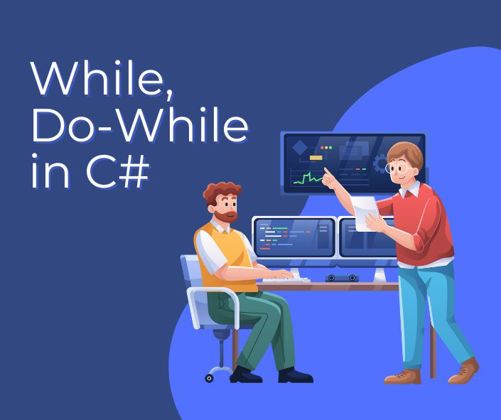
Loops are crucial for programming, enabling us to perform repeated actions based on specific conditions. Two common loop structures in C# are the while
loop and the do-while
loop. While they have similar purposes, they are structured differently, and each is suited to distinct scenarios. This article dives into the fundamentals of each, offers real-life examples, best practices, and provides a sample application to demonstrate how they work in action.
Overview of while
and do-while
Loops
The while
Loop
The while
loop runs a block of code as long as a specified condition is true
. It is best suited when you may or may not need the loop to execute, depending on whether the condition is met at the start.
Syntax:
csharp while (condition) { // Code to execute as long as the condition is true }
Example: Checking bank balance Imagine checking your bank balance and notifying yourself when it’s below a threshold.
csharp decimal balance = 1000m; decimal withdrawal = 200m; while (balance > 500) { Console.WriteLine($"Your balance is {balance}, which is safe."); balance -= withdrawal; }
In this example, as long as balance
is above 500, the loop continues to execute, updating the balance each time.
The do-while
Loop
The do-while
loop is similar to the while
loop but guarantees that the code block runs at least once, regardless of the condition. The condition is checked after the block of code executes.
Syntax:
csharp do { // Code to execute } while (condition);
Example: User input validation Imagine you’re prompting a user to enter a positive number. Using a do-while
loop ensures the prompt appears at least once.
csharp int number; do { Console.WriteLine("Enter a positive number:"); number = int.Parse(Console.ReadLine()); } while (number <= 0);
Here, the user is prompted to enter a number, and if it’s not positive, the prompt reappears. This continues until a positive number is entered.
Real-Life Example of while
and do-while
- While Loop Example: Monitoring temperature in a system Imagine you have a temperature sensor, and you want the system to continue checking the temperature as long as it stays within a safe range.
csharp int temperature = 70; // current temperature while (temperature < 100) { Console.WriteLine($"Temperature is safe at {temperature} degrees."); temperature += 5; // simulate temperature increase }
- This code could represent a system that monitors a room's temperature, allowing it to run safely until the temperature reaches a critical level.
- Do-While Loop Example: Asking for a password Think about a system that prompts for a password. You want the prompt to appear at least once, regardless of the entered password.
csharp string password; string correctPassword = "secure123"; do { Console.WriteLine("Enter your password:"); password = Console.ReadLine(); } while (password != correctPassword); Console.WriteLine("Access granted.");
- This code ensures the user is prompted to enter a password at least once and repeats until they enter the correct password.
Best Practices for while
and do-while
Loops
- Avoid Infinite Loops: Always ensure the loop’s condition will eventually be false to avoid creating infinite loops, which can crash your program.
- Use
while
for Pre-Condition Scenarios: Choose awhile
loop when you need to check the condition before executing the block of code. This is ideal for tasks that might not need to run at all if the condition isn’t met. - Use
do-while
for Post-Condition Scenarios: Use ado-while
loop if you need the loop to execute at least once. This is particularly useful for tasks that must happen at least once before checking conditions, like prompting for user input. - Limit Scope of Variables: Define variables within the smallest scope possible to reduce memory usage and increase code readability.
- Minimize Loop Complexity: Avoid deeply nested loops or conditions within loops. Complex loops can slow down performance and make debugging harder.
- Clear Comments and Naming: Write clear comments to describe the purpose of your loops, and use descriptive variable names to make your code easier to read and maintain.
Simple Application: Sum of Positive Numbers
Let’s build a small C# application that uses a do-while
loop to allow a user to enter multiple positive numbers, adding them up until they decide to stop by entering a negative number.
csharp int number; int sum = 0; do { Console.WriteLine("Enter a positive number (or a negative number to end):"); number = int.Parse(Console.ReadLine()); if (number > 0) { sum += number; } } while (number > 0); Console.WriteLine($"The sum of all positive numbers entered is: {sum}");
Explanation:
- This program keeps prompting the user for positive numbers and adds them to
sum
. - If the user enters a negative number, the loop stops.
- The final sum of all positive numbers is displayed.
This example demonstrates how do-while
ensures the prompt runs at least once, even if the first entry is negative.
Features of while
and do-while
Loops in C#
- Flexible Execution: Both loops allow dynamic execution based on conditions. The
while
loop checks conditions beforehand, whiledo-while
executes at least once before evaluating the condition. - Reduced Code Redundancy: Using loops allows repeating actions without rewriting code, saving space and improving efficiency.
- User Control: Loops can be controlled based on user input, as seen in input validation examples.
- Error Handling: Loops can incorporate error-handling routines, ensuring a program can run correctly even with invalid inputs.
Summary and Conclusion
Understanding while
and do-while
loops is essential in C#. Each loop type is beneficial for specific scenarios, allowing repetitive tasks based on conditions. The while
loop checks conditions before running, ideal for situations that may not need execution. The do-while
loop runs at least once before condition checks, perfect for initial user prompts or actions.
Key Takeaways
- Use
while
for pre-condition checks anddo-while
for post-condition requirements. - Carefully manage loop conditions to avoid infinite loops.
- Implement best practices to maintain code efficiency and readability.