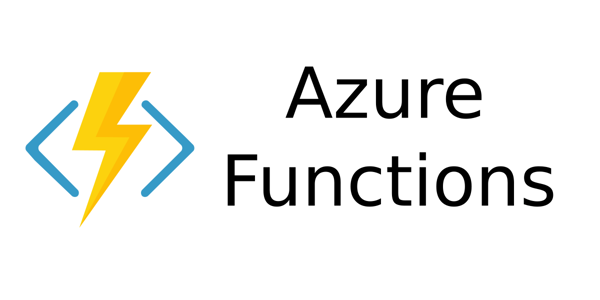
As cloud technologies evolve, the shift toward serverless computing has become more prevalent. Azure Functions, a serverless computing platform by Microsoft, enables developers to execute code in response to various events without managing infrastructure. Unlike traditional services, where developers must manage servers, networking, and storage, Azure Functions abstracts the infrastructure, letting developers focus solely on building applications.
In this article, we will explore Azure Functions, discuss their real-world applications, provide a step-by-step guide to getting started with Visual Studio 2022, and cover best practices to follow.
What is Azure Functions?
Azure Functions is a serverless computing service that allows you to execute small pieces of code (called "functions") in the cloud. These functions are event-driven and can be triggered by several events like HTTP requests, database changes, file uploads, or messages in a queue. You pay only for the time your function runs, making it highly cost-effective.
Real-Life Example: Automating Email Notifications
Let's take a real-world example to understand how Azure Functions can help businesses. Imagine you run an e-commerce website where you want to send an email notification to the user whenever they place an order. Instead of setting up dedicated servers to handle email dispatching, you can deploy a function that triggers when a new order is placed in the database. Azure Functions will handle this automatically without needing to manage servers.
This saves you time, money, and resources while ensuring scalability, as the function will automatically scale according to the number of incoming orders.
Setting Up Azure Functions in Visual Studio 2022
You can easily build Azure Functions using Visual Studio 2022. Follow these steps to create your first Azure Function:
Step 1: Install Azure Functions Tools for Visual Studio
- Open Visual Studio 2022.
- Go to Tools > Get Tools and Features.
- In the Workloads tab, check Azure Development.
- Ensure Azure Functions tools are selected in the summary pane, and install them.
Step 2: Create a New Azure Function Project
- Open Visual Studio 2022 and select Create a new project.
- Search for Azure Functions in the search bar and choose the Azure Functions template.
- Name your project and click Create.
Step 3: Select Trigger Type
You will be prompted to select a trigger type. For this example, let's choose an HTTP
trigger:
- Choose HTTP trigger from the list.
- Set the Authorization level to Anonymous for testing purposes (you can secure it later).
- Click Create.
Step 4: Write the Function Code
Visual Studio will generate a template function. Here’s a simple HTTP-triggered function that returns a greeting based on a query string parameter or request body:
csharp using System.IO; using Microsoft.AspNetCore.Mvc; using Microsoft.Azure.WebJobs; using Microsoft.Azure.WebJobs.Extensions.Http; using Microsoft.AspNetCore.Http; using Microsoft.Extensions.Logging; using Newtonsoft.Json; public static class HttpTriggeredFunction { [FunctionName("HttpTriggeredFunction")] public static async Task<IActionResult> Run( [HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req, ILogger log) { log.LogInformation("C# HTTP trigger function processed a request."); string name = req.Query["name"]; if (string.IsNullOrEmpty(name)) { return new BadRequestObjectResult("Please pass a name in the query string or in the request body."); } return new OkObjectResult($"Hello, {name}!"); } }
Step 5: Test the Function Locally
You can now run the function locally:
- Press F5 or click Start Debugging to run your Azure Function locally.
- Visual Studio will open a terminal showing the function URL (e.g.,
http://localhost:7071/api/HttpTriggeredFunction
). - Open a browser and navigate to the URL with a query parameter:
http://localhost:7071/api/HttpTriggeredFunction?name=Azure
The function will return Hello, Azure!
.
Step 6: Deploy to Azure
Once your function works locally, it’s time to deploy it to Azure:
- In Solution Explorer, right-click your project and select Publish.
- Choose Azure as the target, and follow the prompts to deploy your function to your Azure account.
Best Practices for Azure Functions
- Use Proper Triggers and Bindings
- Azure Functions support various triggers, such as HTTP, Queue, Timer, Blob, and Cosmos DB. Use the most appropriate trigger for your use case. Additionally, bindings allow you to connect to external resources like databases without writing much boilerplate code.
- Implement Dependency Injection
- Use dependency injection to manage services within your Azure Functions. This improves testability and maintainability by allowing you to separate business logic from the function code.
- Example:
csharp public class Startup : FunctionsStartup { public override void Configure(IFunctionsHostBuilder builder) { builder.Services.AddSingleton<IMyService, MyService>(); } }
- Optimize Cold Start
- Azure Functions may experience delays known as cold starts, particularly in consumption plans where functions are not always active. To mitigate this, consider using the Premium Plan or Always On settings in the App Service.
- Monitor and Log
- Enable Application Insights for detailed logging and performance monitoring. This will help you track failures, analyze usage patterns, and optimize your functions.
- Security
- Always secure your HTTP-triggered functions. Use API keys, OAuth, or Azure Active Directory for securing access. If your function interacts with sensitive data, implement encryption and follow proper authentication practices.
- Error Handling
- Ensure your functions handle errors gracefully. Implement retry policies for transient failures using Azure Functions Retry Policy. This will automatically retry failed executions under certain conditions.
- Avoid Long-Running Functions
- Azure Functions are not designed for long-running tasks. For operations that take more time, consider using Durable Functions. Durable Functions allow you to orchestrate workflows and manage state across multiple executions.
- Use Asynchronous Code
- Write your Azure Functions using asynchronous patterns. This allows functions to scale better by not blocking threads on I/O operations.
- Example:
csharp public static async Task<IActionResult> RunAsync(HttpRequest req) { string data = await SomeAsyncMethod(); return new OkObjectResult(data); }
- Scale Efficiently
- Azure Functions automatically scale based on demand. To ensure efficient scaling, avoid heavy computations within your function and offload resource-intensive tasks to other services like Azure Logic Apps or Azure Service Bus.
- Use App Settings for Configurations
- Store configuration values like connection strings, API keys, and URLs in the App Settings of your Azure Function App instead of hardcoding them in your function code.