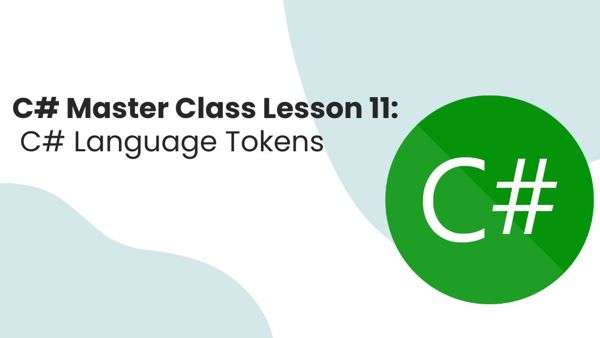
In C#, tokens are the smallest elements of a program that are meaningful to the compiler. They are like the basic building blocks used to construct a C# program. The C# language has several types of tokens, including keywords, identifiers, literals, operators, and separators. Each token has a specific role in the code, helping define its structure and functionality.
1. Keywords
Keywords are reserved words predefined by the C# language and have special meanings. They are used to define the syntax and structure of C# programs. Since these words are reserved, you cannot use them as identifiers (e.g., variable names or method names).
Example:
csharp int age = 30; if (age > 18) { Console.WriteLine("Adult"); }
int
,if
, andConsole.WriteLine
are all examples of keywords.int
defines a data type (integer).if
is used for conditional logic.Console.WriteLine
is a method for outputting to the console.
Some commonly used keywords in C# include:
- Data Types:
int
,float
,bool
,string
,double
- Control Structures:
if
,else
,switch
,for
,while
,do
- Access Modifiers:
public
,private
,protected
,internal
- Class and Method Modifiers:
static
,abstract
,virtual
,override
- Exception Handling:
try
,catch
,finally
,throw
Example of a reserved keyword misuse:
csharp int int = 10; // This will throw an error, as 'int' is a reserved keyword.
2. Identifiers
Identifiers are names given to various elements such as variables, methods, classes, and other objects in the program. Identifiers are user-defined and must follow specific rules:
- Can contain letters, digits, and underscores (
_
). - Must start with a letter or an underscore.
- Cannot contain spaces.
- Cannot be a keyword.
- C# is case-sensitive, so
myVariable
andMyVariable
are different identifiers.
Example:
csharp int age = 25; string firstName = "John"; bool isEmployed = true;
In this example:
age
,firstName
, andisEmployed
are identifiers representing a variable name, and their values are stored in memory.
3. Literals
Literals are constant values assigned to variables. These are fixed values in the program that do not change during execution. Literals can be of different types:
- Integer Literals: Whole numbers like
10
,-5
. - Floating-Point Literals: Decimal numbers like
3.14
,-0.01
. - Boolean Literals:
true
orfalse
. - Character Literals: Single characters enclosed in single quotes like
'A'
,'b'
. - String Literals: A sequence of characters enclosed in double quotes like
"Hello, World!"
.
Example:
csharp int x = 42; // Integer literal float pi = 3.14f; // Floating-point literal char grade = 'A'; // Character literal string message = "Welcome to C#"; // String literal bool isActive = true; // Boolean literal
Here, 42
, 3.14f
, 'A'
, "Welcome to C#"
, and true
are all literals.
4. Operators
Operators are tokens that perform operations on variables and values. C# supports various types of operators:
- Arithmetic Operators: Perform mathematical operations.
+
,-
,*
,/
,%
- Relational (Comparison) Operators: Compare two values.
==
,!=
,>
,<
,>=
,<=
- Logical Operators: Perform logical operations, often used with boolean values.
&&
,||
,!
- Assignment Operators: Assign values to variables.
=
,+=
,-=
,*=
,/=
- Bitwise Operators: Operate on the binary representation of data.
&
,|
,^
,~
,<<
,>>
- Unary Operators: Operate on a single operand.
++
,--
,+
,-
Example:
csharp int a = 10; int b = 20; int sum = a + b; // Arithmetic operator bool isEqual = a == b; // Comparison operator bool condition = (a < b) && (b > 15); // Logical operator a += 5; // Assignment operator
In this example:
+
is an arithmetic operator.==
is a comparison operator.&&
is a logical operator.+=
is an assignment operator.
5. Separators (Delimiters)
Separators (also known as delimiters or punctuation) are tokens that help define the structure of the code by separating statements and blocks. C# uses the following separators:
- Parentheses
()
: Used for grouping expressions or function calls. - Braces
{}
: Define code blocks like loops, methods, or classes. - Square Brackets
[]
: Used for arrays or indexers. - Comma
,
: Separates multiple items in method calls, parameters, or variable declarations. - Semicolon
;
: Marks the end of a statement. - Dot
.
: Used for accessing members of a class or object (e.g., methods, properties).
Example:
csharp int[] numbers = { 1, 2, 3 }; // Square brackets and braces Console.WriteLine(numbers[0]); // Parentheses and dot operator for (int i = 0; i < numbers.Length; i++) // Parentheses, braces, and semicolon { Console.WriteLine(numbers[i]); }
In this example:
{}
is used to define code blocks for the array and thefor
loop.[]
is used for accessing array elements..
is used to accessLength
property of the array andWriteLine()
method ofConsole
.,
separates elements in the array.;
marks the end of a statement.
6. Comments
Although not exactly a token in terms of syntactical meaning, comments are an important part of writing readable and maintainable code. Comments are ignored by the compiler and are used to annotate the code to help other developers (or yourself) understand its purpose.
- Single-line comment: Starts with
//
- Multi-line comment: Enclosed between
/* */
Example:
csharp // This is a single-line comment int value = 100; /* This is a multi-line comment */
Comments do not affect the execution of the code.
7. Preprocessor Directives
Preprocessor directives are special tokens that start with #
and are used to instruct the compiler to preprocess information before actual compilation starts. These tokens help in conditional compilation, error checking, and including/excluding code.
Example:
csharp #define DEBUG using System; class Program { static void Main() { #if DEBUG Console.WriteLine("Debug mode is enabled"); #endif Console.WriteLine("Hello, World!"); } }
In this example:
#define
defines a conditional compilation symbol.#if
checks if theDEBUG
symbol is defined and conditionally includes the code block within it.
Conclusion
In C#, tokens are essential building blocks that create the foundation of the language. They include keywords, which define the structure of the code; identifiers, which name variables and functions; literals, which represent constant values; operators, which perform operations on variables; separators, which define structure and boundaries; comments, which provide annotations; and preprocessor directives, which control the compilation process. Understanding how to use these tokens effectively is key to writing clean, efficient, and functional C# code.