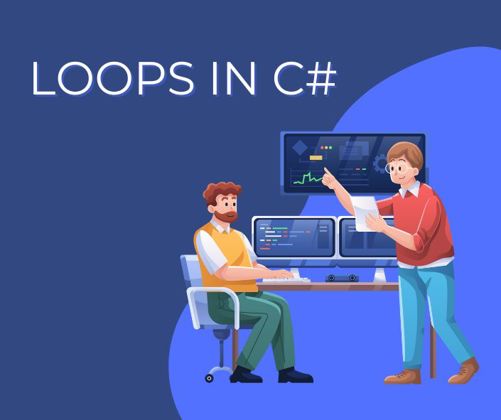
Loops are fundamental in programming, allowing repetitive actions until a condition is met. In C#, loops streamline tasks that would otherwise require manual repetition. This article explains the main loop types in C#, along with examples, best practices, and a simple application to demonstrate their use.
Types of Loops in C#
C# offers several types of loops, each suited to different scenarios. The main types are:
for
loopwhile
loopdo...while
loopforeach
loop
1. for
Loop
A for
loop is ideal for situations where the number of iterations is known. It consists of three parts: initialization, condition, and increment.
Syntax:
csharp for (initialization; condition; increment) { // Code to execute in each iteration }
Example: Display numbers from 1 to 5.
csharp for (int i = 1; i <= 5; i++) { Console.WriteLine(i); }
Explanation: The loop initializes i
to 1, checks if i
is less than or equal to 5, then increments i
in each iteration. The output will be:
1 2 3 4 5
Real-life Scenario
Imagine a student grading system that needs to calculate the total marks for five subjects. A for
loop makes it efficient to sum the marks.
2. while
Loop
A while
loop runs as long as its condition is true
. It’s useful when the number of iterations isn’t known initially.
Syntax:
csharp while (condition) { // Code to execute }
Example: Calculate the factorial of a number.
csharp int number = 5; int factorial = 1; while (number > 1) { factorial *= number; number--; } Console.WriteLine(factorial);
Explanation: This code calculates 5!
(factorial of 5) by multiplying factorial
by number
and decreasing number
until it reaches 1.
3. do...while
Loop
The do...while
loop ensures the code runs at least once before checking the condition. This loop is handy for scenarios requiring one guaranteed execution.
Syntax:
csharp do { // Code to execute } while (condition);
Example: Prompt the user to enter a positive number.
csharp int input; do { Console.WriteLine("Enter a positive number:"); input = int.Parse(Console.ReadLine()); } while (input <= 0);
Explanation: The program asks the user for a positive number. The loop continues until the user enters a positive value, ensuring that the prompt appears at least once.
4. foreach
Loop
The foreach
loop iterates over each element in a collection, making it suitable for arrays, lists, and other collections.
Syntax:
csharp foreach (var item in collection) { // Code to execute }
Example: Display a list of names.
csharp string[] names = { "Alice", "Bob", "Charlie" }; foreach (string name in names) { Console.WriteLine(name); }
Explanation: The loop iterates over each name in the array and displays it. This loop is ideal for traversing collections without tracking indices.
Simple Application: Calculating Average Score
Let’s build a simple C# application that calculates the average score of students.
csharp int[] scores = { 85, 90, 78, 92, 88 }; int total = 0; foreach (int score in scores) { total += score; } double average = (double)total / scores.Length; Console.WriteLine($"The average score is {average}");
Explanation: This program uses a foreach
loop to add all scores and then calculates the average by dividing the total by the number of scores. It’s an efficient way to find the average score in a list.
Best Practices for Using Loops in C#
- Avoid Infinite Loops: Ensure that loops have an exit condition. Infinite loops can crash applications or consume excessive resources.
- Use the Right Loop Type: Choose the loop type that best matches your scenario. For instance, use
for
loops for counted iterations andwhile
loops for conditions. - Keep Conditions Simple: Complex conditions in loops can slow down performance. Simplify the logic whenever possible.
- Avoid Nested Loops Where Possible: Nested loops increase time complexity. Use single loops or consider alternative structures for improved performance.
- Minimize Side Effects: Loops that modify external variables can lead to unintended consequences. Keep side effects within the loop limited or documented.
- Utilize
break
andcontinue
Wisely: Thebreak
statement exits the loop, whilecontinue
skips to the next iteration. Use them for efficiency but avoid overuse as they can complicate readability.
Features of Loops in C#
- Repetitive Tasks: Loops simplify repetitive tasks, such as iterating over data, processing collections, or executing code a specific number of times.
- Flexible Structures: Different loop types give developers flexibility based on the required conditions.
- Dynamic Conditions: Loops can operate based on runtime conditions, adapting as data changes.
- Code Efficiency: Loops reduce code redundancy by performing repetitive actions with minimal code.
Building a Number Guessing Game
Here’s a simple number-guessing game using loops, showcasing how loops can create an interactive program.
csharp Random random = new Random(); int target = random.Next(1, 101); int guess; int attempts = 0; Console.WriteLine("Guess the number (between 1 and 100):"); while (true) { guess = int.Parse(Console.ReadLine()); attempts++; if (guess < target) { Console.WriteLine("Too low, try again."); } else if (guess > target) { Console.WriteLine("Too high, try again."); } else { Console.WriteLine($"Correct! You guessed the number in {attempts} attempts."); break; } }
Explanation: The program generates a random target number between 1 and 100. The player enters guesses until they find the target number. The while
loop continues until the correct guess, after which the break
statement exits the loop.