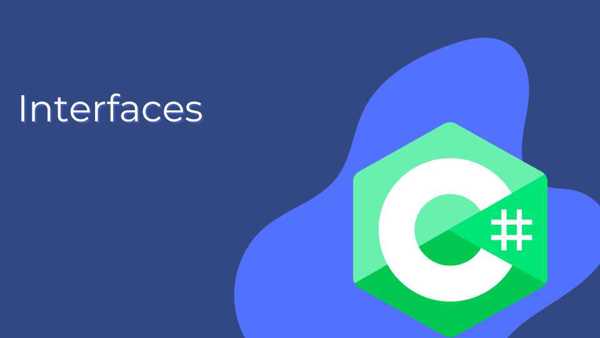
1. What is Interface Implementation?
In C#, an interface is like a contract that defines a set of methods, properties, or events that a class must implement. There are two ways to implement an interface: implicitly and explicitly.
2. Implicit Interface Implementation
- Layman's Explanation: This is the default way of implementing an interface. When you implement an interface implicitly, the methods are directly available on the class instance.
- Real-Life Example: Think of a car that implements a
IDrivable
interface. TheDrive()
method is directly accessible when you use the car object.
public interface IDrivable { void Drive(); } public class Car : IDrivable { public void Drive() { Console.WriteLine("Car is driving."); } } // Usage Car myCar = new Car(); myCar.Drive(); // Directly accessible
3. Explicit Interface Implementation
- Layman's Explanation: This is a more specific way of implementing an interface. The methods are only accessible when the object is treated as the interface type. It’s like having a hidden feature in your car that only works when you use a specific key (the interface).
- Real-Life Example: Imagine a car that also implements a
IFlyable
interface. TheFly()
method is only accessible when you treat the car as anIFlyable
object.
public interface IFlyable { void Fly(); } public class FlyingCar : IDrivable, IFlyable { public void Drive() { Console.WriteLine("FlyingCar is driving."); } void IFlyable.Fly() // Explicit implementation { Console.WriteLine("FlyingCar is flying."); } } // Usage FlyingCar myFlyingCar = new FlyingCar(); myFlyingCar.Drive(); // Directly accessible IFlyable flyingCar = myFlyingCar; // Treat as IFlyable flyingCar.Fly(); // Now Fly() is accessible
4. Best Practices and Features
- Best Practices:
- Use implicit implementation for commonly used methods.
- Use explicit implementation to avoid naming conflicts or to hide methods that are not part of the primary purpose of the class.
- Document explicitly implemented methods clearly since they are not directly visible on the class.
- Use explicit implementation to enforce stricter encapsulation.
- Avoid overusing explicit implementation as it can make the code harder to read.
- Features:
- Explicit implementation helps resolve naming conflicts when a class implements multiple interfaces with the same method name.
- Implicit implementation is simpler and more intuitive for most use cases.
5. Pros and Cons
- Implicit Implementation:
- Pros:
- Easier to use and understand.
- Methods are directly accessible on the class instance.
- Cons:
- Can lead to naming conflicts if multiple interfaces have the same method names.
- Explicit Implementation:
- Pros:
- Avoids naming conflicts.
- Provides better encapsulation by hiding interface methods from the class API.
- Cons:
- Slightly more complex to use.
- Methods are not directly accessible on the class instance.
6. Alternatives
- Abstract Classes: If you need to provide some default implementation, use an abstract class instead of an interface.
- Default Interface Methods (C# 8.0+): Allows interfaces to provide default implementations for methods, reducing the need for explicit implementation in some cases.
7. When to Use It
- Use implicit implementation when:
- The method is a core part of the class functionality.
- You want the method to be directly accessible on the class instance.
- Use explicit implementation when:
- You have naming conflicts between interfaces.
- You want to hide certain methods from the class API.
- The method is not part of the primary purpose of the class.
8. Advanced Example in a Project Context
Imagine you’re building a payment processing system. You have two interfaces: ICreditCardPayment
and IPayPalPayment
. Both have a ProcessPayment()
method, but the implementation differs.
public interface ICreditCardPayment { void ProcessPayment(); } public interface IPayPalPayment { void ProcessPayment(); } public class PaymentProcessor : ICreditCardPayment, IPayPalPayment { public void ProcessPayment() // Implicit implementation for ICreditCardPayment { Console.WriteLine("Processing credit card payment."); } void IPayPalPayment.ProcessPayment() // Explicit implementation for IPayPalPayment { Console.WriteLine("Processing PayPal payment."); } } // Usage PaymentProcessor processor = new PaymentProcessor(); processor.ProcessPayment(); // Calls ICreditCardPayment.ProcessPayment() IPayPalPayment paypalProcessor = processor; paypalProcessor.ProcessPayment(); // Calls IPayPalPayment.ProcessPayment()