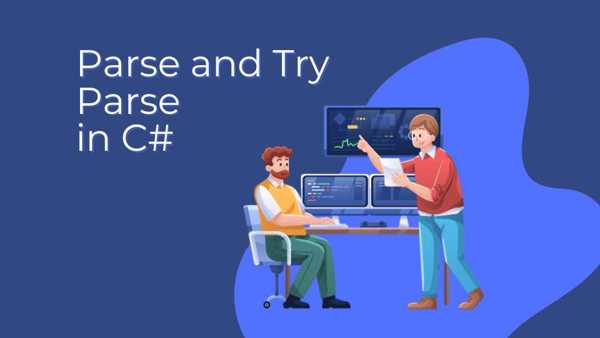
Parsing in C# is the process of converting a string (a sequence of characters) into another data type, such as an integer, float, or date. Think of it like translating a word from one language to another. For example, if you have the string "123"
, parsing it into an integer would give you the number 123
.
Real-Life Example:
Imagine you’re reading a recipe, and the instructions say, "Bake at 350 degrees." The temperature "350"
is written as text (a string), but your oven needs it as a number. Parsing is like converting that text into a number so your oven can understand it.
Advanced Code Example
Here’s how you can use parsing in C#:
using System; class Program { static void Main(string[] args) { // Example 1: Parsing a string to an integer string numberString = "42"; int number = int.Parse(numberString); Console.WriteLine($"Parsed integer: {number}"); // Output: Parsed integer: 42 // Example 2: Parsing a string to a double string doubleString = "3.14"; double pi = double.Parse(doubleString); Console.WriteLine($"Parsed double: {pi}"); // Output: Parsed double: 3.14 // Example 3: Parsing a string to a DateTime string dateString = "2023-10-15"; DateTime date = DateTime.Parse(dateString); Console.WriteLine($"Parsed date: {date.ToShortDateString()}"); // Output: Parsed date: 10/15/2023 } }
How it works:
int.Parse
,double.Parse
, andDateTime.Parse
are methods that convert strings into their respective data types.- If the string is not in the correct format (e.g.,
"abc"
forint.Parse
), the program will throw an exception (error).
Best Practices and Features of Parsing
- Use TryParse for Safety: Instead of
Parse
, useTryParse
to avoid exceptions when the string format is invalid.
string input = "abc"; int result; bool success = int.TryParse(input, out result); if (success) { Console.WriteLine($"Parsed integer: {result}"); } else { Console.WriteLine("Invalid input!"); }
- Handle Culture-Specific Formats: Use culture-specific parsing for dates and numbers (e.g.,
DateTime.Parse
withCultureInfo
). - Validate Input: Always validate or sanitize input before parsing to avoid errors.
- Use Appropriate Data Types: Choose the correct parsing method for the data type you need (e.g.,
int.Parse
,double.Parse
,DateTime.Parse
).
Pros and Cons of Parsing
Pros:
- Simple and Direct: Parsing is straightforward for converting strings to other data types.
- Built-In Methods: C# provides built-in methods like
int.Parse
,double.Parse
, andDateTime.Parse
. - Flexible: Works with various data types, including numbers, dates, and custom types.
Cons:
- Exceptions on Failure:
Parse
throws an exception if the input string is invalid, which can crash your program. - Limited Error Handling: Without
TryParse
, handling invalid input requires additional code. - Culture Sensitivity: Parsing can fail if the string format doesn’t match the expected culture-specific format.
Alternatives to Parsing
- TryParse: Use
TryParse
to safely handle invalid input without throwing exceptions. - csharp
- Copy
string input = "123"; int result; if (int.TryParse(input, out result)) { Console.WriteLine($"Parsed integer: {result}"); }
- Convert Class: Use the
Convert
class for more flexible conversions.
string input = "123"; int result = Convert.ToInt32(input);
- Custom Parsing Logic: Write your own parsing logic for complex or custom data types.
When to Use Parsing
Use parsing when:
- You need to convert a string into a numeric, date, or other data type.
- The input string is guaranteed to be in the correct format.
- You want a quick and simple way to perform the conversion.
Avoid parsing when:
- The input format is unpredictable or unreliable (use
TryParse
instead). - You need to handle culture-specific formats without proper configuration.
- The conversion logic is complex and requires custom handling.
Summary
Parsing in C# is a powerful tool for converting strings into other data types like integers, doubles, or dates. It’s simple and direct but can throw exceptions if the input is invalid. Use TryParse
for safer error handling, and always validate input before parsing. Parsing is ideal for predictable input formats, but for more complex scenarios, consider alternatives like Convert
or custom parsing logic.