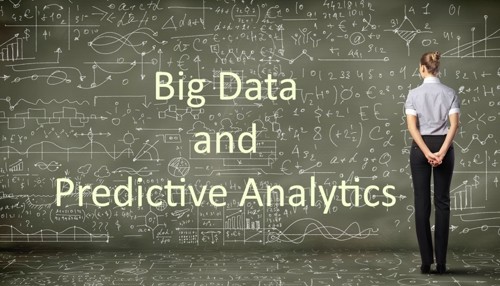
In the bustling city of Lagos, Emmanuel runs a small manufacturing plant. His factory produces essential machinery parts, but maintaining the equipment has always been a challenge. Unexpected breakdowns often halt production, leading to costly repairs and delays. Emmanuel knew there had to be a better way to predict equipment failures before they happened. That’s when he discovered predictive maintenance and decided to use C# and Azure Machine Learning to develop a solution.
This blog post will guide you through Emmanuel’s journey of implementing predictive maintenance using C# and Azure Machine Learning, complete with step-by-step solutions and source code.
Step 1: Setting Up Your Environment
To start with predictive maintenance, Emmanuel needed to set up his environment for using Azure Machine Learning with C#. Here’s how he did it:
Create an Azure Account: Emmanuel signed up for an Azure account at the Azure portal.
Set Up Azure Machine Learning Workspace: In the Azure portal, he created a new Machine Learning Workspace, which provided him with the necessary tools and resources for model development and deployment.
Install Azure SDK for .NET: Emmanuel needed the Azure SDK for .NET to interact with Azure services using C#. He installed the SDK via NuGet Package Manager in Visual Studio:
bashInstall-Package Microsoft.Azure.Management.MachineLearningServices
Install Other Required Packages: Emmanuel also installed additional packages such as
Microsoft.ML
for machine learning tasks in C#:bashInstall-Package Microsoft.ML
Step 2: Preparing the Data
Predictive maintenance relies heavily on historical data. Emmanuel gathered data from his factory’s sensors, which recorded metrics like temperature, vibration, and pressure. This data needed preprocessing before feeding it into the machine learning model.
Here’s how Emmanuel preprocessed the data using C#:
csharpusing System;
using System.Linq;
using Microsoft.ML;
using Microsoft.ML.Data;
public class SensorData
{
public float Temperature { get; set; }
public float Vibration { get; set; }
public float Pressure { get; set; }
public bool IsFailure { get; set; }
}
public class ModelInput
{
[LoadColumn(0)]
public float Temperature { get; set; }
[LoadColumn(1)]
public float Vibration { get; set; }
[LoadColumn(2)]
public float Pressure { get; set; }
[LoadColumn(3)]
public bool IsFailure { get; set; }
}
public class DataLoader
{
public static IDataView LoadData(string dataPath, MLContext mlContext)
{
return mlContext.Data.LoadFromTextFile<ModelInput>(dataPath, separatorChar: ',', hasHeader: true);
}
}
In this code, Emmanuel used ML.NET to load and preprocess the sensor data. He defined a SensorData
class to represent the structure of his data and used the LoadFromTextFile
method to load it into an IDataView
object.
Step 3: Building the Predictive Maintenance Model
Emmanuel’s goal was to build a model that could predict equipment failures based on sensor readings. He used a Binary Classification algorithm to achieve this.
Here’s the C# code to build and train the model:
csharppublic class ModelBuilder
{
public static ITransformer BuildModel(MLContext mlContext, IDataView trainData)
{
var pipeline = mlContext.Transforms.Concatenate("Features", new[] { "Temperature", "Vibration", "Pressure" })
.Append(mlContext.BinaryClassification.Trainers.SdcaLogisticRegression(labelColumnName: "IsFailure", maximumNumberOfIterations: 100));
var model = pipeline.Fit(trainData);
return model;
}
}
In this code, Emmanuel created a pipeline that concatenates feature columns into a single Features
column and uses the SdcaLogisticRegression trainer for binary classification. The model was then trained using the historical sensor data.
Step 4: Evaluating the Model
To ensure the model’s accuracy, Emmanuel needed to evaluate its performance. He split his data into training and testing datasets and used the testing data to assess how well the model performed.
Here’s how he did it:
csharppublic class ModelEvaluator
{
public static void EvaluateModel(MLContext mlContext, ITransformer model, IDataView testData)
{
var predictions = model.Transform(testData);
var metrics = mlContext.BinaryClassification.Evaluate(predictions, labelColumnName: "IsFailure");
Console.WriteLine($"Log-loss: {metrics.LogLoss}");
Console.WriteLine($"AUC: {metrics.AreaUnderRocCurve}");
}
}
This code evaluates the model’s performance using metrics like Log-loss and AUC (Area Under the ROC Curve). Emmanuel checked these metrics to ensure his model could accurately predict equipment failures.
Step 5: Deploying the Model
Once satisfied with the model’s performance, Emmanuel deployed it to Azure. He created a web service in Azure Machine Learning to host the model, allowing real-time predictions on new data.
Here’s how Emmanuel deployed the model:
Create a Web Service: In the Azure Machine Learning workspace, Emmanuel created a new Inference Pipeline and deployed the trained model as a web service.
Create an Azure Function: He wrote an Azure Function to call the web service and obtain predictions. This function was integrated into his factory’s monitoring system.
Integrate with Factory Systems: Emmanuel’s factory sensors sent real-time data to the Azure Function, which then used the deployed model to predict potential failures.
Real-World Impact
Emmanuel’s implementation of predictive maintenance significantly reduced unexpected breakdowns in his factory. The system alerted him to potential failures before they occurred, allowing for timely maintenance and avoiding costly repairs. As a result, his factory saw an increase in production efficiency and a decrease in maintenance costs.
By leveraging C# and Azure Machine Learning, Emmanuel was able to implement a robust predictive maintenance solution that improved the operational efficiency of his factory.