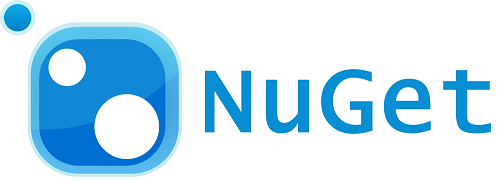
NuGet is the official package manager for .NET. It provides developers with a vast array of libraries, tools, and extensions that enhance productivity, optimize code, and solve real-world problems. As a .NET developer, integrating the right NuGet packages can make your application more efficient, secure, and scalable.
In this article, we'll explore the top 20 NuGet packages that you should consider adding to your .NET application. We’ll provide examples and step-by-step solutions to demonstrate how these packages can improve your development process.
1. Newtonsoft.Json / System.Text.Json
- Purpose: JSON serialization and deserialization
- Use Case: When working with APIs or managing data in JSON format, you need a reliable way to serialize and deserialize JSON.
Installation:
bash Install-Package Newtonsoft.Json
Example:
csharp using Newtonsoft.Json; var jsonString = JsonConvert.SerializeObject(new { Name = "John", Age = 30 }); var person = JsonConvert.DeserializeObject<Person>(jsonString);
2. Serilog
- Purpose: Structured logging for .NET applications
- Use Case: Every application needs a robust logging mechanism. Serilog provides highly customizable and structured logs.
Installation:
bash Install-Package Serilog
Example:
csharp Log.Logger = new LoggerConfiguration() .WriteTo.Console() .CreateLogger(); Log.Information("Application started.");
3. Entity Framework Core
- Purpose: Object-relational mapper (ORM) for .NET
- Use Case: Managing databases in your .NET application is easier with EF Core, which allows seamless interaction between your models and the database.
Installation:
bash Install-Package Microsoft.EntityFrameworkCore
Example:
csharp public class AppDbContext : DbContext { public DbSet<User> Users { get; set; } }
4. AutoMapper
- Purpose: Object mapping
- Use Case: Automatically map properties between objects, simplifying data transformation between layers in your application.
Installation:
bash Install-Package AutoMapper
Example:
csharp var config = new MapperConfiguration(cfg => cfg.CreateMap<User, UserDto>()); var mapper = config.CreateMapper(); UserDto userDto = mapper.Map<UserDto>(user);
5. Polly
- Purpose: Resilience and transient-fault-handling
- Use Case: Polly allows you to implement retry, circuit-breaker, timeout, and other fault-handling policies.
Installation:
bash Install-Package Polly
Example:
csharp var retryPolicy = Policy.Handle<HttpRequestException>().Retry(3); retryPolicy.Execute(() => CallApi());
6. FluentValidation
- Purpose: Validation for .NET models
- Use Case: FluentValidation simplifies the validation process for models, enabling clear and reusable validation rules.
Installation:
bash Install-Package FluentValidation
Example:
csharp public class UserValidator : AbstractValidator<User> { public UserValidator() { RuleFor(user => user.Name).NotEmpty(); } }
7. Swashbuckle.AspNetCore
- Purpose: API documentation using Swagger
- Use Case: Automatically generate API documentation for your ASP.NET Core applications with Swagger.
Installation:
bash Install-Package Swashbuckle.AspNetCore
Example:
csharp services.AddSwaggerGen(); app.UseSwagger();
8. MediatR
- Purpose: Implements the Mediator pattern
- Use Case: MediatR helps decouple components by implementing the mediator pattern. It's ideal for complex business logic.
Installation:
bash Install-Package MediatR
Example:
csharp public class GetUserQuery : IRequest<User> { }
9. NLog
- Purpose: Advanced logging framework
- Use Case: NLog is another popular logging library, with advanced configuration and targeting capabilities.
Installation:
bash Install-Package NLog
Example:
csharp LogManager.GetCurrentClassLogger().Info("Application started.");
10. Dapper
- Purpose: Lightweight ORM
- Use Case: Dapper is a micro ORM that offers fast and simple data access, without the overhead of Entity Framework.
Installation:
bash Install-Package Dapper
Example:
csharp using (var connection = new SqlConnection(connectionString)) { var user = connection.QueryFirst<User>("SELECT * FROM Users WHERE Id = @Id", new { Id = 1 }); }
11. Hangfire
- Purpose: Background job processing
- Use Case: Use Hangfire to run background jobs in your .NET applications, such as sending emails or processing tasks asynchronously.
Installation:
bash Install-Package Hangfire
12. xUnit
- Purpose: Unit testing framework
- Use Case: Write and run unit tests for your application using xUnit.
Installation:
bash Install-Package xUnit
13. Duende IdentityServer
- Purpose: Authentication and authorization server
- Use Case: Use IdentityServer4 to handle OAuth2 and OpenID Connect in your .NET applications.
Installation:
bash Install-Package IdentityServer
14. Refit
- Purpose: Type-safe REST API client
- Use Case: Refit simplifies consuming REST APIs by generating strongly-typed clients.
Installation:
bash Install-Package Refit
15. MiniProfiler
- Purpose: Profiling tool for performance analysis
- Use Case: Monitor the performance of your application with MiniProfiler.
Installation:
bash Install-Package MiniProfiler.AspNetCore.Mvc
16. NSwag
- Purpose: Generate Swagger and C# clients
- Use Case: NSwag generates Swagger UI and .NET client code for ASP.NET Core APIs.
Installation:
bash Install-Package NSwag.AspNetCore
17. Elasticsearch.Net
- Purpose: Interact with Elasticsearch
- Use Case: Use this library to search and index data into Elasticsearch from your .NET applications.
Installation:
bash Install-Package Elasticsearch.Net
18. MassTransit
- Purpose: Distributed application framework
- Use Case: Handle messaging in a distributed system using MassTransit.
Installation:
bash Install-Package MassTransit
19. Quartz.NET
- Purpose: Task scheduling
- Use Case: Use Quartz.NET for scheduling tasks, such as sending out daily reports or running jobs.
Installation:
bash Install-Package Quartz
20. MailKit
- Purpose: Email client library
- Use Case: Send and receive emails from your .NET application using MailKit.
Installation:
bash Install-Package MailKit
Example:
csharp var message = new MimeMessage(); message.From.Add(new MailboxAddress("Name", "you@example.com")); message.To.Add(new MailboxAddress("Recipient", "recipient@example.com")); message.Subject = "Test Email"; using (var client = new SmtpClient()) { client.Connect("smtp.server.com", 587); client.Authenticate("username", "password"); client.Send(message); client.Disconnect(true); }
Conclusion
These top 20 NuGet packages are essential tools for any .NET developer. From JSON serialization with Newtonsoft.Json to logging with Serilog and job scheduling with Quartz.NET, these libraries help solve everyday problems efficiently. By integrating these packages, you can improve the performance, scalability, and maintainability of your .NET applications.
Make sure to explore each package and understand how it can add value to your project, saving you countless hours of development time.