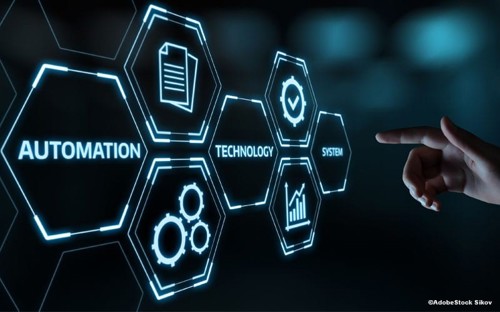
In today’s world, automation is not just a luxury—it’s a necessity. With endless repetitive tasks like sending emails, renaming files, or entering data, individuals and businesses spend a lot of time on routine activities. Fortunately, Python, a powerful and easy-to-learn programming language, provides an excellent platform for automating many such tasks.
In this blog, we’ll explore how to automate some of these daily tasks with Python, using real-life examples. Whether you're managing emails, organizing files, or dealing with endless spreadsheets, you’ll find that Python scripts can save you valuable time and effort.
Real-Life Scenario 1: Automating Email Sending
Imagine you are running a small online business and often need to send hundreds of emails to customers, vendors, or partners. Doing this manually is time-consuming and prone to errors. With Python, you can automate this task using the smtplib
library.
Step-by-Step Guide: Sending Emails with Python
Step 1: Import the Necessary Libraries
You'll need the smtplib
and email.mime
libraries to send emails.
pythonimport smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
Step 2: Set Up the Email Server
You'll be connecting to your email provider’s SMTP server. For example, if you're using Gmail:
python# Set up the server connection
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
# Log in to your email account
email = 'your_email@gmail.com'
password = 'your_password'
server.login(email, password)
Step 3: Create the Email Message
You can now construct the email, specifying the recipient, subject, and body content.
python# Create the message
msg = MIMEMultipart()
msg['From'] = email
msg['To'] = 'recipient@example.com'
msg['Subject'] = 'Automated Email'
body = 'Hello, this is an automated email sent by a Python script.'
msg.attach(MIMEText(body, 'plain'))
Step 4: Send the Email
Finally, send the email and close the server connection.
pythonserver.sendmail(email, 'recipient@example.com', msg.as_string())
server.quit()
With this simple script, you can now automate the process of sending emails to one or multiple recipients by modifying the recipient address in a loop.
Real-Life Scenario 2: Renaming Files in Bulk
You might find yourself in a situation where you have hundreds of files that need renaming. For example, photographers often end up with a set of files like IMG001.jpg
, IMG002.jpg
, and so on. Manually renaming these files to something more descriptive is tedious, but a Python script can handle this task effortlessly.
Step-by-Step Guide: Renaming Files in Python
Step 1: Import the Necessary Libraries
You'll need the os
library to interact with the file system.
pythonimport os
Step 2: Specify the Directory and Files
Set the directory where the files are located and iterate through them.
python# Path to the directory containing the files
directory = '/path/to/your/files/'
# Iterate through each file in the directory
for filename in os.listdir(directory):
if filename.endswith('.jpg'):
new_name = f"photo_{filename[-7:-4]}.jpg" # Renaming logic
os.rename(os.path.join(directory, filename), os.path.join(directory, new_name))
print(f'Renamed: {filename} to {new_name}')
In this example, the script renames each .jpg
file in the directory to something like photo_001.jpg
, photo_002.jpg
, and so on.
Step 3: Run the Script
Once the script is executed, all the files will be renamed according to the pattern you defined, saving hours of manual labor.
Real-Life Scenario 3: Automating Data Entry with Python
Data entry is another task ripe for automation. Let's say you're constantly copying information from spreadsheets into a database or another document. With Python, you can use libraries like pandas
and openpyxl
to automate this process.
Step-by-Step Guide: Automating Data Entry
Step 1: Install and Import Libraries
You'll need pandas
to handle spreadsheets and openpyxl
to work with Excel files.
bashpip install pandas openpyxl
pythonimport pandas as pd
Step 2: Load the Spreadsheet
Load an Excel spreadsheet into a DataFrame, a data structure in Python for managing tabular data.
python# Load the Excel file
df = pd.read_excel('data.xlsx', engine='openpyxl')
# Display the first few rows of the data
print(df.head())
Step 3: Process and Enter Data Automatically
Now, let's assume you want to transfer specific data from the spreadsheet into another application. You can extract the required data and use Python’s automation capabilities to feed it into a web form or a database.
python# Filter and process the data (e.g., selecting rows where 'status' is 'active')
filtered_data = df[df['status'] == 'active']
# Iterate over the filtered data and perform tasks like entering it into a database
for index, row in filtered_data.iterrows():
name = row['name']
email = row['email']
print(f'Processing: {name}, {email}')
# You can use APIs or other methods to input this data into another system
Real-Life Use Cases
Email Campaigns: Marketing teams can use Python scripts to automate email campaigns. Instead of manually sending newsletters to thousands of subscribers, a Python script can loop through a list of recipients and send personalized emails in seconds.
File Management for Digital Agencies: Graphic designers or video production houses often deal with hundreds of files daily. Automating tasks like renaming files, organizing them into directories, or even converting file formats can save them significant time and effort.
Data Entry for Research: Researchers often deal with massive datasets in Excel or CSV files. Instead of manually inputting the data into another system, they can use Python scripts to automate this, ensuring that the process is quick and error-free.
Why Automate with Python?
Efficiency: Once set up, Python scripts can handle large volumes of tasks in a fraction of the time it takes to do them manually.
Accuracy: Human errors can creep in when performing repetitive tasks like entering data or renaming files. Automation ensures that tasks are done consistently and without mistakes.
Scalability: Whether you're dealing with a few tasks or thousands, Python can scale to meet your needs.
Flexibility: Python offers libraries for almost every kind of task, from sending emails to interacting with APIs. You can tailor your script to meet specific requirements and adapt it as your business grows.