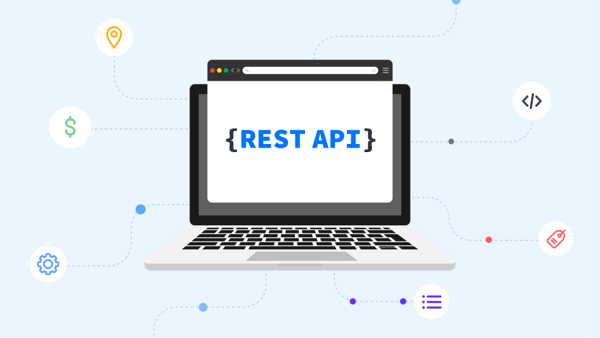
In today’s interconnected world, RESTful APIs have become the backbone of modern software architecture. REST (Representational State Transfer) provides a way for different systems to communicate over HTTP, making it an ideal choice for building scalable web services. .NET is a powerful framework from Microsoft that makes developing RESTful APIs not only straightforward but highly efficient.
If you're a developer looking to create high-performance, scalable APIs in .NET, this article will guide you through best practices and examples that demonstrate how to design and implement robust RESTful APIs. Let’s dive into the key features, real-life examples, and step-by-step solutions that will help you succeed.
Best Practices in Designing RESTful APIs in .NET
1. Use Proper HTTP Methods
One of the core principles of REST is the proper use of HTTP methods (verbs). Here's a breakdown of the most commonly used HTTP methods and how to implement them:
- GET: Retrieve data from the server.
- POST: Submit data to the server (often used for creating resources).
- PUT: Update an existing resource.
- DELETE: Remove a resource.
Example in .NET:
csharp [HttpGet] public IActionResult GetProducts() { var products = _productService.GetAll(); return Ok(products); } [HttpPost] public IActionResult CreateProduct([FromBody] ProductDto productDto) { var createdProduct = _productService.Add(productDto); return CreatedAtRoute("GetProductById", new { id = createdProduct.Id }, createdProduct); } [HttpPut("{id}")] public IActionResult UpdateProduct(int id, [FromBody] ProductDto productDto) { _productService.Update(id, productDto); return NoContent(); } [HttpDelete("{id}")] public IActionResult DeleteProduct(int id) { _productService.Delete(id); return NoContent(); }
Real-life analogy: Imagine a library system. You might send a GET request to retrieve the list of books, a POST request to add a new book, a PUT request to update an existing book’s details, and a DELETE request to remove a book from the catalog.
2. Use Meaningful Resource Names
When designing your API, ensure that the resource names are nouns and not verbs, representing the entities being manipulated. Also, make sure the names are meaningful and reflect the action performed on the resource.
Good practice:
/products
(Plural noun for collections)/products/123
(Singular noun for a specific resource)
Bad practice:
/getAllProducts
/deleteProduct
In a real-world scenario, think of a customer service ticketing system. An endpoint named /tickets/
makes more sense than /fetchAllTickets
, as the former represents a collection of resources, and the latter reflects an unnecessary action.
3. Versioning Your API
API versioning ensures that your clients don’t break when you introduce new changes. Here are some common versioning strategies:
- URL Versioning:
/v1/products
- Query String Versioning:
/products?version=1
- Header Versioning: Using custom headers like
X-API-Version
.
Example in .NET:
csharp [ApiVersion("1.0")] [Route("api/v{version:apiVersion}/products")] [ApiController] public class ProductsV1Controller : ControllerBase { // API logic here } [ApiVersion("2.0")] [Route("api/v{version:apiVersion}/products")] [ApiController] public class ProductsV2Controller : ControllerBase { // Version 2 API logic here }
In a real-life business scenario, imagine your software is running multiple versions of APIs to support older mobile apps while still delivering new features for updated ones.
4. Use Status Codes Wisely
HTTP status codes communicate the result of an API request. Use them appropriately to provide meaningful feedback to clients:
- 200 OK: The request was successful.
- 201 Created: The resource was created.
- 204 No Content: The request was successful, but no content to return.
- 400 Bad Request: The request was malformed or invalid.
- 401 Unauthorized: Authentication failed.
- 404 Not Found: The requested resource doesn’t exist.
- 500 Internal Server Error: Something went wrong on the server.
Example:
csharp public IActionResult GetProduct(int id) { var product = _productService.GetById(id); if (product == null) { return NotFound(); // 404 } return Ok(product); // 200 }
In a real-life analogy, status codes are like the response you get when making a request in a store. "404 Not Found" is akin to a store clerk telling you that the product you're looking for isn’t in stock.
5. Paginate Large Responses
For endpoints that return large sets of data, implementing pagination is essential to prevent overloading both the client and the server.
Example in .NET:
csharp [HttpGet] public IActionResult GetProducts([FromQuery] int pageNumber = 1, [FromQuery] int pageSize = 10) { var products = _productService.GetPagedProducts(pageNumber, pageSize); return Ok(products); }
Imagine an e-commerce platform where you query for products. Instead of returning thousands of results at once, pagination allows you to get 10 or 20 items per page.
6. Use DTOs and Avoid Exposing Internal Models
Data Transfer Objects (DTOs) allow you to control what data is exposed via the API. Exposing your internal domain models could result in unwanted data exposure or tight coupling between the API and the database.
Example in .NET:
csharp public class ProductDto { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } }
This ensures that sensitive data, such as internal IDs or timestamps, are not exposed to API consumers.
7. Secure Your API
Security is paramount when designing any API. Consider the following:
- Authentication: Use OAuth 2.0 or JWT (JSON Web Tokens) for securing your API.
- HTTPS Only: Ensure that all API communication happens over HTTPS.
- Rate Limiting: Prevent abuse by limiting the number of requests a client can make in a certain period.
Example in .NET:
csharp services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme) .AddJwtBearer(options => { options.TokenValidationParameters = new TokenValidationParameters { ValidateIssuer = true, ValidateAudience = true, ValidateLifetime = true, ValidateIssuerSigningKey = true, ValidIssuer = Configuration["Jwt:Issuer"], ValidAudience = Configuration["Jwt:Audience"], IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:Key"])) }; });
In real-life terms, this is akin to requiring a customer to show their ID before allowing them into a secure building.
8. Implement Caching for Better Performance
Reduce server load and improve response times by caching frequently requested data. Tools like Redis or in-memory caching can help.
Example in .NET:
csharp [HttpGet] [ResponseCache(Duration = 60)] public IActionResult GetProducts() { var products = _productService.GetAll(); return Ok(products); }
This is like a restaurant keeping popular items prepped in advance, so customers don’t wait as long for their meals.
9. Handle Errors Gracefully
Proper error handling ensures that your API provides meaningful and helpful responses when things go wrong. Avoid returning stack traces or internal exceptions in production, as they can expose vulnerabilities. Instead, use standardized error messages and codes.
Example in .NET:
csharp public IActionResult GetProduct(int id) { try { var product = _productService.GetById(id); if (product == null) { return NotFound(new { message = "Product not found" }); // 404 } return Ok(product); // 200 } catch (Exception ex) { return StatusCode(500, new { message = "An error occurred", details = ex.Message }); // 500 } }
In real life, this is similar to getting a clear message from customer support when a service is unavailable, rather than confusing technical details.
10. Use Asynchronous Programming
To improve scalability and performance, especially when dealing with I/O-bound tasks like database queries or external service calls, it's best to use asynchronous methods in your API. .NET offers great support for async/await, which prevents thread blocking and enhances performance.
Example in .NET:
csharp [HttpGet("{id}")] public async Task<IActionResult> GetProductAsync(int id) { var product = await _productService.GetByIdAsync(id); if (product == null) { return NotFound(); } return Ok(product); }
Asynchronous programming is like delegating tasks to others so you can focus on other jobs in the meantime, making the process more efficient.
11. Implement Logging and Monitoring
Logging and monitoring are essential for tracking the health and performance of your API. Use structured logging and integrate tools like Serilog, ELK stack, or Azure Monitor to capture relevant information about API requests, errors, and performance metrics.
Example in .NET:
csharp public class ProductsController : ControllerBase { private readonly ILogger<ProductsController> _logger; public ProductsController(ILogger<ProductsController> logger) { _logger = logger; } [HttpGet("{id}")] public IActionResult GetProduct(int id) { _logger.LogInformation("Fetching product with ID: {ProductId}", id); var product = _productService.GetById(id); if (product == null) { _logger.LogWarning("Product with ID: {ProductId} not found", id); return NotFound(); } return Ok(product); } }
In a real-world scenario, this is akin to having security cameras in a store, so you can track activity and quickly respond to issues.
12. Enforce Data Validation
Before processing input data from users, ensure it is properly validated to prevent malformed or harmful requests from entering the system. Use model validation attributes and custom validation logic when necessary.
Example in .NET:
csharp public class ProductDto { [Required] [StringLength(100, MinimumLength = 3)] public string Name { get; set; } [Range(1, 10000)] public decimal Price { get; set; } } [HttpPost] public IActionResult CreateProduct([FromBody] ProductDto productDto) { if (!ModelState.IsValid) { return BadRequest(ModelState); // 400 Bad Request } var createdProduct = _productService.Add(productDto); return CreatedAtRoute("GetProductById", new { id = createdProduct.Id }, createdProduct); }
Data validation is like requiring proof of identity before issuing a credit card—ensuring only valid data is processed.
13. Use HATEOAS (Hypermedia as the Engine of Application State)
HATEOAS is an extension of REST that provides clients with navigation links in the responses to help them discover other actions they can take. It makes APIs more flexible and self-descriptive.
Example in .NET:
csharp public class ProductResource { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } public List<Link> Links { get; set; } } public class Link { public string Href { get; set; } public string Rel { get; set; } public string Method { get; set; } } public IActionResult GetProductWithLinks(int id) { var product = _productService.GetById(id); if (product == null) { return NotFound(); } var resource = new ProductResource { Id = product.Id, Name = product.Name, Price = product.Price, Links = new List<Link> { new Link { Href = Url.Link("GetProductById", new { id = product.Id }), Rel = "self", Method = "GET" }, new Link { Href = Url.Link("DeleteProduct", new { id = product.Id }), Rel = "delete", Method = "DELETE" } } }; return Ok(resource); }
This is similar to an e-commerce website suggesting related products or actions you can take, like adding a product to your wishlist or proceeding to checkout.
14. Document Your API
Proper documentation is critical for API consumers. Tools like Swagger (via Swashbuckle in .NET) can help auto-generate interactive API documentation.
Example in .NET:
csharp services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new OpenApiInfo { Title = "Product API", Version = "v1" }); }); app.UseSwagger(); app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "Product API V1"); });
Documentation is like providing users with a user manual for a product, helping them understand how to use it effectively.
15. Test Your API Thoroughly
Testing ensures that your API functions as expected. Unit tests verify individual components, while integration tests ensure that your API works well with other systems. Tools like XUnit, NUnit, and Moq in .NET can help you write comprehensive test suites.
Example in .NET:
csharp public class ProductServiceTests { private readonly ProductService _productService; private readonly Mock<IProductRepository> _mockRepo; public ProductServiceTests() { _mockRepo = new Mock<IProductRepository>(); _productService = new ProductService(_mockRepo.Object); } [Fact] public void GetProductById_ProductExists_ReturnsProduct() { // Arrange var product = new Product { Id = 1, Name = "Test Product" }; _mockRepo.Setup(r => r.GetById(1)).Returns(product); // Act var result = _productService.GetById(1); // Assert Assert.NotNull(result); Assert.Equal("Test Product", result.Name); } }
Testing is like ensuring all components of a machine work smoothly before rolling it out to users.
16. Use Dependency Injection
To make your API more modular and testable, employ Dependency Injection (DI), a core feature in ASP.NET Core. DI allows you to inject dependencies like services or repositories into controllers instead of creating them directly.
Example in .NET:
csharp public class ProductsController : ControllerBase { private readonly IProductService _productService; public ProductsController(IProductService productService) { _productService = productService; } [HttpGet] public IActionResult GetProducts() { var products = _productService.GetAll(); return Ok(products); } }
Dependency injection is like a chef in a restaurant getting the ingredients delivered by a supplier, rather than growing them himself—saving time and ensuring focus.
17. Paginate Large Datasets
When dealing with large amounts of data, it's crucial to implement pagination to avoid overwhelming your API users or overloading your server. Pagination splits data into manageable chunks, which improves performance and usability.
Example in .NET:
csharp [HttpGet] public IActionResult GetProducts([FromQuery] int page = 1, [FromQuery] int pageSize = 10) { var products = _productService.GetAll() .Skip((page - 1) * pageSize) .Take(pageSize) .ToList(); return Ok(products); }
Pagination in APIs is like paging through a book—rather than handing someone the entire book at once, you allow them to flip through it at their own pace.
18. Rate Limiting
Rate limiting protects your API from abuse by limiting the number of requests a client can make within a certain time frame. This can prevent DoS (Denial of Service) attacks and ensure fair usage among users.
Example in .NET:
You can implement rate limiting using AspNetCoreRateLimit library, which allows you to configure limits per client IP or API key.
json "IpRateLimiting": { "EnableEndpointRateLimiting": true, "StackBlockedRequests": false, "RealIpHeader": "X-Real-IP", "ClientIdHeader": "X-ClientId", "GeneralRules": [ { "Endpoint": "*", "Period": "1m", "Limit": 100 }, { "Endpoint": "*", "Period": "1h", "Limit": 1000 } ] }
Rate limiting is akin to controlling the flow of customers into a store, allowing a set number at a time to avoid overcrowding.
19. Secure Sensitive Data
Ensure sensitive information such as passwords, API keys, and personal data is not exposed. Use encryption both at rest and in transit (HTTPS), and implement secure authentication mechanisms like OAuth2 or JWT (JSON Web Tokens).
Example in .NET:
You can configure JWT authentication in ASP.NET Core with the following middleware:
csharp public void ConfigureServices(IServiceCollection services) { var key = Encoding.ASCII.GetBytes(Configuration["Jwt:Key"]); services.AddAuthentication(x => { x.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme; x.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme; }).AddJwtBearer(x => { x.RequireHttpsMetadata = true; x.SaveToken = true; x.TokenValidationParameters = new TokenValidationParameters { ValidateIssuerSigningKey = true, IssuerSigningKey = new SymmetricSecurityKey(key), ValidateIssuer = false, ValidateAudience = false }; }); }
Securing sensitive data is like locking away personal files in a safe, ensuring they can only be accessed by authorized people.
20. Version Your API
As your API evolves, it's important to version it to avoid breaking changes for existing consumers. API versioning allows you to introduce new functionality while maintaining backward compatibility.
Example in .NET:
csharp [ApiVersion("1.0")] [ApiVersion("2.0")] [Route("api/v{version:apiVersion}/products")] public class ProductsController : ControllerBase { [HttpGet] [MapToApiVersion("1.0")] public IActionResult GetV1() { return Ok("This is version 1.0"); } [HttpGet] [MapToApiVersion("2.0")] public IActionResult GetV2() { return Ok("This is version 2.0"); } }
Versioning is like releasing new models of a car while still offering support and spare parts for the older versions.
Conclusion
Designing and implementing a RESTful API in .NET requires following established best practices to ensure scalability, security, and ease of use. By adhering to the principles of REST, using appropriate HTTP methods, providing clear resource names, and maintaining a focus on performance through caching and pagination, you can build reliable APIs that meet the needs of modern applications.