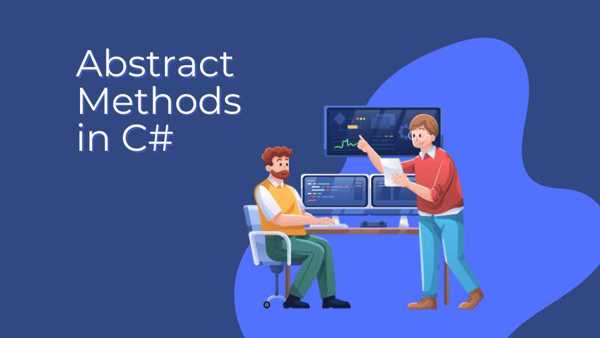
Abstract methods in C# are like "blueprints" for methods that must be defined by derived (child) classes. Think of an abstract method as a rule that says, "Every class that inherits from me must have this method, but I won't tell you how to implement it." It’s like a parent saying to their child, "You must learn to cook, but I won't teach you how—you figure it out yourself."
Real-Life Example:
Imagine you’re designing a game with different types of characters: warriors, mages, and archers. Each character has a unique way of attacking, but you want to ensure that every character has an Attack
method. You can define an abstract Attack
method in a base class (e.g., Character
), and each specific character class (e.g., Warrior
, Mage
) will provide its own implementation of how the attack works.
Advanced Code Example
Here’s how you can use abstract methods in C#:
using System; // Base class with an abstract method abstract class Character { // Abstract method: no implementation, must be overridden by derived classes public abstract void Attack(); // Regular method with implementation public void Walk() { Console.WriteLine("The character is walking."); } } // Derived class: Warrior class Warrior : Character { // Implementation of the abstract method public override void Attack() { Console.WriteLine("Warrior slashes with a sword!"); } } // Derived class: Mage class Mage : Character { // Implementation of the abstract method public override void Attack() { Console.WriteLine("Mage casts a fireball!"); } } class Program { static void Main(string[] args) { // Create instances of derived classes Character warrior = new Warrior(); Character mage = new Mage(); // Call the abstract method (implementation depends on the derived class) warrior.Attack(); // Output: Warrior slashes with a sword! mage.Attack(); // Output: Mage casts a fireball! // Call the regular method warrior.Walk(); // Output: The character is walking. } }
How it works:
- The
Character
class defines an abstract methodAttack()
and a regular methodWalk()
. - The
Warrior
andMage
classes inherit fromCharacter
and provide their own implementations of theAttack()
method. - When you call
Attack()
on aCharacter
object, the actual implementation depends on the derived class.
Best Practices and Features of Abstract Methods
- Enforce Implementation: Abstract methods ensure that derived classes provide specific functionality.
- Flexibility: Derived classes can implement the method in their own way, allowing for polymorphism.
- Base Class Logic: Abstract classes can include both abstract methods (no implementation) and regular methods (with implementation).
- Cannot Instantiate Abstract Classes: You cannot create an instance of an abstract class, which ensures it’s only used as a base class.
- Use with Interfaces: Abstract classes can be combined with interfaces to provide both default behavior and required functionality.
Pros and Cons of Abstract Methods
Pros:
- Code Reusability: Abstract classes allow you to define common behavior in the base class while letting derived classes implement specific behavior.
- Polymorphism: Abstract methods enable polymorphic behavior, where a base class reference can point to derived class objects.
- Enforces Structure: Ensures that all derived classes implement the required methods, reducing the risk of missing functionality.
Cons:
- Rigidity: Abstract classes create a tight coupling between the base class and derived classes, making the design less flexible.
- Single Inheritance: C# does not support multiple inheritance, so a class can only inherit from one abstract class.
- Complexity: Overusing abstract methods can make the code harder to understand and maintain.
Alternatives to Abstract Methods
- Interfaces: Use interfaces to define a contract that classes must implement. Unlike abstract classes, interfaces cannot provide any implementation.
interface ICharacter { void Attack(); } class Warrior : ICharacter { public void Attack() { Console.WriteLine("Warrior slashes with a sword!"); } }
- Virtual Methods: Use virtual methods in a base class if you want to provide a default implementation that can be overridden by derived classes.
- csharp
- Copy
class Character { public virtual void Attack() { Console.WriteLine("The character attacks!"); } } class Warrior : Character { public override void Attack() { Console.WriteLine("Warrior slashes with a sword!"); } }
When to Use Abstract Methods
Use abstract methods when:
- You want to enforce that derived classes implement specific functionality.
- You need to provide a common base class with some shared behavior but allow derived classes to define their own behavior.
- You want to achieve polymorphism, where a base class reference can point to objects of different derived classes.
Avoid abstract methods when:
- You don’t need to enforce method implementation (use regular or virtual methods instead).
- You need multiple inheritance (use interfaces instead).
- The design can be simplified without abstract classes.
Summary
Abstract methods in C# are a way to define a "contract" that derived classes must follow. They are useful for enforcing structure and enabling polymorphism but can introduce rigidity and complexity. Use abstract methods when you need to ensure specific behavior in derived classes, and consider alternatives like interfaces or virtual methods when flexibility is more important.