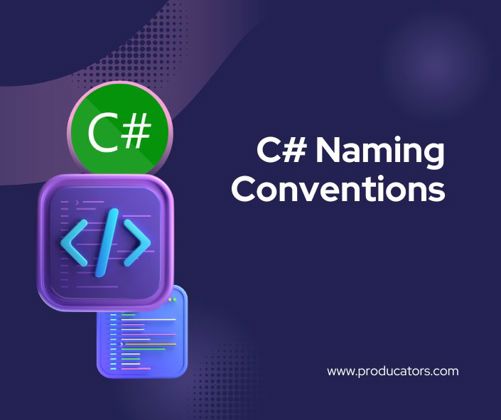
C# naming conventions are guidelines for writing code in a consistent and readable way. Following these conventions makes your code easier to understand and maintain. Here's an overview of common C# naming conventions with examples:
1. Pascal Case (PascalCasing)
Pascal Case starts with an uppercase letter, and the first letter of each subsequent word is also capitalized. This is used for:
- Class Names
- Method Names
- Properties
Example:
csharp // Class Name public class CustomerOrder { // Property public string OrderNumber { get; set; } // Method public void ProcessOrder() { // Code here } }
In the example, CustomerOrder
(class), OrderNumber
(property), and ProcessOrder
(method) all follow Pascal Case.
2. Camel Case (camelCasing)
Camel Case starts with a lowercase letter, and the first letter of each subsequent word is capitalized. This is typically used for:
- Local Variables
- Method Parameters
- Private Fields (with underscore)
Example:
csharp public class CustomerOrder { // Private field (often with an underscore prefix) private string _customerName; // Constructor with parameters public CustomerOrder(string orderNumber, string customerName) { _customerName = customerName; // Local variable: orderNumber } }
In the example, _customerName
(private field) follows camelCase with an underscore prefix, and orderNumber
and customerName
(method parameters) are in camelCase.
3. Constants (ALL_CAPS)
Constants are usually named in all uppercase letters with underscores between words. This helps distinguish constant values from other variables.
Example:
csharp public class MathConstants { // Constant public const double PI = 3.14159; }
In this example, PI
is a constant value written in all uppercase letters.
4. Interfaces (Prefix with 'I')
Interface names in C# typically start with an uppercase letter 'I' followed by a name that follows Pascal Case. This convention helps differentiate interfaces from classes and other types.
Example:
csharp public interface IOrderProcessor { void ProcessOrder(); }
In the example, IOrderProcessor
is an interface name that starts with "I" and follows Pascal Case.
5. Enum (Pascal Case)
Enum names and their members should follow Pascal Case. Enums should be named to represent a set of closely related values.
Example:
csharp public enum OrderStatus { Pending, Shipped, Delivered, Canceled }
Here, OrderStatus
(enum name) and Pending
, Shipped
, Delivered
, Canceled
(enum values) follow Pascal Case.
6. Private Fields (camelCase with Underscore)
Private fields are often written in camelCase and are prefixed with an underscore.
Example:
csharp public class Customer { // Private field private string _customerName; }
In this example, _customerName
is a private field following camelCase and using an underscore as a prefix.
7. Namespace Names (Pascal Case)
Namespaces should use Pascal Case and usually represent the company, project, or functionality. It's common to structure namespaces hierarchically, starting with the company or project name followed by the specific module or functionality.
Example:
csharp namespace CompanyName.ProjectName.ModuleName { public class CustomerOrder { // Code here } }
In this example, CompanyName
, ProjectName
, and ModuleName
all follow Pascal Case.
8. File Names (Pascal Case)
Each C# file should generally contain one class, interface, or enum, and the file name should match the class or interface name using Pascal Case.
Example:
plaintext CustomerOrder.cs IOrderProcessor.cs OrderStatus.cs
If your class is named CustomerOrder
, the corresponding file should be named CustomerOrder.cs
.
9. Event Names (Pascal Case)
Events should also follow Pascal Case and typically use a verb or action that describes the event. It's a common practice to add the "EventHandler" suffix to event handler names.
Example:
csharp public class Order { // Event public event EventHandler OrderProcessed; // Method to trigger event protected virtual void OnOrderProcessed() { OrderProcessed?.Invoke(this, EventArgs.Empty); } }
In this example, OrderProcessed
is the event name, following Pascal Case, and OrderProcessed
is also used in the event handler.
10. Boolean Variables (Prefixes like "is", "has", "can")
Boolean variables should clearly indicate that they hold true
or false
values by starting with verbs such as "is", "has", or "can".
Example:
csharp public class Order { public bool IsProcessed { get; set; } public bool HasShipped { get; set; } public bool CanCancelOrder { get; set; } }
In this example, IsProcessed
, HasShipped
, and CanCancelOrder
are Boolean properties that clearly convey their purpose.
11. Method Parameters (camelCase)
Method parameters should be written in camelCase and should use meaningful names that explain their purpose.
Example:
csharp public void ProcessOrder(string orderNumber, DateTime orderDate) { // Code here }
In this example, orderNumber
and orderDate
are method parameters following camelCase.
12. Static Fields (Pascal Case)
Static fields in C# generally follow Pascal Case (without underscores) because they are accessible at the class level and should stand out.
Example:
csharp public class DatabaseConfig { public static string ConnectionString { get; set; } }
In this example, ConnectionString
is a static field following Pascal Case.
13. Readonly Fields (camelCase with underscore)
Readonly fields that are not constants but are immutable after being initialized should follow camelCase with an underscore.
Example:
csharp public class Employee { private readonly string _employeeId; public Employee(string employeeId) { _employeeId = employeeId; } }
In this example, _employeeId
is a readonly field using camelCase with an underscore.
14. Attributes (Pascal Case with "Attribute" Suffix)
Custom attributes should follow Pascal Case and end with the suffix "Attribute". Even though you can omit the "Attribute" suffix when applying it, it's part of the convention when defining custom attributes.
Example:
csharp public class AuthorAttribute : Attribute { public string Name { get; set; } public AuthorAttribute(string name) { Name = name; } }
In this example, AuthorAttribute
follows Pascal Case with the "Attribute" suffix.
15. Interfaces for Async Methods (Pascal Case with "Async" Suffix)
When naming asynchronous methods, it's a common practice to append the suffix "Async" to make it clear that the method returns a Task
or Task<T>
and is asynchronous.
Example:
csharp public interface IOrderProcessor { Task ProcessOrderAsync(int orderId); }
In this example, ProcessOrderAsync
is an asynchronous method that follows Pascal Case with the "Async" suffix.
16. Collection and Array Naming (Plural Nouns)
When naming variables that store collections or arrays, use plural nouns to indicate that they hold multiple values.
Example:
csharp public class CustomerOrder { public List<string> Products { get; set; } public string[] CustomerAddresses { get; set; } }
In this example, Products
(List) and CustomerAddresses
(array) are named using plural nouns to reflect that they contain multiple items.
17. Delegate Names (Pascal Case with "Handler" Suffix)
Delegates should use Pascal Case and usually end with the suffix "Handler" to indicate their role.
Example:
csharp public delegate void OrderProcessedHandler(object sender, EventArgs e);
In this example, OrderProcessedHandler
is a delegate name using Pascal Case with the "Handler" suffix.
18. Extension Methods (Pascal Case)
Extension methods should be written in Pascal Case and placed in a static class with the appropriate naming to clarify their purpose.
Example:
csharp public static class StringExtensions { public static bool IsNullOrEmpty(this string value) { return string.IsNullOrEmpty(value); } }
In this example, IsNullOrEmpty
is an extension method following Pascal Case, and StringExtensions
is a static class indicating that it contains extensions for strings.
19. Properties with Backing Fields (camelCase with underscore for fields)
When using properties with backing fields, the property should follow Pascal Case, while the backing field should follow camelCase with an underscore.
Example:
csharp public class Employee { private string _name; public string Name { get { return _name; } set { _name = value; } } }
In this example, Name
(property) follows Pascal Case, and _name
(backing field) follows camelCase with an underscore.
20. Parameter Naming in Constructors and Methods (Descriptive camelCase)
When naming parameters in constructors and methods, use descriptive camelCase names that clearly indicate the parameter's role. This helps improve readability and understanding of the purpose of each parameter.
Example:
csharp public class Customer { private string _customerName; // Constructor with descriptive parameter name public Customer(string customerName) { _customerName = customerName; } // Method with descriptive parameters public void UpdateCustomerDetails(string newName, string newAddress) { _customerName = newName; // Update address logic here } }
In this example, customerName
, newName
, and newAddress
are method and constructor parameters that clearly describe the values being passed. This improves clarity when reading or debugging code.