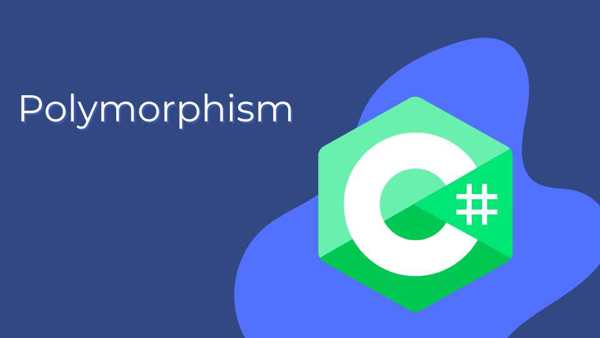
Polymorphism is a core concept in Object-Oriented Programming (OOP) that allows objects of different types to be treated as objects of a common type. Advanced polymorphism takes this concept further by leveraging features like interfaces, abstract classes, generics, delegates, and design patterns to create more flexible, reusable, and maintainable code.
1. Advanced Polymorphism Concepts in C#
a. Interfaces
- What it is: An interface defines a contract that classes must implement. It allows multiple unrelated classes to share common behavior.
- Example in Real Life: A USB port is like an interface. It can connect to a mouse, keyboard, or flash drive, as long as they follow the USB standard.
- Code Example:
interface IDrawable { void Draw(); } class Circle : IDrawable { public void Draw() => Console.WriteLine("Drawing a circle."); } class Square : IDrawable { public void Draw() => Console.WriteLine("Drawing a square."); } IDrawable shape = new Circle(); shape.Draw(); // Drawing a circle. shape = new Square(); shape.Draw(); // Drawing a square.
b. Abstract Classes
- What it is: An abstract class provides a base implementation that derived classes can inherit and extend. It can contain both abstract (must be overridden) and concrete methods.
- Example in Real Life: A vehicle is an abstract concept. A car and a bike are concrete implementations of a vehicle.
- Code Example:
abstract class Vehicle { public abstract void Start(); public void Stop() => Console.WriteLine("Vehicle stopped."); } class Car : Vehicle { public override void Start() => Console.WriteLine("Car started."); } class Bike : Vehicle { public override void Start() => Console.WriteLine("Bike started."); } Vehicle vehicle = new Car(); vehicle.Start(); // Car started. vehicle.Stop(); // Vehicle stopped.
c. Generics with Polymorphism
- What it is: Generics allow you to write code that works with any data type while maintaining type safety.
- Example in Real Life: A toolbox that can hold any type of tool (hammer, screwdriver, wrench) but ensures you only take out the tool you need.
- Code Example:
class Toolbox<T> { public T Tool { get; set; } } Toolbox<Hammer> hammerBox = new Toolbox<Hammer>(); hammerBox.Tool = new Hammer();
d. Delegates and Events
- What it is: Delegates are type-safe function pointers that enable runtime polymorphism by allowing methods to be passed as parameters.
- Example in Real Life: A remote control button that can be programmed to perform different actions (e.g., turn on the TV, dim the lights).
- Code Example:
delegate void ButtonAction(); class RemoteControl { public ButtonAction Action { get; set; } public void PressButton() => Action?.Invoke(); } RemoteControl remote = new RemoteControl(); remote.Action = () => Console.WriteLine("TV turned on."); remote.PressButton(); // TV turned on.
e. Design Patterns (e.g., Strategy Pattern)
- What it is: Design patterns like the Strategy Pattern use polymorphism to define a family of algorithms and make them interchangeable.
- Example in Real Life: A navigation app that can switch between different route strategies (fastest, shortest, scenic).
- Code Example:
interface IRouteStrategy { void CalculateRoute(); } class FastestRoute : IRouteStrategy { public void CalculateRoute() => Console.WriteLine("Calculating fastest route."); } class ScenicRoute : IRouteStrategy { public void CalculateRoute() => Console.WriteLine("Calculating scenic route."); } class Navigator { private IRouteStrategy _strategy; public void SetStrategy(IRouteStrategy strategy) => _strategy = strategy; public void CalculateRoute() => _strategy?.CalculateRoute(); } Navigator navigator = new Navigator(); navigator.SetStrategy(new FastestRoute()); navigator.CalculateRoute(); // Calculating fastest route.
2. Best Practices and Features
Best Practices
- Favor Composition Over Inheritance: Use interfaces and composition to achieve polymorphism instead of deep inheritance hierarchies.
- Use SOLID Principles: Follow principles like the Open/Closed Principle (open for extension, closed for modification) and Liskov Substitution Principle.
- Avoid Tight Coupling: Use dependency injection to decouple classes and make them more testable.
- Use Generics for Type Safety: Leverage generics to write reusable and type-safe code.
- Keep It Simple: Avoid over-engineering. Use polymorphism only when it adds value.
Features
- Flexibility: Easily extend functionality without modifying existing code.
- Reusability: Share common behavior across multiple classes.
- Maintainability: Changes in one part of the code don’t affect other parts.
- Testability: Polymorphic code is easier to test with mocking and dependency injection.
3. Pros and Cons
Pros
- Extensibility: New types can be added without changing existing code.
- Code Reusability: Reduces redundancy by sharing common behavior.
- Dynamic Behavior: Runtime polymorphism allows for flexible and dynamic behavior.
- Decoupling: Reduces dependencies between classes.
Cons
- Complexity: Can make the code harder to understand for beginners.
- Performance Overhead: Runtime polymorphism (e.g., method overriding) can introduce slight performance overhead.
- Overuse: Excessive use of polymorphism can lead to overly complex designs.
4. Alternatives
- Conditional Logic: Use
if-else
orswitch
statements to handle different types. However, this can lead to messy and hard-to-maintain code. - Pattern Matching: In modern C#, use pattern matching to handle different types in a clean way.
switch (shape) { case Circle c: c.Draw(); break; case Square s: s.Draw(); break; default: Console.WriteLine("Unknown shape."); break; }
5. When to Use Advanced Polymorphism
- When You Have Multiple Related Types: Use polymorphism to handle objects of different types in a unified way.
- When You Need Extensibility: Use it when you expect to add new types in the future.
- When You Want to Reduce Code Duplication: Use it to share common behavior across classes.
- When You Need Dynamic Behavior: Use runtime polymorphism to decide behavior at runtime.
- When Working with Frameworks: Frameworks like ASP.NET Core heavily rely on polymorphism for dependency injection and middleware.
Advanced Code Example
using System; // Interface interface IShape { void Draw(); } // Concrete implementations class Circle : IShape { public void Draw() => Console.WriteLine("Drawing a circle."); } class Square : IShape { public void Draw() => Console.WriteLine("Drawing a square."); } // Generic class class ShapeDrawer<T> where T : IShape, new() { public void DrawShape() { T shape = new T(); shape.Draw(); } } // Strategy Pattern interface IDrawStrategy { void Draw(); } class FastDraw : IDrawStrategy { public void Draw() => Console.WriteLine("Drawing quickly."); } class DetailedDraw : IDrawStrategy { public void Draw() => Console.WriteLine("Drawing with details."); } class DrawingContext { private IDrawStrategy _strategy; public void SetStrategy(IDrawStrategy strategy) => _strategy = strategy; public void Draw() => _strategy?.Draw(); } class Program { static void Main() { // Using generics with polymorphism ShapeDrawer<Circle> circleDrawer = new ShapeDrawer<Circle>(); circleDrawer.DrawShape(); // Drawing a circle. // Using strategy pattern DrawingContext context = new DrawingContext(); context.SetStrategy(new FastDraw()); context.Draw(); // Drawing quickly. context.SetStrategy(new DetailedDraw()); context.Draw(); // Drawing with details. } }
Explanation of Advanced Code
- Interfaces:
IShape
defines a contract for drawing shapes. - Generics:
ShapeDrawer<T>
is a generic class that works with any type implementingIShape
. - Strategy Pattern:
DrawingContext
uses the Strategy Pattern to switch between different drawing strategies at runtime.
Advanced polymorphism in C# enables you to write flexible, reusable, and maintainable code. By mastering these concepts, you can build robust applications that are easy to extend and adapt to changing requirements.