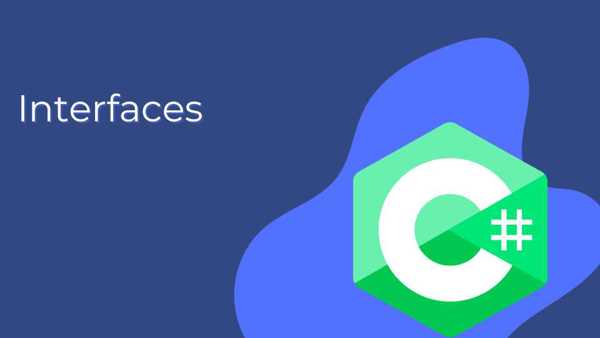
Interfaces are one of the most powerful features in C# and a cornerstone of Object-Oriented Programming (OOP). They define a contract that classes must follow, specifying what a class should do without dictating how it should do it. This allows for greater flexibility, modularity, and testability in your code.
1. What Are Interfaces?
Layman's Explanation
An interface is like a blueprint or a set of rules that a class must follow. It specifies what methods and properties a class must have, but it doesn’t provide the implementation. Think of it as a job description that outlines the responsibilities of a role, but not how to perform them.
Real-Life Example
Imagine a USB port. It defines a standard (interface) that any device (class) must follow to connect and communicate. Whether it’s a mouse, keyboard, or flash drive, as long as they adhere to the USB standard, they can work with any computer.
2. How Do Interfaces Work?
Basic Syntax
An interface defines a set of methods, properties, or events without implementation. Classes that implement the interface must provide the actual implementation.
interface IUSB { void Connect(); void TransferData(); } class Mouse : IUSB { public void Connect() { Console.WriteLine("Mouse connected."); } public void TransferData() { Console.WriteLine("Mouse data transferred."); } } class FlashDrive : IUSB { public void Connect() { Console.WriteLine("Flash drive connected."); } public void TransferData() { Console.WriteLine("Data copied to/from flash drive."); } }
Usage
IUSB device = new Mouse(); device.Connect(); // Mouse connected. device.TransferData(); // Mouse data transferred. device = new FlashDrive(); device.Connect(); // Flash drive connected. device.TransferData(); // Data copied to/from flash drive.
3. Real-Life Example in a Project
Let’s say you’re building a payment processing system. Different payment methods (credit card, PayPal, cryptocurrency) can be implemented as classes that follow a common interface.
Interface
interface IPaymentMethod { bool ProcessPayment(double amount); string GetPaymentConfirmation(); }
Implementations
class CreditCard : IPaymentMethod { public bool ProcessPayment(double amount) { // Simulate payment processing Console.WriteLine($"Processing credit card payment of ${amount}."); return true; // Assume payment is successful } public string GetPaymentConfirmation() { return "Credit card payment confirmed."; } } class PayPal : IPaymentMethod { public bool ProcessPayment(double amount) { // Simulate payment processing Console.WriteLine($"Processing PayPal payment of ${amount}."); return true; // Assume payment is successful } public string GetPaymentConfirmation() { return "PayPal payment confirmed."; } }
Usage in a Project
class PaymentProcessor { private IPaymentMethod _paymentMethod; public PaymentProcessor(IPaymentMethod paymentMethod) { _paymentMethod = paymentMethod; } public void MakePayment(double amount) { if (_paymentMethod.ProcessPayment(amount)) { Console.WriteLine(_paymentMethod.GetPaymentConfirmation()); } else { Console.WriteLine("Payment failed."); } } } // Client code IPaymentMethod paymentMethod = new CreditCard(); PaymentProcessor processor = new PaymentProcessor(paymentMethod); processor.MakePayment(100.00); // Processes credit card payment
4. Best Practices and Features
Best Practices
- Follow the Interface Segregation Principle: Create small, specific interfaces instead of large, general ones.
- Use Dependency Injection: Pass interfaces as dependencies to promote loose coupling.
- Name Interfaces Clearly: Use
I
prefix (e.g.,IPaymentMethod
) to indicate it’s an interface. - Avoid Method Implementation: Interfaces should only define contracts, not provide implementation (use abstract classes for shared logic).
- Document Interfaces: Clearly describe the purpose and usage of each interface.
Features
- Loose Coupling: Classes depend on interfaces, not concrete implementations.
- Extensibility: New classes can implement existing interfaces without modifying existing code.
- Testability: Interfaces make it easy to mock dependencies in unit tests.
- Multiple Inheritance: A class can implement multiple interfaces.
5. Pros and Cons
Pros
- Flexibility: Enables swapping implementations at runtime.
- Modularity: Promotes separation of concerns.
- Testability: Simplifies unit testing with mocking.
- Reusability: Interfaces can be reused across multiple projects.
Cons
- Complexity: Overuse of interfaces can make the code harder to understand.
- Boilerplate Code: Requires implementing all interface members in every class.
- No Shared Logic: Interfaces cannot provide default implementation (prior to C# 8.0).
6. Alternatives
Abstract Classes
- Use abstract classes when you need to provide shared logic or default implementations.
- Example:
abstract class PaymentMethod { public abstract bool ProcessPayment(double amount); public virtual string GetPaymentConfirmation() { return "Payment confirmed."; } }
Delegates
- Use delegates for single-method contracts.
- Example:
public delegate bool PaymentHandler(double amount); class PaymentProcessor { private PaymentHandler _handler; public PaymentProcessor(PaymentHandler handler) { _handler = handler; } public void MakePayment(double amount) { if (_handler(amount)) { Console.WriteLine("Payment successful."); } } }
7. When to Use Interfaces
- When You Need Multiple Implementations: Use interfaces when multiple classes need to follow the same contract but provide different implementations.
- When You Want Loose Coupling: Use interfaces to decouple classes and promote dependency injection.
- When You Need Extensibility: Use interfaces to allow future extensions without modifying existing code.
- When You Need Testability: Use interfaces to mock dependencies in unit tests.
Advanced Code Example
Let’s build a logging system where different loggers (file logger, database logger) implement a common interface.
Interface
interface ILogger { void Log(string message); void LogError(string error); }
Implementations
class FileLogger : ILogger { public void Log(string message) { // Write to a file System.IO.File.AppendAllText("log.txt", $"[INFO] {message}\n"); } public void LogError(string error) { // Write to a file System.IO.File.AppendAllText("log.txt", $"[ERROR] {error}\n"); } } class DatabaseLogger : ILogger { public void Log(string message) { // Simulate database logging Console.WriteLine($"Logging to database: {message}"); } public void LogError(string error) { // Simulate database logging Console.WriteLine($"Logging error to database: {error}"); } }
Usage in a Project
class Application { private ILogger _logger; public Application(ILogger logger) { _logger = logger; } public void Run() { _logger.Log("Application started."); try { // Simulate application logic throw new Exception("Test error"); } catch (Exception ex) { _logger.LogError(ex.Message); } } } // Client code ILogger logger = new FileLogger(); Application app = new Application(logger); app.Run();
Explanation of Advanced Code
- Interface:
ILogger
defines a contract for logging. - Implementations:
FileLogger
andDatabaseLogger
provide specific logging mechanisms. - Dependency Injection: The
Application
class depends onILogger
, making it flexible and testable.