visual representations of data that are easy to interpret and actionable.
In this post, we'll walk you through how to use these popular Python libraries to create interactive and informative visualizations. We’ll provide real-life examples, step-by-step guidance, and downloadable source code to get you started.
Step 1: Setting Up Your Environment
Before we begin creating visualizations, let's set up the Python environment by installing the necessary libraries:
bashpip install matplotlib seaborn plotly
These packages are essential for generating static and interactive plots. Matplotlib is the foundation for basic plots, Seaborn builds on top of Matplotlib to produce more sophisticated statistical plots, and Plotly allows for interactive plots.
Step 2: Visualizing Data with Matplotlib
Matplotlib is one of the most widely used plotting libraries in Python. It’s highly customizable, making it suitable for everything from basic line graphs to complex 3D plots.
Real-Life Example: Sales Trend Visualization
Let's start by plotting a sales trend over time using Matplotlib.
pythonimport matplotlib.pyplot as plt
import pandas as pd
# Sample data: Monthly sales over the course of a year
months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
sales = [1500, 1800, 2200, 2600, 3000, 3500, 4000, 3700, 4200, 4800, 5000, 5500]
# Creating the plot
plt.plot(months, sales, marker='o')
# Adding title and labels
plt.title('Monthly Sales Trend')
plt.xlabel('Month')
plt.ylabel('Sales (in USD)')
# Display the plot
plt.show()
In this example, we plot a simple line graph of sales trends across months. This can be used in a business context to track performance and identify peak sales periods.
Step 3: Enhancing Visualizations with Seaborn
Seaborn builds on top of Matplotlib to make visualizations more attractive and easier to interpret. It offers high-level abstractions for creating plots like heatmaps, bar plots, and violin plots.
Real-Life Example: Visualizing a Correlation Matrix
Correlation matrices help businesses understand relationships between multiple variables. For example, a retail company may want to analyze the correlation between sales, advertisement spending, and customer visits.
pythonimport seaborn as sns
import numpy as np
# Sample data: Correlation between variables
data = pd.DataFrame({
'Sales': [200, 300, 500, 700, 1000],
'Ad Spend': [100, 150, 300, 450, 600],
'Customer Visits': [400, 500, 600, 800, 1000]
})
# Compute the correlation matrix
corr = data.corr()
# Plot the heatmap
sns.heatmap(corr, annot=True, cmap='coolwarm')
# Adding title
plt.title('Correlation Matrix')
# Display the plot
plt.show()
This example produces a heatmap showing how different variables (e.g., sales, ad spend, and customer visits) are correlated. Such visualizations are valuable when making data-driven marketing decisions.
Step 4: Creating Interactive Visualizations with Plotly
Plotly offers the ability to create interactive visualizations that allow users to hover over data points, zoom in, and explore the data more thoroughly. This is particularly useful in presentations or dashboards.
Real-Life Example: Interactive Sales Bar Chart
Let's create an interactive bar chart to visualize quarterly sales using Plotly.
pythonimport plotly.graph_objects as go
# Sample data: Quarterly sales
quarters = ['Q1', 'Q2', 'Q3', 'Q4']
sales = [12000, 15000, 18000, 22000]
# Creating the bar chart
fig = go.Figure([go.Bar(x=quarters, y=sales)])
# Adding title and labels
fig.update_layout(
title='Quarterly Sales Performance',
xaxis_title='Quarter',
yaxis_title='Sales (in USD)'
)
# Display the plot
fig.show()
Here, Plotly’s interactivity allows users to hover over each bar and see the exact sales figure. This feature is especially helpful in a business dashboard, where decision-makers need to engage with the data more dynamically.
Step 5: Combining Libraries for Advanced Data Visualization
Often, combining libraries can yield even more powerful visualizations. For example, you can use Seaborn for statistical visualizations and Plotly for interactivity.
Real-Life Example: Visualizing Product Sales Across Regions
A company may want to visualize how sales are distributed across different regions and compare it with a target.
pythonimport plotly.express as px
import seaborn as sns
# Sample data: Product sales by region
regions = ['North', 'South', 'East', 'West']
sales = [3500, 2700, 4000, 3200]
target = [3000, 3000, 4000, 3000]
# Plot using Plotly
fig = px.bar(x=regions, y=sales, title='Sales by Region', labels={'x':'Region', 'y':'Sales (USD)'})
fig.add_trace(go.Scatter(x=regions, y=target, mode='lines+markers', name='Target'))
# Show interactive plot
fig.show()
# Using Seaborn for deeper statistical analysis
sns.barplot(x=regions, y=sales)
plt.title('Sales by Region')
plt.show()
In this example, we use Plotly to create an interactive bar chart that compares sales and target values across regions, and we use Seaborn to add further statistical analysis. Combining the two libraries can create rich, multi-dimensional visualizations.
Conclusion: Best Practices for Python Data Visualization
- Choose the right tool: Use Matplotlib for basic static plots, Seaborn for statistical plots, and Plotly for interactive visualizations.
- Keep it simple: Avoid over-complicating the visualization. The goal is to communicate the insights effectively.
- Focus on the audience: Tailor your visualization to the audience's needs. Decision-makers may prefer simple visuals, while data scientists may prefer detailed statistical insights.
- Color palettes: Choose clear and contrasting color palettes, especially when displaying complex data.
With Python’s powerful libraries, creating insightful and interactive visualizations becomes a breeze, making it an essential tool for anyone working with data. By following the steps outlined here, you can start building your own data visualization pipeline that suits your business needs.