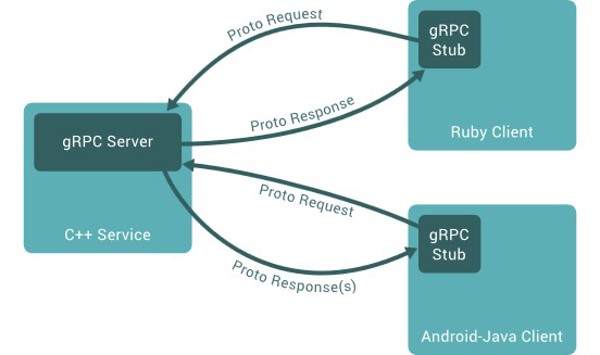
In a world where milliseconds can define user experience, building high-performance, low-latency APIs is more critical than ever. Traditional REST APIs have served the web for years, but gRPC—a modern, open-source Remote Procedure Call (RPC) framework developed by Google—offers a new way to communicate efficiently between services, especially for microservices-based systems and real-time applications.
gRPC provides a more efficient alternative to REST, boasting features like low-latency communications, binary serialization, streaming, and tight integration with HTTP/2. In this article, we’ll explore how to use gRPC services to build a high-performance API, focusing on practical, real-life examples. We’ll also cover how to implement a full CRUD (Create, Read, Update, Delete) operation using gRPC in .NET, showcasing best practices along the way.
Why Use gRPC for High-Performance APIs?
To understand why gRPC is such a good fit for building fast, low-latency APIs, let's compare it to REST:
- Efficiency: REST relies on human-readable text formats like JSON or XML, which are parsed and interpreted at runtime. gRPC uses Protocol Buffers (protobufs), a binary format that’s much more efficient in terms of space and speed.
- Streaming: gRPC offers native streaming support, enabling real-time bidirectional communication between clients and servers, whereas REST is typically limited to single request/response cycles.
- HTTP/2: gRPC operates on HTTP/2, allowing multiplexing, compression, and full-duplex streaming by default, significantly reducing latency compared to REST APIs using HTTP/1.1.
Real-Life Example: Microservices and Real-Time Communication
Let’s say you’re working for a logistics company that tracks thousands of vehicles in real time. Each vehicle sends telemetry data (location, speed, status) to a central API. REST might work fine for a small fleet, but as the number of vehicles grows, latency and overhead from REST’s human-readable data formats can cause performance bottlenecks. Switching to gRPC, you can minimize the overhead and handle real-time streaming of data from all vehicles efficiently, while ensuring low latency for critical updates.
Getting Started with gRPC in .NET
gRPC is well-integrated into .NET and setting it up is straightforward. To get started, let’s go through the steps required to build a gRPC service in a .NET project.
Step 1: Setting Up a gRPC Service
First, you need to create a new gRPC project in .NET:
bash dotnet new grpc -n GrpcServiceDemo
Once the project is created, you’ll find a Protos
folder containing .proto
files. These files define your gRPC service’s contracts. A simple Proto
file might look like this:
protobuf syntax = "proto3"; option csharp_namespace = "GrpcServiceDemo"; service VehicleService { rpc GetVehicle (VehicleRequest) returns (VehicleResponse); rpc CreateVehicle (Vehicle) returns (VehicleResponse); rpc UpdateVehicle (Vehicle) returns (VehicleResponse); rpc DeleteVehicle (VehicleRequest) returns (Empty); } message Vehicle { int32 id = 1; string make = 2; string model = 3; int32 year = 4; } message VehicleRequest { int32 id = 1; } message VehicleResponse { Vehicle vehicle = 1; }
This .proto
file defines a VehicleService
with methods for CRUD operations. Each method accepts and returns proto
-defined messages.
Step 2: Generate gRPC Code
Once your .proto
file is defined, .NET will automatically generate the necessary client and server code from the .proto
file when you build your project.
Make sure to install the necessary NuGet packages:
bash dotnet add package Grpc.AspNetCore dotnet add package Google.Protobuf
Step 3: Implement the gRPC Service
Next, let’s implement the gRPC service. The generated base class for VehicleService
will be in the GrpcServiceDemo
namespace.
csharp public class VehicleService : GrpcServiceDemo.VehicleService.VehicleServiceBase { private static readonly List<Vehicle> Vehicles = new List<Vehicle>(); public override Task<VehicleResponse> GetVehicle(VehicleRequest request, ServerCallContext context) { var vehicle = Vehicles.FirstOrDefault(v => v.Id == request.Id); return Task.FromResult(new VehicleResponse { Vehicle = vehicle }); } public override Task<VehicleResponse> CreateVehicle(Vehicle request, ServerCallContext context) { Vehicles.Add(request); return Task.FromResult(new VehicleResponse { Vehicle = request }); } public override Task<VehicleResponse> UpdateVehicle(Vehicle request, ServerCallContext context) { var vehicle = Vehicles.FirstOrDefault(v => v.Id == request.Id); if (vehicle != null) { vehicle.Make = request.Make; vehicle.Model = request.Model; vehicle.Year = request.Year; } return Task.FromResult(new VehicleResponse { Vehicle = request }); } public override Task<Empty> DeleteVehicle(VehicleRequest request, ServerCallContext context) { var vehicle = Vehicles.FirstOrDefault(v => v.Id == request.Id); if (vehicle != null) { Vehicles.Remove(vehicle); } return Task.FromResult(new Empty()); } }
In this implementation, we’ve created a simple in-memory store for vehicle data and implemented CRUD operations for our gRPC service.
Step 4: Configuring and Running the gRPC Service
Open Startup.cs
or Program.cs
(depending on your .NET version), and make sure to register the gRPC service:
csharp var builder = WebApplication.CreateBuilder(args); var app = builder.Build(); app.MapGrpcService<VehicleService>(); app.MapGet("/", () => "This is a gRPC service!"); app.Run();
This minimal setup will start the gRPC server at the default URL, usually localhost:5001
for HTTPS.
Step 5: Building the gRPC Client
Once your service is running, you can create a client to consume the service. Let’s say you’re building a client to consume the VehicleService
.
csharp var channel = GrpcChannel.ForAddress("https://localhost:5001"); var client = new VehicleService.VehicleServiceClient(channel); // Create a vehicle var response = await client.CreateVehicleAsync(new Vehicle { Id = 1, Make = "Toyota", Model = "Camry", Year = 2022 }); Console.WriteLine($"Created Vehicle: {response.Vehicle.Make} {response.Vehicle.Model}");
This client connects to the gRPC service and sends a request to create a new vehicle. The process is asynchronous, taking advantage of gRPC’s support for non-blocking operations.
Best Practices for Building gRPC Services
1. Use Protobuf Effectively
gRPC’s efficiency comes from its use of Protocol Buffers (protobuf). Make sure to define your protobuf messages carefully, as changes to the .proto
file can break backward compatibility. Use field numbers judiciously and avoid removing them once added.
2. Batch Streaming for Real-Time Data
Leverage gRPC's streaming capabilities for real-time applications. Whether you're receiving telemetry data from devices or sending continuous updates to a dashboard, use server-side streaming for efficient data delivery.
3. Consider Load Balancing
gRPC works well with load balancers, particularly because it uses HTTP/2 multiplexing to reduce connection overhead. If you’re building a distributed microservices architecture, make sure to configure your services to handle load balancing efficiently.
4. Optimize for Low Latency
gRPC is built for low latency, but you can further optimize by using compression, minimizing large payloads, and reducing network hops.
5. Secure Communication
Always ensure that your gRPC services use TLS encryption. gRPC has built-in support for secure connections, and it’s important to secure API traffic between clients and servers.
Conclusion
gRPC offers a powerful way to build high-performance, low-latency APIs, especially when dealing with microservices, real-time data, or communication-heavy applications. By using Protocol Buffers and HTTP/2, gRPC enables faster and more efficient API calls compared to REST. The example we walked through shows how to build a CRUD API using gRPC in .NET, but the potential for this technology extends far beyond simple data operations.