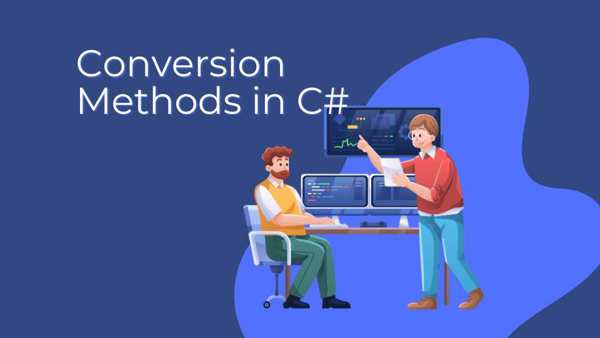
In C#, conversion methods are used to change the type of a variable from one data type to another. This is often necessary when you want to perform operations that require compatible types or when you need to store data in a different format. Let’s break this down in simple terms, with real-life examples, advanced code, and best practices.
1. Types of Conversion Methods in C#
a. Implicit Conversion
- What it is: Automatically done by the compiler when there is no risk of data loss.
- Example in Real Life: Pouring water from a small cup into a larger one. You don’t lose any water.
- Code Example:
int smallNumber = 100; long bigNumber = smallNumber; // Implicit conversion from int to long
- When to Use: When converting from a smaller data type (e.g.,
int
) to a larger one (e.g.,long
).
b. Explicit Conversion (Casting)
- What it is: Manually done by the developer when there’s a risk of data loss.
- Example in Real Life: Pouring water from a large bucket into a small cup. You might lose some water.
- Code Example:
double bigNumber = 123.45; int smallNumber = (int)bigNumber; // Explicit conversion from double to int
- When to Use: When converting from a larger data type (e.g.,
double
) to a smaller one (e.g.,int
).
c. Conversion Using Helper Methods
- What it is: Using built-in methods like
Convert.ToInt32()
,ToString()
,Parse()
, andTryParse()
. - Example in Real Life: Converting a paper document into a digital PDF using a scanner.
- Code Example:
string numberString = "123"; int number = Convert.ToInt32(numberString); // Convert string to int
- When to Use: When converting between incompatible types like
string
toint
.
d. Custom Conversion (User-Defined)
- What it is: Creating your own conversion logic using methods or operators.
- Example in Real Life: Converting a recipe from metric units to imperial units.
- Code Example:
public class Temperature { public double Celsius { get; set; } public static explicit operator Fahrenheit(Temperature temp) { return new Fahrenheit { Value = (temp.Celsius * 9 / 5) + 32 }; } } public class Fahrenheit { public double Value { get; set; } } Temperature temp = new Temperature { Celsius = 25 }; Fahrenheit fahr = (Fahrenheit)temp; // Custom conversion
- When to Use: When you need to define your own conversion logic.
2. Best Practices and Features
Best Practices
- Use
TryParse
for Safe Conversions: Avoid exceptions by usingTryParse
for string-to-number conversions.
string input = "123"; int result; bool success = int.TryParse(input, out result);
- Avoid Data Loss: Be cautious when using explicit casting to prevent losing data.
- Use Helper Methods: Prefer
Convert
orParse
methods for readability and simplicity. - Validate Input: Always validate input before performing conversions.
- Use Custom Conversions Sparingly: Only create custom conversions when absolutely necessary.
Features
- Type Safety: Ensures that conversions are valid and prevent runtime errors.
- Flexibility: Supports both built-in and user-defined conversions.
- Performance: Implicit conversions are faster as they are handled by the compiler.
3. Pros and Cons
Pros
- Flexibility: Allows you to work with different data types seamlessly.
- Readability: Helper methods like
Convert.ToInt32()
make code easier to understand. - Customization: You can define your own conversion logic.
Cons
- Data Loss: Explicit conversions can lead to data loss (e.g., converting
double
toint
). - Exceptions: Invalid conversions can throw exceptions (e.g.,
FormatException
). - Complexity: Custom conversions can make code harder to maintain.
4. Alternatives
- Generics: Use generics to avoid the need for type conversions in some cases.
- Polymorphism: Use inheritance and interfaces to handle different types without conversion.
- Serialization: Convert objects to JSON or XML for storage or transmission.
5. When to Use Conversion Methods
- When Working with APIs: APIs often return data as strings, which need to be converted to numbers or other types.
- Data Processing: When reading data from files or databases, conversions are often necessary.
- User Input: Convert user input (e.g., from a text box) to the required data type.
- Interoperability: When working with different systems or libraries that use different data types.
Advanced Code Example
using System; class Program { static void Main() { // Implicit Conversion int intValue = 100; long longValue = intValue; Console.WriteLine($"Implicit Conversion: {longValue}"); // Explicit Conversion double doubleValue = 123.45; int intValue2 = (int)doubleValue; Console.WriteLine($"Explicit Conversion: {intValue2}"); // Helper Method Conversion string stringValue = "456"; int intValue3 = Convert.ToInt32(stringValue); Console.WriteLine($"Helper Method Conversion: {intValue3}"); // TryParse for Safe Conversion string invalidString = "abc"; int result; bool success = int.TryParse(invalidString, out result); Console.WriteLine($"TryParse Conversion: Success = {success}, Result = {result}"); // Custom Conversion Temperature temp = new Temperature { Celsius = 25 }; Fahrenheit fahr = (Fahrenheit)temp; Console.WriteLine($"Custom Conversion: {fahr.Value}°F"); } } public class Temperature { public double Celsius { get; set; } public static explicit operator Fahrenheit(Temperature temp) { return new Fahrenheit { Value = (temp.Celsius * 9 / 5) + 32 }; } } public class Fahrenheit { public double Value { get; set; } }
This code demonstrates all the conversion methods discussed, along with best practices and advanced techniques.