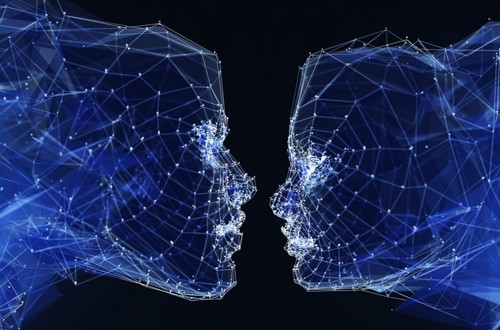
As Sarah, a developer in Lagos, thought about how her startup could revolutionize the way fashion trends are analyzed, she realized she needed a way to automate the classification of thousands of images into categories like "traditional wear," "casual," and "formal." Instead of manually tagging images, Sarah decided to use image classification—the process of teaching a computer to categorize images automatically. To do this, she turned to C# and TensorFlow.
This guide explains how Sarah built an image classification tool using C# and TensorFlow, from setting up the environment to deploying a model that can classify images in real-time.
Step 1: Setting Up the Environment
To begin, Sarah needed to set up her development environment for C# and TensorFlow.NET—a C# binding for TensorFlow. Here’s how she did it:
Install Visual Studio: Sarah opened Visual Studio and created a new C# project.
Install TensorFlow.NET Package: In the NuGet Package Manager, she searched for
TensorFlow.NET
and installed it by running the following command:bashInstall-Package TensorFlow.NET
Prepare Image Data: Sarah prepared a dataset of fashion images that she would classify. For simplicity, she categorized the images into three folders:
Traditional
,Casual
, andFormal
.
Step 2: Loading and Preprocessing the Images
Before training a model, Sarah needed to load and preprocess her images so they could be fed into the neural network. TensorFlow.NET provided her with the tools to do this in C#.
Here’s the code Sarah used to load and preprocess the images:
csharpusing System.Drawing;
using Tensorflow;
using static Tensorflow.Binding;
public class ImagePreprocessor
{
public static Tensor PreprocessImage(string imagePath)
{
Bitmap bitmap = new Bitmap(imagePath);
var resizedImage = new Bitmap(bitmap, new Size(128, 128)); // Resize the image to 128x128 pixels
var imageArray = new float[128 * 128 * 3]; // 3 for RGB channels
int index = 0;
for (int y = 0; y < resizedImage.Height; y++)
{
for (int x = 0; x < resizedImage.Width; x++)
{
var pixel = resizedImage.GetPixel(x, y);
imageArray[index++] = pixel.R / 255f; // Normalize R channel
imageArray[index++] = pixel.G / 255f; // Normalize G channel
imageArray[index++] = pixel.B / 255f; // Normalize B channel
}
}
return tf.constant(imageArray, shape: new long[] { 128, 128, 3 });
}
}
In this code, Sarah used System.Drawing to load and resize the images to a uniform size of 128x128 pixels, and she normalized the RGB values between 0 and 1 to improve model accuracy.
Step 3: Building the Image Classification Model
Next, Sarah needed to build a Convolutional Neural Network (CNN), which is a type of neural network that works well for image classification. TensorFlow.NET allowed her to define and train this model using familiar C# syntax.
Here’s how she set up her model:
csharppublic class ImageClassifier
{
public static Sequential BuildModel()
{
var model = keras.Sequential();
model.add(keras.layers.Conv2D(32, kernel_size: (3, 3), activation: "relu", input_shape: (128, 128, 3)));
model.add(keras.layers.MaxPooling2D(pool_size: (2, 2)));
model.add(keras.layers.Conv2D(64, (3, 3), activation: "relu"));
model.add(keras.layers.MaxPooling2D(pool_size: (2, 2)));
model.add(keras.layers.Conv2D(128, (3, 3), activation: "relu"));
model.add(keras.layers.Flatten());
model.add(keras.layers.Dense(128, activation: "relu"));
model.add(keras.layers.Dense(3, activation: "softmax")); // 3 output classes
model.compile(optimizer: keras.optimizers.Adam(), loss: keras.losses.SparseCategoricalCrossentropy(), metrics: new[] { "accuracy" });
return model;
}
}
This code defines a CNN with three convolutional layers, which are followed by max-pooling layers to reduce spatial dimensions. The final dense layers classify the image into one of three categories: Traditional
, Casual
, or Formal
.
Step 4: Training the Model
After building the model, Sarah had to train it on her image dataset. She split the data into training and validation sets to monitor the model’s performance.
Here’s the code to train the model:
csharppublic class ModelTrainer
{
public static void TrainModel(Sequential model, Tensor trainImages, Tensor trainLabels, Tensor valImages, Tensor valLabels)
{
model.fit(trainImages, trainLabels, batch_size: 32, epochs: 10, validation_data: (valImages, valLabels));
}
}
Sarah loaded the training images and their corresponding labels into the trainImages
and trainLabels
tensors. She trained the model for 10 epochs with a batch size of 32. The validation data helped her monitor how well the model was learning.
Step 5: Evaluating and Testing the Model
Once the training was complete, Sarah evaluated the model’s accuracy and tested it on unseen images to check if it could correctly classify new data.
csharppublic class ModelEvaluator
{
public static void EvaluateModel(Sequential model, Tensor testImages, Tensor testLabels)
{
var evalResult = model.evaluate(testImages, testLabels);
Console.WriteLine($"Test loss: {evalResult[0]}");
Console.WriteLine($"Test accuracy: {evalResult[1]}");
}
}
This code prints out the model’s accuracy and loss on the test data, giving Sarah a sense of how well the model performs on unseen images.
Step 6: Real-World Application
After training the model, Sarah deployed it to a web application where users could upload their fashion images to receive automated category predictions. The tool proved invaluable for fashion retailers, allowing them to analyze market trends and better manage their product inventory.
Conclusion
By using C# and TensorFlow.NET, Sarah was able to build an image classification model that categorizes images into distinct fashion styles. From setting up the environment and preprocessing data to training the model and deploying it, this project highlights how powerful machine learning can be when integrated with C#.
Not only did Sarah automate a tedious task, but she also provided a solution that could be easily applied across other industries, such as healthcare for medical image analysis or retail for product categorization.
Did you know? Image classification is widely used in healthcare for diagnosing diseases through medical imaging—demonstrating the vast potential of AI across different fields!