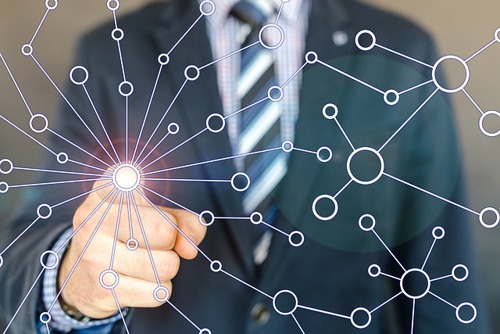
Chapter One: The Curious Programmer’s Journey into Deep Learning
It all began on a sunny afternoon when Ada, a passionate C# programmer, stumbled upon an article about Convolutional Neural Networks (CNNs). Ada had always been fascinated by artificial intelligence, but deep learning felt like uncharted territory for her. Her curiosity got the better of her, and she decided to explore how she could implement CNNs in C#.
As she delved deeper, Ada quickly realized that CNNs were the backbone of many real-world applications. From recognizing faces in photos to detecting cancerous cells in medical images, CNNs were revolutionizing industries. This was no ordinary algorithm; it was a key to solving complex problems.
What are Convolutional Neural Networks (CNNs)?
CNNs are a specialized class of deep learning algorithms designed to process and analyze visual data. Unlike traditional neural networks that deal with flat data, CNNs are capable of learning spatial hierarchies from images.
Imagine you’re tasked with recognizing different animals in a picture. Your brain naturally focuses on specific parts of the image—the ears, the tail, the overall shape—to identify whether it's a dog, cat, or bird. CNNs work similarly, breaking down images into smaller sections and recognizing patterns.
At its core, CNNs consist of three key layers:
- Convolutional Layer: This layer applies filters to the input image, detecting features like edges and textures. It’s like scanning the image bit by bit.
- Pooling Layer: This layer reduces the dimensionality of the data, making it easier for the model to process. Think of it as summarizing the most important parts of the image.
- Fully Connected Layer: After feature extraction, the network connects all the detected features and makes a final prediction. This is akin to putting all the puzzle pieces together to form a complete picture.
The Real-World Impact of CNNs
Ada marveled at how CNNs could solve real-world problems. One of the most impressive use cases she came across was autonomous driving. CNNs were being used in self-driving cars to identify pedestrians, road signs, and obstacles in real-time.
Another powerful application was in the medical field, where CNNs helped in diagnosing diseases. By analyzing medical scans, CNNs could detect anomalies that even trained doctors might miss, offering a second opinion that could save lives.
With this newfound knowledge, Ada was ready to try implementing CNNs in C#. She realized that while C# wasn't the most common language for deep learning, libraries like ML.NET and TensorFlow.NET could bring her vision to life.
Writing a CNN in C#: Step-by-Step Guide
Ada decided to tackle a simple image classification problem: recognizing handwritten digits using the famous MNIST dataset. Here’s how she did it.
Step 1: Setting Up the Environment
To start, Ada installed ML.NET and TensorFlow.NET. Using NuGet Package Manager in Visual Studio, she added the following packages:
bashdotnet add package Microsoft.ML dotnet add package SciSharp.TensorFlow.Redist
Step 2: Preparing the Data
Ada downloaded the MNIST dataset and wrote some code to load it into her application. The dataset consists of 28x28 grayscale images of handwritten digits (0-9).
csharppublic static (float[][], int[]) LoadData(string filePath)
{
// Load your data here
// This is where you preprocess the dataset into a format that ML.NET can understand
}
Step 3: Defining the CNN Model
Ada constructed the CNN model using the TensorFlow.NET library. She started by defining the layers:
csharpvar model = tf.keras.models.Sequential();
model.add(tf.keras.layers.Conv2D(32, (3, 3), activation: "relu", input_shape: (28, 28, 1)));
model.add(tf.keras.layers.MaxPooling2D(pool_size: (2, 2)));
model.add(tf.keras.layers.Conv2D(64, (3, 3), activation: "relu"));
model.add(tf.keras.layers.MaxPooling2D(pool_size: (2, 2)));
model.add(tf.keras.layers.Flatten());
model.add(tf.keras.layers.Dense(128, activation: "relu"));
model.add(tf.keras.layers.Dense(10, activation: "softmax"));
Here’s what each part of the code does:
- Conv2D Layer: This layer applies 32 filters, each of size 3x3, to the input image. The activation function
relu
ensures non-linearity, allowing the network to learn complex patterns. - MaxPooling Layer: This reduces the spatial dimensions of the image by selecting the most important features.
- Flatten Layer: Converts the 2D matrix into a 1D vector.
- Dense Layer: Fully connected layer to classify the image into one of 10 categories (0-9).
Step 4: Compiling the Model
Next, Ada compiled the model, specifying the optimizer, loss function, and metrics:
csharpmodel.compile(optimizer: "adam", loss: "categorical_crossentropy", metrics: new string[] { "accuracy" });
The optimizer helps adjust weights during training, while the loss function measures how well the model is performing. Accuracy was chosen as the evaluation metric.
Step 5: Training the Model
Ada trained the model using the MNIST dataset:
csharpmodel.fit(trainImages, trainLabels, batch_size: 64, epochs: 10, validation_data: (testImages, testLabels));
Here, the model is trained over 10 epochs with a batch size of 64. After training, Ada could test the model’s accuracy on new images.
Step 6: Evaluating the Model
Finally, Ada evaluated the model on the test dataset:
csharpvar score = model.evaluate(testImages, testLabels);
Console.WriteLine($"Test loss: {score[0]} / Test accuracy: {score[1]}");
Real-Life Example: Handwriting Recognition
Ada’s CNN could now recognize handwritten digits with impressive accuracy. She imagined this technology being applied to digitize old handwritten documents, automate postal systems, or even assist visually impaired people in reading handwritten text.
The success of her project made Ada realize that deep learning, even with C#, wasn’t just for academic purposes. It had real-world applications that could change lives.