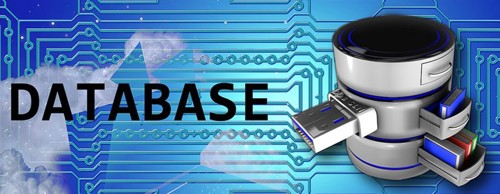
Database management is a critical aspect of application development, data analysis, and business operations. As a developer or data analyst, interacting with databases efficiently is crucial to ensure data integrity, consistency, and performance. In this post, we’ll explore the best practices for using Python to interact with databases, perform queries, and migrate data. Using real-life examples and Python libraries like SQLite3, SQLAlchemy, and Pandas, you can easily streamline these tasks.
Let’s take a practical approach, going step-by-step into how to manage databases and handle data efficiently with Python.
Step 1: Setting Up a Python Environment for Database Interaction
Before diving into querying or migrating data, we need to set up the environment for database management in Python. Depending on the database you're working with, you can use different libraries like:
- SQLite: A lightweight database for small to medium-sized applications.
- MySQL or PostgreSQL: For more robust, scalable databases.
- SQLAlchemy: A popular ORM (Object Relational Mapper) that helps you interact with SQL databases in an object-oriented way.
Let’s first install some of the most common database libraries:
bashpip install sqlite3 pandas sqlalchemy psycopg2
Step 2: Database Connection and Setup Using SQLite
For this example, we’ll use SQLite because it’s built into Python and is great for small applications or local storage. Let’s create a connection to an SQLite database and perform basic queries.
Connecting to the Database
pythonimport sqlite3
# Establish a connection to SQLite database
conn = sqlite3.connect('example.db')
# Create a cursor object to interact with the database
cursor = conn.cursor()
# Create a simple table
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
Here, we’re creating a table called users
, with columns for id
, name
, and age
. This setup can be used for a small-scale user management system.
Step 3: Inserting Data into the Database
Let’s insert some data into our users
table to illustrate how you can populate a database.
python# Insert sample data into the users table
cursor.execute("INSERT INTO users (name, age) VALUES ('John Doe', 30)")
cursor.execute("INSERT INTO users (name, age) VALUES ('Jane Doe', 25)")
# Commit the changes
conn.commit()
By using SQL queries, we’ve inserted two new users into our database. This is particularly useful for real-world applications such as CRM (Customer Relationship Management) tools, where user data needs to be stored and managed.
Step 4: Querying Data from the Database
Let’s retrieve data from the database and print it.
python# Retrieve all users from the database
cursor.execute("SELECT * FROM users")
# Fetch all the results
rows = cursor.fetchall()
# Print out each row
for row in rows:
print(row)
In this example, we’ve fetched all rows from the users
table and printed them. This basic operation is essential for displaying data in web apps, admin dashboards, or data reporting tools.
Real-life Example: Sales Dashboard
Imagine a small retail business wants to use a sales dashboard to track customers and their purchases. You can build an admin panel using Python and a web framework like Flask, where admins query the database and generate reports on sales.
Step 5: Using SQLAlchemy for Advanced Database Interactions
For more complex database operations and when you need to support multiple databases (e.g., SQLite, MySQL, or PostgreSQL), SQLAlchemy is a great tool. It allows you to use Python objects to interact with the database, simplifying CRUD (Create, Read, Update, Delete) operations.
Setting Up SQLAlchemy
pythonfrom sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Create a SQLite engine
engine = create_engine('sqlite:///example.db', echo=True)
# Define a base class for declarative models
Base = declarative_base()
# Define a User model
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
# Create the users table
Base.metadata.create_all(engine)
# Create a session
Session = sessionmaker(bind=engine)
session = Session()
Here, we’ve defined a User class that maps to the users
table in the database. With SQLAlchemy, the interaction with the database is much more streamlined, making it easy to create, read, update, and delete records.
Step 6: Migrating Data
Migrating data between databases or within the same database (e.g., adding columns, renaming tables) is a common task in database management.
Example: Migrating Data Using Pandas
Let’s say you have data in a CSV file, and you want to migrate it to a database.
pythonimport pandas as pd
# Load CSV data
data = pd.read_csv('users.csv')
# Insert data into the SQLite database
data.to_sql('users', conn, if_exists='append', index=False)
In this example, we’re using Pandas to load a CSV file and migrate the data into the users
table in the database. This is highly useful for real-life scenarios like importing customer data or migrating legacy databases to a new system.
Step 7: Performing Queries and Data Analysis
Once data is in the database, you can perform powerful queries and even combine them with data analysis techniques.
Example: Query and Analyze Data
python# Retrieve all users who are older than 25
cursor.execute("SELECT * FROM users WHERE age > 25")
rows = cursor.fetchall()
# Print the results
for row in rows:
print(row)
Let’s say you’re building a customer segmentation tool for a marketing team. By querying the database for users of certain demographics (e.g., age), you can tailor marketing strategies based on data-driven insights.
Conclusion: Best Practices for Managing Databases with Python
Whether you’re building a small application, a complex CRM system, or an analytics tool, interacting with databases through Python can be highly efficient. Here are some best practices to keep in mind:
- Use ORM for complex applications: For large-scale projects, use ORM tools like SQLAlchemy to simplify database interactions.
- Backup before migration: Always back up your data before migrating, whether it’s within the same database or between databases.
- Query optimization: Optimize your queries by using indexes and only retrieving the data you need.
- Data integrity: Always ensure data integrity through validations both on the application and database side.
- Documentation: Maintain clear documentation for all migrations and database changes to avoid confusion during updates.
By following these best practices and using Python libraries like SQLite3, SQLAlchemy, and Pandas, you’ll be able to interact with databases, perform efficient queries, and migrate data seamlessly in real-world applications.