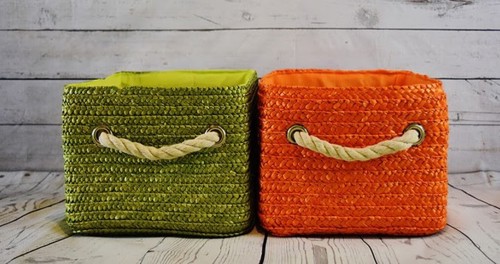
It was a sunny afternoon when Tobi, a young programmer, found himself stuck on a basic programming task. His task seemed simple: create a program that calculated the total cost of groceries after adding tax. But the term "variable" kept popping up in the tutorials, and he wasn't sure what it meant or how it worked. As he sat there, lost in thought, he noticed his grocery list on the table. That's when the light bulb went off.
"Variables," he realized, "are like placeholders for my groceries. They store information just like this list stores the items I need to buy."
Let’s dive into what variables are in programming, using real-life examples to break down this fundamental concept step by step.
What is a Variable in Programming?
In programming, a variable is like a box where you store data or values that can change over time. This data could be numbers, words, or even more complex structures. You give this box a name so you can easily refer to it later in your program.
Think of it this way: When you go shopping, you need a way to remember what items to buy. You write down the names of the items and the quantities you need. Each item on the list is a placeholder (or "variable") for the actual product you’ll buy at the store.
How Variables Work in Real Life: A Grocery List Example
Imagine you’re creating a grocery list with the following items:
- 2 loaves of bread
- 1 pack of eggs
- 3 liters of milk
In a programming sense, your list could look something like this:
bread = 2
eggs = 1
milk = 3
Here, "bread", "eggs", and "milk" are the variables, while the numbers 2, 1, and 3 represent the values stored in those variables. Later, if you need to calculate the total cost, you can reference the variables (just like you’d reference your grocery list in the store).
Step-by-Step: How to Use Variables in Programming
1. Declaring a Variable
Declaring a variable is like creating a box and giving it a label. In most programming languages, you start by choosing a name for your variable. This name should be descriptive, so it’s clear what the variable represents.
For example, in Python, you might declare a variable for the price of bread like this:
pythonbread_price = 200 # The price of a loaf of bread in ₦
In this case, "bread_price" is the variable, and 200 is the value stored in it (let’s assume bread costs ₦200).
2. Assigning a Value to a Variable
Assigning a value to a variable is just like adding something to your shopping list. You’re saying, "I need two loaves of bread," or "This loaf of bread costs ₦200."
In programming, you assign a value to a variable using the equals sign (=
):
pythonbread_price = 200 # Assigning the value 200 to the variable bread_price
If the price of bread changes tomorrow, you don’t need to rewrite your whole program. You can simply update the value of "bread_price":
pythonbread_price = 250 # The new price of bread
This flexibility makes variables extremely useful.
Using Variables in a Program: A Complete Example
Let’s follow Tobi as he writes a simple program to calculate the total cost of groceries. He needs to calculate the cost of 2 loaves of bread, 1 pack of eggs, and 3 liters of milk, given their prices:
- Bread: ₦200 per loaf
- Eggs: ₦500 per pack
- Milk: ₦150 per liter
Tobi’s program looks like this:
python# Declaring the variables and their values
bread_price = 200
eggs_price = 500
milk_price = 150
# Declaring the quantity of each item
bread_quantity = 2
eggs_quantity = 1
milk_quantity = 3
# Calculating the total cost
total_cost = (bread_price * bread_quantity) + (eggs_price * eggs_quantity) + (milk_price * milk_quantity)
# Output the total cost
print("Total cost of groceries is ₦", total_cost)
In this program:
- bread_price, eggs_price, and milk_price store the prices of the items.
- bread_quantity, eggs_quantity, and milk_quantity store the quantities of each item.
- total_cost calculates the final cost by multiplying the price and quantity of each item and summing them.
Why Variables Are Important in Programming
Variables are one of the most important concepts in programming because they allow you to:
- Store Data: Variables store information that can be used and manipulated throughout a program.
- Reuse Data: Once a value is stored in a variable, you can use it multiple times without rewriting the data.
- Make Changes Easily: If something changes (like the price of bread), you can update the value of a variable without changing the entire program.
- Improve Readability: Variables with descriptive names make programs easier to read and understand.
Real-Life Example of Variables: Tracking Finances
Now that Tobi understood variables, he began to see them everywhere in his daily life. He thought about how he tracks his monthly budget. He might create variables like this:
pythonrent = 15000 # Rent costs ₦15,000 per month
electricity = 5000 # Electricity costs ₦5,000 per month
groceries = 10000 # Groceries cost ₦10,000 per month
total_expenses = rent + electricity + groceries # Calculating the total expenses
print("Total monthly expenses: ₦", total_expenses)
In this example:
- rent, electricity, and groceries are variables that store different expenses.
- The variable total_expenses adds them all together, allowing Tobi to see his total monthly costs at a glance.
How Variables Evolve with Programming
As Tobi advanced in his programming journey, he learned that variables could store more than just numbers. Variables can also store:
- Strings (Text): Names, addresses, or any form of text.
- Booleans (True/False): Useful in conditional statements.
- Lists or Arrays: Collections of data, like a list of grocery items.
For example, in Python, a variable could store a list like this:
pythongrocery_list = ["bread", "eggs", "milk"]
Tobi could now track his grocery items, expenses, and much more—all thanks to variables.
Conclusion: Why Variables Matter in Programming and Life
Just like Tobi realized, variables are essential in both programming and everyday tasks. They help store and manipulate data in ways that make our lives more organized and efficient. Whether you’re programming a chatbot, managing finances, or just creating a shopping list, variables are the building blocks that bring order to information.