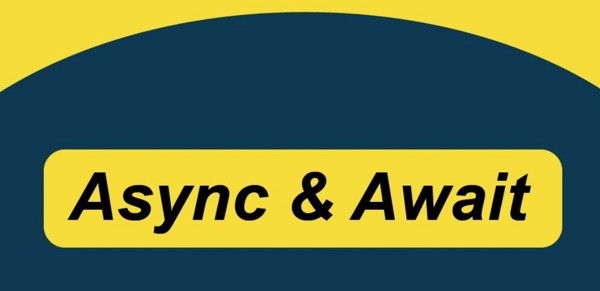
Asynchronous programming in C# has been greatly simplified with the introduction of the async
and await
keywords. By allowing asynchronous operations to be run without blocking threads, we can achieve efficient, responsive applications. However, there are common pitfalls and best practices to ensure that your code runs smoothly and efficiently.
1. Always Use async
and await
for Asynchronous Work, Not Threads
Threads and Task.Run
can be useful, but async/await
is more efficient for I/O-bound operations such as reading from a database or an API.
Example:
csharp // Avoid using Task.Run for I/O-bound operations public void FetchDataWithTaskRun() { Task.Run(() => FetchDataFromApi()); } // Correct: Use async/await public async Task FetchDataWithAsyncAwait() { var data = await FetchDataFromApiAsync(); Console.WriteLine(data); } private async Task<string> FetchDataFromApiAsync() { await Task.Delay(1000); // Simulate async I/O operation return "Data fetched from API"; }
In a real-world case, such as fetching data from a web API in an ASP.NET Core application, using async/await
ensures that the server doesn’t block threads waiting for the I/O operation to complete.
2. Use try/catch
for Error Handling in Async Methods
In async
methods, exceptions are wrapped in a Task
. You can catch these exceptions using try/catch
.
Example:
csharp public async Task<string> FetchDataSafeAsync(string url) { try { // Simulate an asynchronous operation var data = await FetchDataFromApiAsync(url); return data; } catch (Exception ex) { // Handle the error gracefully Console.WriteLine($"Error fetching data: {ex.Message}"); return null; } } private async Task<string> FetchDataFromApiAsync(string url) { await Task.Delay(500); // Simulating delay throw new Exception("API Error"); }
In real life, when building an e-commerce app fetching product details from external APIs, properly handling errors will prevent app crashes and improve user experience by displaying appropriate error messages.
3. Avoid Using await
in Loops
await
inside a loop is a common mistake that causes tasks to run sequentially, significantly slowing down the program. Instead, use Task.WhenAll()
to execute tasks concurrently.
Example:
csharp // Incorrect: Awaiting inside a loop public async Task ProcessItemsSequentiallyAsync(List<string> items) { foreach (var item in items) { await ProcessItemAsync(item); // Runs one by one } } // Correct: Run all tasks concurrently public async Task ProcessItemsConcurrentlyAsync(List<string> items) { var tasks = items.Select(item => ProcessItemAsync(item)); await Task.WhenAll(tasks); // Run tasks in parallel } private async Task ProcessItemAsync(string item) { await Task.Delay(500); // Simulate asynchronous work Console.WriteLine($"Processed {item}"); }
In real-world cases, say you're processing multiple files in a document management system, processing them sequentially would slow down the application. Running the tasks concurrently improves performance.
4. Don’t Use async void
Except for Event Handlers
Using async void
should be avoided as it doesn’t allow you to handle exceptions properly. The only acceptable use of async void
is for event handlers where no return value is expected.
Example:
csharp // Correct usage of async void for event handlers private async void Button_Click(object sender, EventArgs e) { await SaveDataAsync(); } // Incorrect: Avoid using async void elsewhere public async void SaveDataAsync() { await Task.Delay(1000); // Simulate saving data }
In a real-life application, such as a Windows Forms or WPF app, async void
is acceptable for event handlers like button clicks. However, elsewhere in your code, use Task
as the return type.
5. Avoid Blocking the Main Thread with Result
or Wait()
Calling .Result
or .Wait()
on a Task
blocks the main thread and negates the benefits of asynchronous programming. Always use await
instead.
Example:
csharp // Incorrect: Blocking with .Result public string FetchDataBlocking() { return FetchDataFromApiAsync().Result; // This blocks the thread } // Correct: Use await public async Task<string> FetchDataAsync() { var data = await FetchDataFromApiAsync(); return data; }
Blocking the thread in a web application will lead to thread starvation, impacting scalability and performance. Always prefer await
to avoid such issues.
6. Use ConfigureAwait(false)
for Library Code
When writing library code or services that are not dependent on UI synchronization contexts, use ConfigureAwait(false)
to avoid capturing the context and improve performance.
Example:
csharp public async Task<string> FetchDataWithConfigureAwaitAsync() { var data = await FetchDataFromApiAsync().ConfigureAwait(false); return data; }
In real-world applications, like microservices, this ensures that unnecessary synchronization context switching is avoided, which leads to better performance, especially in high-concurrency scenarios.
7. Return Task
Instead of void
for Asynchronous Methods
Methods that perform asynchronous work should always return Task
(or Task<T>
for methods that return a value). This makes error handling easier and ensures the task is properly awaited.
Example:
csharp // Incorrect: Returning void public async void SendEmailAsync() { await Task.Delay(1000); // Simulate sending an email } // Correct: Returning Task public async Task SendEmailTaskAsync() { await Task.Delay(1000); // Simulate sending an email }
In real-world applications, such as sending emails or processing payments, returning a Task
ensures that the operation is tracked and handled appropriately.
8. Leverage Task.WhenAny()
for Timeout Operations
If you want to implement a timeout for a long-running task, Task.WhenAny()
allows you to specify a timeout duration and handle the result accordingly.
Example:
csharp public async Task<string> FetchDataWithTimeoutAsync() { var timeoutTask = Task.Delay(5000); // 5-second timeout var fetchTask = FetchDataFromApiAsync(); var completedTask = await Task.WhenAny(fetchTask, timeoutTask); if (completedTask == timeoutTask) { return "Request timed out"; } return await fetchTask; }
In real-world scenarios, such as querying an external API with potential network issues, timeouts ensure that your application doesn’t hang indefinitely.