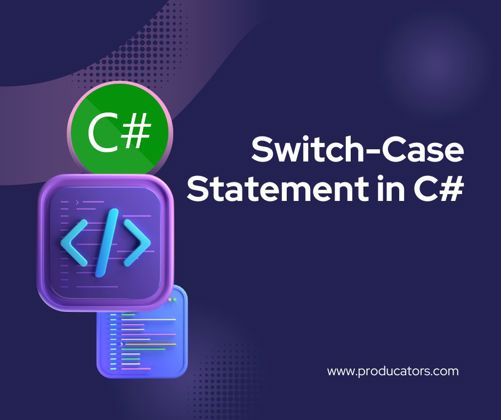
In C#, the switch-case
statement is a control flow structure that allows you to execute different blocks of code based on the value of a variable. It provides an alternative to using multiple if-else
statements and can make your code more readable and efficient when dealing with multiple conditions.
Syntax of switch-case
Here’s the basic syntax of a switch-case
statement in C#:
csharp switch (expression) { case value1: // Code to execute if expression equals value1 break; case value2: // Code to execute if expression equals value2 break; default: // Code to execute if none of the cases match break; }
- Expression: The value being checked (e.g., an integer, string, or enum).
- Case values: Values that are compared with the expression.
- Break: Prevents fall-through to the next case.
- Default: An optional block that executes if no case matches.
Example 1: Simple switch-case
Statement
csharp int day = 3; switch (day) { case 1: Console.WriteLine("Monday"); break; case 2: Console.WriteLine("Tuesday"); break; case 3: Console.WriteLine("Wednesday"); break; default: Console.WriteLine("Invalid day"); break; }
Breakdown:
- The
switch-case
checks the value ofday
. - Since
day
equals3
, "Wednesday" is printed. - The
default
case is executed if the value ofday
doesn't match any case.
Real-Life Example
Consider a vending machine that sells different drinks:
- If the customer selects 1, they get a soda.
- If they select 2, they get water.
- If they select 3, they get juice.
Here’s how this logic looks in C#:
csharp int selection = 2; switch (selection) { case 1: Console.WriteLine("Dispensing Soda"); break; case 2: Console.WriteLine("Dispensing Water"); break; case 3: Console.WriteLine("Dispensing Juice"); break; default: Console.WriteLine("Invalid selection"); break; }
In this case, if the user selects 2, "Dispensing Water" is printed.
Best Practices for switch-case
Statements
- Use
switch-case
for Multiple Conditions: If you’re checking the same variable against multiple values,switch-case
is more efficient and readable thanif-else
. - Example:
csharp switch (value) { case 1: case 2: case 3: // Handle these cases similarly break; }
- Always Include a
default
Case: Thedefault
block acts as a safety net if none of the cases match. It's important to handle unexpected inputs. - Avoid Fall-Through: In C#, you must explicitly break out of each case to avoid unintended fall-through to the next case. This is different from languages like C, where fall-through is allowed.
- Keep
switch
Statements Simple: Avoid overly complexswitch
statements with too many cases. If you find yourself with more than 5–7 cases, consider using other structures like enums or polymorphism. - Use
switch-case
with Enums for Better Code Readability: When possible, use enums withswitch-case
to make your code more meaningful. - Example:
csharp enum Color { Red, Green, Blue } switch (color) { case Color.Red: // Code for red break; case Color.Green: // Code for green break; }
Features of switch-case
Statements
- Efficient Execution: For large sets of conditions,
switch-case
can be faster thanif-else
, especially when there are many comparisons. - Cleaner Syntax:
switch-case
provides a clear, structured way to handle multiple conditions, improving code readability. - Supports Multiple Data Types: The
switch-case
statement works with various data types, including integers, strings, and enums. - Use of
goto case
andgoto default
: Allows jumping between cases, though it's generally considered poor practice as it can lead to complex, hard-to-read code. - Pattern Matching Support (C# 7.0+): Modern versions of C# allow pattern matching within
switch-case
, adding more flexibility and power to the structure.
switch-case
vs if-else
Both switch-case
and if-else
statements are used to make decisions in code, but switch-case
is often preferred when:
- You are checking the value of a single variable against many possible options.
- The code becomes too cluttered with nested
if-else
statements. - You want a cleaner, more readable alternative.
For example:
csharp int score = 90; if (score >= 90) { Console.WriteLine("Grade A"); } else if (score >= 80) { Console.WriteLine("Grade B"); } else if (score >= 70) { Console.WriteLine("Grade C"); } else { Console.WriteLine("Grade D"); }
This logic can be simplified with switch-case
:
csharp int score = 90; switch (score / 10) { case 9: case 10: Console.WriteLine("Grade A"); break; case 8: Console.WriteLine("Grade B"); break; case 7: Console.WriteLine("Grade C"); break; default: Console.WriteLine("Grade D"); break; }
Real-Life Scenario for switch-case
Let’s say you are working on a pizza ordering system where customers can choose different sizes of pizza, and the price is determined based on the selected size:
csharp string pizzaSize = "Large"; switch (pizzaSize) { case "Small": Console.WriteLine("The price is $5."); break; case "Medium": Console.WriteLine("The price is $7."); break; case "Large": Console.WriteLine("The price is $10."); break; default: Console.WriteLine("Invalid size selected."); break; }
Here, the program will print "The price is $10." since the selected size is "Large."
Build a Simple Application Using switch-case
Let’s build a simple calculator application that performs basic arithmetic operations like addition, subtraction, multiplication, and division based on user input.
csharp using System; public class SimpleCalculator { public static void Main(string[] args) { Console.WriteLine("Enter first number:"); double num1 = Convert.ToDouble(Console.ReadLine()); Console.WriteLine("Enter operator (+, -, *, /):"); char operation = Console.ReadLine()[0]; Console.WriteLine("Enter second number:"); double num2 = Convert.ToDouble(Console.ReadLine()); switch (operation) { case '+': Console.WriteLine($"Result: {num1 + num2}"); break; case '-': Console.WriteLine($"Result: {num1 - num2}"); break; case '*': Console.WriteLine($"Result: {num1 * num2}"); break; case '/': if (num2 != 0) { Console.WriteLine($"Result: {num1 / num2}"); } else { Console.WriteLine("Cannot divide by zero."); } break; default: Console.WriteLine("Invalid operator."); break; } } }
Explanation:
- The user is prompted to enter two numbers and an arithmetic operator.
- Based on the operator (
+
,-
,*
,/
), the corresponding calculation is performed. - The
default
case handles invalid operators.