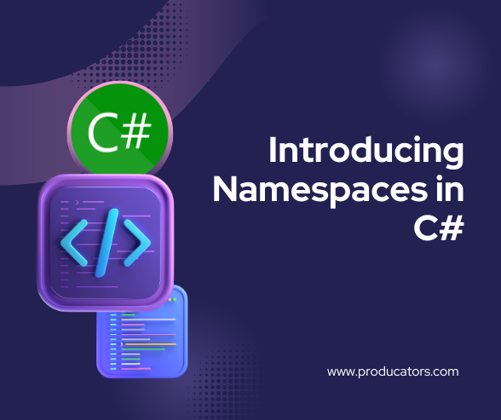
Introducing Namespaces in C#
Namespaces in C# are used to organize large code projects, avoid naming conflicts, and logically group related classes, interfaces, structs, enums, and delegates. They help developers manage code complexity by providing a structured way to partition code into logical sections. Namespaces also provide a layer of abstraction, making it easier to use and reference specific code elements across different files or assemblies.
How Namespaces Work
A namespace is essentially a container for classes and other types. When you create a class in C#, you can place it inside a namespace, and that class will be associated with it. This allows the class to be referenced elsewhere by specifying its full namespace path.
Why Use Namespaces?
- Avoid Naming Conflicts: Namespaces allow developers to create classes with the same name in different parts of the project without causing conflicts.
- Code Organization: Large projects can be broken into logical sections, making the project more maintainable.
- Easy Code Access: Namespaces enable easy referencing of code across different parts of an application using the
using
directive.
Declaring a Namespace
A namespace is declared using the namespace
keyword followed by the desired name.
Basic Example of Declaring and Using a Namespace
csharp namespace MyCompany.ProjectModule { public class Customer { public string Name { get; set; } } public class Order { public string OrderId { get; set; } } }
In this example:
MyCompany.ProjectModule
is the namespace that contains theCustomer
andOrder
classes.- The classes
Customer
andOrder
are encapsulated within this namespace, meaning that they can be referenced using their fully qualified names (MyCompany.ProjectModule.Customer
andMyCompany.ProjectModule.Order
).
Using a Namespace
When you want to access the types defined in a namespace from another part of your project, you use the using
directive, which allows you to import the namespace and access its classes directly.
Example of Using a Namespace
csharp using MyCompany.ProjectModule; public class MainApp { public static void Main(string[] args) { // Using Customer and Order from MyCompany.ProjectModule namespace Customer customer = new Customer(); customer.Name = "John Doe"; Order order = new Order(); order.OrderId = "12345"; } }
In this example, the using MyCompany.ProjectModule;
directive is used at the top of the file, allowing the Customer
and Order
classes to be referenced directly in the MainApp
class.
Fully Qualified Names
In some cases, you may choose to use the fully qualified name of a class (i.e., the class name with its namespace) rather than importing the namespace. This is useful when there are naming conflicts between classes from different namespaces.
Example of Fully Qualified Name
csharp public class MainApp { public static void Main(string[] args) { // Using fully qualified names without the 'using' directive MyCompany.ProjectModule.Customer customer = new MyCompany.ProjectModule.Customer(); customer.Name = "John Doe"; MyCompany.ProjectModule.Order order = new MyCompany.ProjectModule.Order(); order.OrderId = "12345"; } }
In this example, the Customer
and Order
classes are accessed using their fully qualified names without importing the MyCompany.ProjectModule
namespace at the top of the file.
Nested Namespaces
Namespaces can also be nested within one another to further organize code.
Example of Nested Namespace
csharp namespace MyCompany { namespace ProjectModule { public class Customer { public string Name { get; set; } } public class Order { public string OrderId { get; set; } } } }
In this case, the Customer
and Order
classes are in the MyCompany.ProjectModule
namespace, but you can also write it as:
csharp namespace MyCompany.ProjectModule { public class Customer { public string Name { get; set; } } public class Order { public string OrderId { get; set; } } }
System Namespace
The System namespace is one of the most commonly used namespaces in C#. It contains fundamental classes and base types, such as String
, Int32
, Console
, Math
, etc. Almost every C# application includes using System;
to access these classes.
Example of System Namespace Usage
csharp using System; public class MainApp { public static void Main(string[] args) { // Using the Console class from the System namespace Console.WriteLine("Hello, World!"); } }
In this example, the Console
class from the System
namespace is used to print text to the console.
Aliasing a Namespace
Sometimes, a namespace can be long or there may be conflicts between types in different namespaces. In such cases, you can alias a namespace.
Example of Namespace Alias
csharp using MyProject = MyCompany.ProjectModule; public class MainApp { public static void Main(string[] args) { // Using the alias MyProject to reference the namespace MyProject.Customer customer = new MyProject.Customer(); customer.Name = "Jane Doe"; } }
Here, MyProject
is an alias for the MyCompany.ProjectModule
namespace, making the code shorter and easier to read.
Benefits of Using Namespaces
- Modularity: Namespaces help break code into logical, manageable parts.
- Clarity: By grouping related classes, you improve code readability and maintenance.
- Scalability: As projects grow, namespaces help to organize large codebases, reducing complexity.
- Conflict Avoidance: Namespaces prevent naming collisions when using the same class names in different parts of a project or in referenced libraries.