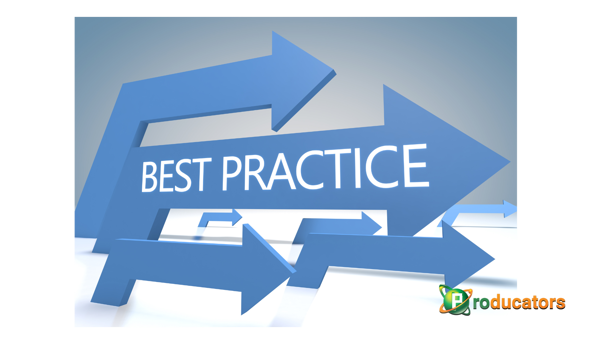
Recursion is a programming concept where a function calls itself to solve a problem. Think of it like a Russian nesting doll: you open one doll, and inside it, there's a smaller doll, and inside that, an even smaller one, and so on. Each doll is similar to the previous one but smaller, just like how a recursive function solves a smaller version of the same problem.
Real-Life Example:
Imagine you're standing in a long line of people, and you want to know how many people are in front of you. You could ask the person in front of you, "How many people are in front of you?" That person would ask the same question to the person in front of them, and so on, until the question reaches the first person in the line, who would say, "There's no one in front of me." Then, each person would add 1 to the number they received and pass it back. Eventually, you'd get the total number of people in front of you.
This is recursion: breaking down a problem into smaller, similar problems until you reach a simple case that can be solved directly.
Advanced Code Example
Let's take a classic example: calculating the factorial of a number. The factorial of a number n
(written as n!
) is the product of all positive integers less than or equal to n
. For example, 5! = 5 * 4 * 3 * 2 * 1 = 120
.
Here’s how you can write this recursively in Python:
python
Copy
def factorial(n): # Base case: if n is 0 or 1, return 1 if n == 0 or n == 1: return 1 # Recursive case: n! = n * (n-1)! else: return n * factorial(n - 1) # Example usage print(factorial(5)) # Output: 120
How it works:
- The function
factorial(n)
calls itself with a smaller value (n-1
). - This continues until it reaches the base case (
n == 0
orn == 1
), where it stops calling itself and starts returning values. - The results are then multiplied together to get the final answer.
Best Practices and Features of Recursion
- Base Case: Always define a base case to stop the recursion. Without it, the function will call itself infinitely, leading to a stack overflow.
- Simplify the Problem: Ensure that each recursive call works on a smaller or simpler version of the problem.
- Avoid Redundant Calculations: Use techniques like memoization (caching results) to avoid recalculating the same values repeatedly.
- Readability: Recursion can make code more readable and elegant for problems that are naturally recursive (e.g., tree traversals).
- Tail Recursion: Some languages optimize tail-recursive functions to avoid stack overflow. In tail recursion, the recursive call is the last operation in the function.
Pros and Cons of Recursion
Pros:
- Elegance: Recursive solutions are often simpler and more intuitive for problems that have a recursive structure (e.g., tree traversal, Fibonacci sequence).
- Divide and Conquer: Recursion naturally fits divide-and-conquer algorithms like merge sort and quicksort.
- Less Code: Recursive solutions can sometimes require fewer lines of code compared to iterative solutions.
Cons:
- Stack Overflow: Each recursive call consumes memory on the call stack. If the recursion is too deep, it can lead to a stack overflow.
- Performance Overhead: Recursive calls can be slower due to the overhead of function calls and maintaining the call stack.
- Debugging Difficulty: Recursive code can be harder to debug because of the multiple layers of function calls.
Alternatives to Recursion
- Iteration: Use loops (e.g.,
for
,while
) to solve the problem iteratively. For example, the factorial function can be written iteratively:
def factorial(n): result = 1 for i in range(1, n + 1): result *= i return result
- Memoization: Store the results of expensive function calls and reuse them when the same inputs occur again.
- Dynamic Programming: Break the problem into smaller subproblems, solve each subproblem once, and store the results to avoid redundant calculations.
When to Use Recursion
Use recursion when:
- The problem can be naturally divided into smaller, similar subproblems (e.g., tree traversal, Fibonacci sequence).
- The problem requires backtracking (e.g., solving a maze or generating permutations).
- The code readability and simplicity are more important than performance (e.g., small datasets or problems with limited depth).
Avoid recursion when:
- The recursion depth is very large, leading to stack overflow.
- Performance is critical, and an iterative solution is more efficient.
- The problem can be solved easily with loops or other techniques.
Summary
Recursion is a powerful tool for solving problems that can be broken down into smaller, similar subproblems. It’s elegant and intuitive for certain tasks but comes with trade-offs like potential stack overflow and performance overhead. Use recursion when it simplifies the problem, but consider alternatives like iteration or dynamic programming for performance-critical applications