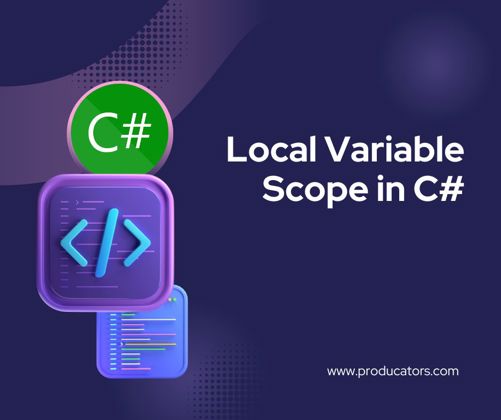
In programming, variables are used to store data, and the scope of a variable defines where that variable can be accessed or used in your code. Understanding the scope of local variables is essential to ensure proper memory management and to avoid errors like accidental modification of variables or access to undefined values.
In C#, local variables have a specific scope, meaning they are only accessible within the block of code where they are declared. This ensures that variables are only used where appropriate and helps to avoid conflicts with other parts of the program.
What is a Local Variable?
A local variable is a variable declared inside a method, constructor, or block of code. These variables are created when the block of code is entered, and destroyed when the block is exited. Local variables are not accessible outside the method or block where they are declared.
Syntax Example
csharp void DisplayMessage() { string message = "Hello, World!"; Console.WriteLine(message); }
In this example, message
is a local variable. It is only accessible within the DisplayMessage
method. Once the method completes execution, the message
variable is destroyed and can no longer be used.
Scope of Local Variables
The scope of a local variable begins from the point where it is declared and ends when the block of code that contains the variable terminates. This ensures that:
- The variable can only be accessed within the method or block where it is declared.
- It avoids conflicts with variables of the same name in different blocks.
Example: Scope of Local Variables
csharp void CalculateSum() { int x = 10; int y = 20; int sum = x + y; Console.WriteLine("Sum: " + sum); }
In the example above, x
, y
, and sum
are local variables. They are only accessible inside the CalculateSum
method. If you try to access any of these variables outside this method, it will result in an error.
Real-Life Example of Local Variable Scope
Imagine you’re writing code for a vending machine. Inside the method that handles selecting an item, you use a local variable to store the selected item. Once the user selects an item and receives their product, that local variable is no longer needed.
csharp void SelectItem() { string selectedItem = "Soda"; Console.WriteLine("You have selected: " + selectedItem); }
Here, selectedItem
is a local variable. After the SelectItem
method completes, selectedItem
is no longer accessible, which is exactly what you want since the item selection is now complete.
Features of Local Variables
- Memory Efficiency: Local variables are destroyed as soon as the block of code they belong to finishes execution. This ensures efficient use of memory.
- Encapsulation: Local variables are not accessible outside their block, keeping your code modular and preventing unintended modifications from other parts of the program.
- No Default Value: Local variables must be initialized before use because they don’t have a default value. Unlike class-level fields that default to
null
or0
, local variables must be assigned a value explicitly.
Best Practices for Using Local Variables
- Limit Variable Scope: Declare variables as close to their usage as possible. This reduces the likelihood of errors and keeps the scope minimal.
- Example:
csharp for (int i = 0; i < 10; i++) { int temp = i * 2; // Declare 'temp' inside the loop Console.WriteLine(temp); }
- Avoid Redefining Variables in Nested Scopes: Avoid reusing the same variable name in nested blocks to prevent confusion and potential errors.
- Example:
csharp int value = 10; if (true) { int value = 20; // Avoid this practice as it leads to ambiguity Console.WriteLine(value); }
- Initialize Variables Before Use: Always initialize local variables before using them. Accessing an uninitialized local variable will result in a compilation error.
- Example:
csharp Copy code int result; // result is uninitialized here result = 100; // Always initialize before using
- Use Descriptive Names: Local variable names should clearly describe the value they hold. This makes your code easier to read and maintain.
- Example:
csharp Copy code string customerName = "John Doe"; int totalItemsPurchased = 5;
- Declare Variables Only When Needed: Do not declare all your variables at the top of the method. Declare them only when needed to keep the code clean and more maintainable.
Building a Simple Application Using Local Variables
Let’s build a simple calculator using local variables to perform arithmetic operations. The calculator will take two numbers from the user, perform a selected operation, and return the result.
csharp using System; public class SimpleCalculator { public static void Main(string[] args) { Console.WriteLine("Enter the first number:"); double num1 = Convert.ToDouble(Console.ReadLine()); Console.WriteLine("Enter the second number:"); double num2 = Convert.ToDouble(Console.ReadLine()); Console.WriteLine("Enter an operator (+, -, *, /):"); char operation = Console.ReadLine()[0]; double result = 0; switch (operation) { case '+': result = num1 + num2; break; case '-': result = num1 - num2; break; case '*': result = num1 * num2; break; case '/': if (num2 != 0) { result = num1 / num2; } else { Console.WriteLine("Cannot divide by zero."); } break; default: Console.WriteLine("Invalid operator."); break; } if (operation == '+' || operation == '-' || operation == '*' || (operation == '/' && num2 != 0)) { Console.WriteLine("The result is: " + result); } } }
Explanation:
- Local variables
num1
,num2
,operation
, andresult
are declared within theMain
method. - Their scope is limited to the
Main
method, meaning they cannot be accessed outside of it.
Scope and Life Cycle of Local Variables
- Creation: A local variable is created when the execution of its method or block starts.
- Access: The variable can be accessed only within the method or block where it is declared.
- Destruction: Once the method or block exits, the variable is destroyed, and its memory is freed.
This behavior ensures efficient use of memory and prevents issues like accessing invalid or outdated data.
Local Variables vs Global Variables
- Local variables are restricted to the block or method in which they are declared, providing better modularity and control.
- Global variables (also known as fields) are declared at the class level and can be accessed by any method in the class. While this makes them more flexible, it also increases the risk of accidental modification or misuse.