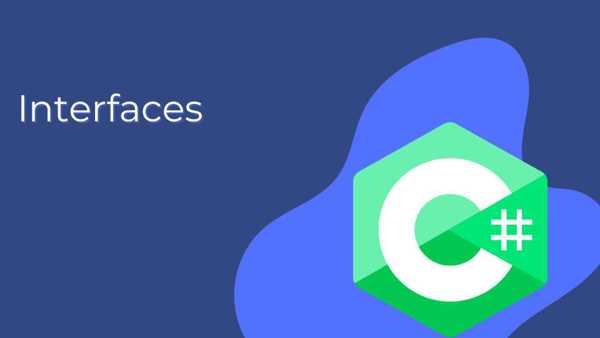
Scenario: A Media Player Application
Imagine you're building a media player application that supports multiple types of media, such as audio and video. Each media type has its own specific methods, but they also share some common functionality. We'll use explicit interface implementation to handle this scenario.
Step 1: Define the Interfaces
We’ll create two interfaces:
IPlayable
– For media that can be played.IStoppable
– For media that can be stopped.
Both interfaces have a method called Play()
and Stop()
, but their implementations will differ for audio and video.
public interface IPlayable { void Play(); } public interface IStoppable { void Stop(); }
Step 2: Create the Media Classes
We’ll create two classes:
AudioPlayer
– Implements bothIPlayable
andIStoppable
.VideoPlayer
– Implements bothIPlayable
andIStoppable
.
We’ll use explicit interface implementation to avoid naming conflicts and to provide specific behavior for each media type.
public class AudioPlayer : IPlayable, IStoppable { // Implicit implementation of IPlayable.Play public void Play() { Console.WriteLine("Audio is playing..."); } // Explicit implementation of IStoppable.Stop void IStoppable.Stop() { Console.WriteLine("Audio playback stopped."); } } public class VideoPlayer : IPlayable, IStoppable { // Implicit implementation of IPlayable.Play public void Play() { Console.WriteLine("Video is playing..."); } // Explicit implementation of IStoppable.Stop void IStoppable.Stop() { Console.WriteLine("Video playback stopped."); } }
Step 3: Use the Media Player
Now, let’s use the AudioPlayer
and VideoPlayer
classes in a program. We’ll demonstrate how explicit interface implementation works.
class Program { static void Main(string[] args) { // Create instances of AudioPlayer and VideoPlayer AudioPlayer audioPlayer = new AudioPlayer(); VideoPlayer videoPlayer = new VideoPlayer(); // Play audio and video audioPlayer.Play(); // Calls IPlayable.Play (implicit implementation) videoPlayer.Play(); // Calls IPlayable.Play (implicit implementation) // Stop audio and video (explicit implementation) ((IStoppable)audioPlayer).Stop(); // Calls IStoppable.Stop for audio ((IStoppable)videoPlayer).Stop(); // Calls IStoppable.Stop for video } }
Step 4: Output
When you run the program, you’ll see the following output:
Copy
Audio is playing... Video is playing... Audio playback stopped. Video playback stopped.
Step 5: Explanation
- Implicit Implementation:
- The
Play()
method is implemented implicitly in bothAudioPlayer
andVideoPlayer
. This means you can callPlay()
directly on the class instance.
- Explicit Implementation:
- The
Stop()
method is implemented explicitly. This means you can only callStop()
when the object is cast to theIStoppable
interface. - This is useful because it allows us to provide different behavior for
Stop()
inAudioPlayer
andVideoPlayer
without causing naming conflicts.
Step 6: Real-World Use Case
Imagine you’re building a plugin system for the media player. Some plugins might only support IPlayable
, while others might support both IPlayable
and IStoppable
. Explicit interface implementation allows you to:
- Hide the
Stop()
method from classes that don’t need it. - Provide specific behavior for
Stop()
in different media types.
Step 7: Complete Solution Code
Here’s the complete code for the solution:
using System; public interface IPlayable { void Play(); } public interface IStoppable { void Stop(); } public class AudioPlayer : IPlayable, IStoppable { // Implicit implementation of IPlayable.Play public void Play() { Console.WriteLine("Audio is playing..."); } // Explicit implementation of IStoppable.Stop void IStoppable.Stop() { Console.WriteLine("Audio playback stopped."); } } public class VideoPlayer : IPlayable, IStoppable { // Implicit implementation of IPlayable.Play public void Play() { Console.WriteLine("Video is playing..."); } // Explicit implementation of IStoppable.Stop void IStoppable.Stop() { Console.WriteLine("Video playback stopped."); } } class Program { static void Main(string[] args) { // Create instances of AudioPlayer and VideoPlayer AudioPlayer audioPlayer = new AudioPlayer(); VideoPlayer videoPlayer = new VideoPlayer(); // Play audio and video audioPlayer.Play(); // Calls IPlayable.Play (implicit implementation) videoPlayer.Play(); // Calls IPlayable.Play (implicit implementation) // Stop audio and video (explicit implementation) ((IStoppable)audioPlayer).Stop(); // Calls IStoppable.Stop for audio ((IStoppable)videoPlayer).Stop(); // Calls IStoppable.Stop for video } }
Step 8: Key Takeaways
- Explicit interface implementation is useful when:
- You have naming conflicts between interfaces.
- You want to hide certain methods from the class API.
- You need to provide different behavior for the same method in different contexts.
- Implicit interface implementation is simpler and more intuitive for most use cases.